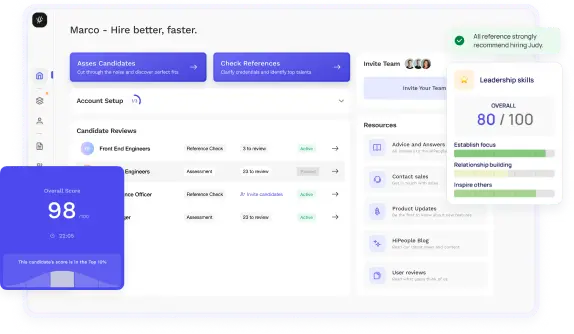
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to demonstrate your mastery of Python programming in the most challenging interviews? Delve into the world of Advanced Python Interview Questions, where we'll equip you with the knowledge and strategies to tackle complex coding challenges, showcase your problem-solving skills, and stand out in interviews for high-level Python roles. Whether you're aiming for a senior Python developer, data scientist, or machine learning engineer position, this guide will be your trusted companion on your journey to interview success.
An advanced Python interview is a job interview specifically designed to evaluate a candidate's proficiency in Python programming beyond the basics. These interviews are typically conducted for roles that require in-depth Python knowledge, such as senior Python developer, data scientist, machine learning engineer, or software architect positions. In such interviews, you can expect to encounter more challenging and complex questions and scenarios compared to entry-level interviews.
Python interviews are pivotal steps in the recruitment process for Python-related roles. Understanding their importance is crucial for candidates and employers alike.
Understanding the significance of Python interviews empowers candidates to prepare effectively and employers to make informed hiring decisions, ultimately contributing to successful and fulfilling career paths.
Preparing for Python interviews is essential to showcase your skills and land your dream job.
Preparing for Python-specific questions is crucial. Here are some common topics and questions you might encounter:
Behavioral questions assess your soft skills, teamwork, and problem-solving abilities. Prepare for questions like:
Navigating a technical interview requires a specific approach.
Practice makes perfect. To prepare effectively:
To wrap up your interview preparation, here are a few tips:
By following these strategies and practicing consistently, you'll be well-prepared to excel in your Python interviews. Remember that preparation and confidence go hand in hand, and with dedication, you can achieve your career goals in the Python programming world.
How to Answer: Describe how inheritance allows a class to inherit properties and methods from another class. Explain the super() function and demonstrate how to create subclasses and access parent class methods and attributes.
Sample Answer: Inheritance in Python is a mechanism where a class (subclass or derived class) inherits attributes and methods from another class (base class or parent class). It promotes code reusability and hierarchical organization. To use inheritance, you can create a subclass that inherits from a parent class using the following syntax:
class Parent:
def __init__(self, name):
self.name = name
class Child(Parent):
def __init__(self, name, age):
super().__init__(name) # Call the parent class constructor
self.age = age
In this example, the Child class inherits the name attribute from the Parent class and adds its own age attribute.
What to Look For: Look for candidates who can explain the concept clearly, demonstrate practical usage, and correctly use super() to access the parent class constructor.
How to Answer: Explain method overriding as a concept where a subclass provides a specific implementation for a method that is already defined in its parent class. Highlight the importance of maintaining the method signature.
Sample Answer: Method overriding in Python occurs when a subclass provides its own implementation of a method that is already defined in its parent class. This allows the subclass to customize the behavior of that method without changing its name or parameters. To override a method, you need to define a method in the subclass with the same name and parameters as the method in the parent class. Here's an example:
class Parent:
def greet(self):
print("Hello from Parent")
class Child(Parent):
def greet(self):
print("Hello from Child")
child = Child()
child.greet() # This will call the greet method in the Child class.
In this example, the Child class overrides the greet method inherited from the Parent class.
What to Look For: Assess whether candidates understand the concept of method overriding, can provide clear examples, and emphasize the importance of method signature consistency.
How to Answer: Describe decorators as functions that modify the behavior of other functions or methods. Explain their syntax and how to create and use decorators in Python.
Sample Answer: In Python, a decorator is a function that takes another function as an argument and extends or modifies its behavior without changing its source code. Decorators are often used to add functionality such as logging, authentication, or memoization to functions or methods.
Here's an example of a simple decorator:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
In this example, the my_decorator function is used as a decorator to modify the behavior of the say_hello function.
What to Look For: Look for candidates who can explain decorators, demonstrate their usage, and provide clear examples. Ensure they understand the concept of function composition and the order of execution.
How to Answer: Differentiate between map(), filter(), and reduce() functions in terms of their purposes and use cases. Provide examples to illustrate each function's usage.
Sample Answer: map(), filter(), and reduce() are three important functions in Python for working with iterables.
numbers = [1, 2, 3, 4, 5]
squared = map(lambda x: x**2, numbers)
numbers = [1, 2, 3, 4, 5]
even_numbers = filter(lambda x: x % 2 == 0, numbers)
from functools import reduce
numbers = [1, 2, 3, 4, 5]
sum_of_numbers = reduce(lambda x, y: x + y, numbers)
What to Look For: Evaluate candidates' understanding of these functional programming concepts and their ability to provide clear explanations and examples for each function.
How to Answer: Explain the Global Interpreter Lock (GIL) as a mutex that allows only one thread to execute Python bytecode at a time. Discuss its impact on multithreading and its implications for CPU-bound and I/O-bound tasks.
Sample Answer: The Global Interpreter Lock (GIL) in Python is a mutex that allows only one thread to execute Python bytecode at a time, even on multi-core processors. This means that in a multi-threaded Python program, only one thread is actively executing Python code at any given moment, while others are waiting for their turn.
The GIL has a significant impact on multithreading in Python. It is beneficial for I/O-bound tasks where threads spend a lot of time waiting for external operations (e.g., reading from files or network sockets). However, it can be detrimental for CPU-bound tasks that require significant computational processing, as it prevents true parallel execution.
Developers often use multiprocessing instead of multithreading to leverage multiple CPU cores for CPU-bound tasks since each process has its own Python interpreter and memory space, avoiding the GIL limitations.
What to Look For: Assess candidates' understanding of the GIL, its impact on multithreading, and their ability to explain its consequences for different types of tasks.
How to Answer: Describe the process of creating and managing threads in Python using the threading module. Explain how to create threads, start them, and manage their execution.
Sample Answer: In Python, you can create and manage threads using the threading module. Here are the basic steps to create and manage threads:
These steps allow you to create and manage concurrent threads in Python.
What to Look For: Verify candidates' familiarity with thread creation, starting threads, and managing their execution using the threading module.
How to Answer: Describe Python's memory management process, including the role of reference counting and the garbage collector. Explain how cyclic references are handled.
Sample Answer: In Python, memory management is primarily based on reference counting and a cyclic garbage collector.
For example, consider two objects A and B, where A references B, and B references A. Without a garbage collector, these objects would never be deleted because their reference counts would never drop to zero. The cyclic garbage collector identifies and resolves such situations.
What to Look For: Assess candidates' understanding of memory management in Python, including reference counting and garbage collection, and their ability to explain how cyclic references are managed.
How to Answer: Explain the concept of Python generators and how they differ from regular functions. Discuss the use of the yield keyword in generator functions.
Sample Answer: Python generators are a type of iterable, similar to lists or tuples, but they are generated lazily as values are needed rather than storing all values in memory at once. Generators are defined using functions with the yield keyword. Here's how they differ from regular functions:
Here's an example of a simple generator function:
def countdown(n):
while n > 0:
yield n
n -= 1
# Usage
for num in countdown(5):
print(num)
In this example, the countdown generator yields values from n down to 1, one at a time, without storing the entire sequence in memory.
What to Look For: Evaluate candidates' understanding of generators, their ability to explain the difference between generators and regular functions, and their proficiency in using the yield keyword.
How to Answer: Explain the process of reading and writing binary files in Python, including the use of the open() function with different modes and the read() and write() methods.
Sample Answer: To read and write binary files in Python, you can use the open() function with the appropriate mode:
In these examples, "rb" mode is used to read, and "wb" mode is used to write binary data. The read() method reads the entire file, and the write() method writes binary data to the file.
What to Look For: Verify candidates' understanding of binary file handling in Python, including the use of modes and file operations.
How to Answer: Describe an efficient approach to read large text files line by line in Python, considering memory constraints. Mention the use of iterators or generator functions.
Sample Answer: To efficiently read large text files line by line in Python without loading the entire file into memory, you can use an iterator or a generator function. Here's an example using a generator function:
def read_large_file(file_path):
with open(file_path, "r") as file:
for line in file:
yield line
# Usage
for line in read_large_file("large_text_file.txt"):
# Process each line
In this example, the read_large_file generator function reads the file line by line, yielding each line to the caller. This approach is memory-efficient because it doesn't load the entire file into memory at once.
What to Look For: Assess candidates' ability to provide an efficient solution for reading large text files line by line, emphasizing the use of iterators or generators.
How to Answer: Explain the purpose of the try, except, and finally blocks in Python error handling. Describe how they work together to handle exceptions and ensure resource cleanup.
Sample Answer: In Python, the try, except, and finally blocks are used for error handling and ensuring resource cleanup.
Here's an example:
try:
# Code that may raise an exception
result = 10 / 0
except ZeroDivisionError:
# Handle the specific exception
print("Division by zero is not allowed.")
finally:
# Cleanup code (e.g., close files)
print("Cleanup code executed.")
In this example, if a ZeroDivisionError occurs, the control goes to the except block, and regardless of the outcome, the finally block ensures the cleanup code is executed.
What to Look For: Evaluate candidates' understanding of error handling using try, except, and finally blocks, and their ability to explain their purpose and usage.
How to Answer: Explain the steps to connect to a relational database in Python and mention popular libraries for database access, such as sqlite3, MySQLdb, psycopg2, or SQLAlchemy.
Sample Answer: To connect to a relational database in Python, you can follow these general steps:
Popular libraries for database access in Python include sqlite3 (for SQLite), MySQLdb (for MySQL), psycopg2 (for PostgreSQL), and SQLAlchemy (which supports multiple database systems). The choice of library depends on the specific database system you're working with and your project requirements.
What to Look For: Assess candidates' knowledge of database connectivity in Python, including the ability to import the appropriate library, establish connections, and perform basic database operations.
How to Answer: Explain SQL injection as a security vulnerability where malicious SQL queries are inserted into input fields, potentially leading to unauthorized access or data loss. Discuss preventive measures, such as using parameterized queries or ORM frameworks.
Sample Answer: SQL injection is a security vulnerability in which an attacker injects malicious SQL code into input fields of a web application or database query. This can lead to unauthorized access, data leakage, or data manipulation. Here's an example of a vulnerable query:
user_input = "'; DROP TABLE users; --"
query = f"SELECT * FROM products WHERE name = '{user_input}'"
To prevent SQL injection in Python applications, follow these best practices:
By following these practices, you can significantly reduce the risk of SQL injection in your Python applications.
What to Look For: Evaluate candidates' understanding of SQL injection, their ability to explain prevention methods, and whether they emphasize the importance of parameterized queries or ORM frameworks.
How to Answer: Explain the Global Interpreter Lock (GIL) as a mutex that allows only one thread to execute Python bytecode at a time. Discuss its impact on multithreading and its implications for CPU-bound and I/O-bound tasks.
Sample Answer: The Global Interpreter Lock (GIL) in Python is a mutex that allows only one thread to execute Python bytecode at a time, even on multi-core processors. This means that in a multi-threaded Python program, only one thread is actively executing Python code at any given moment, while others are waiting for their turn.
The GIL has a significant impact on multithreading in Python. It is beneficial for I/O-bound tasks where threads spend a lot of time waiting for external operations (e.g., reading from files or network sockets). However, it can be detrimental for CPU-bound tasks that require significant computational processing, as it prevents true parallel execution.
Developers often use multiprocessing instead of multithreading to leverage multiple CPU cores for CPU-bound tasks since each process has its own Python interpreter and memory space, avoiding the GIL limitations.
What to Look For: Assess candidates' understanding of the GIL, its impact on multithreading, and their ability to explain its consequences for different types of tasks.
How to Answer: Describe the process of creating and managing threads in Python using the threading module. Explain how to create threads, start them, and manage their execution.
Sample Answer: In Python, you can create and manage threads using the threading module. Here are the basic steps to create and manage threads:
These steps allow you to create and manage concurrent threads in Python.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
First, we will dive into the foundational aspects of Python that form the basis of your programming knowledge. These fundamental concepts are essential for any Python developer, and mastering them will provide you with a strong foundation for more advanced topics.
Data types in Python determine what kind of values a variable can hold. Understanding data types is crucial for effective data manipulation and type handling. Here are some key data types in Python:
Understanding and using these data types effectively is crucial for any Python programming task.
Control flow structures determine the order in which statements and blocks of code are executed. These structures are essential for writing logic and controlling program flow. Here are some key aspects of control flow in Python:
Mastery of control flow structures is vital for writing reliable and efficient Python code.
Functions and modules promote code reusability and organization. They allow you to break your code into smaller, manageable pieces and reuse code across different parts of your program.
By understanding functions and modules, you'll write cleaner, more modular, and more maintainable Python code.
Exception handling is essential for gracefully handling errors and exceptions that may occur during program execution. Properly managing exceptions ensures your code remains robust and resilient.
Effective exception handling is crucial for creating reliable software that can handle unexpected situations gracefully.
Object-oriented programming (OOP) is a powerful paradigm that allows you to model real-world entities and their interactions in your code.
Mastery of OOP principles empowers you to design and develop scalable, maintainable, and organized Python software.
Now, we'll explore advanced Python concepts that are essential for becoming a proficient Python developer. These concepts go beyond the basics and often play a significant role in writing efficient and maintainable Python code.
Decorators are a powerful Python feature that allows you to modify or enhance the behavior of functions or methods. They are widely used for tasks such as logging, authentication, and performance monitoring.
Generators are an efficient way to create iterators in Python. They enable you to work with large datasets without loading everything into memory at once. Topics covered here include:
Mastery of decorators and generators can significantly improve the quality and efficiency of your Python code.
Context managers provide a convenient way to manage resources, such as files or network connections, by automatically acquiring and releasing them. They are commonly used with the with statement.
Understanding context managers is essential for writing clean and resource-efficient code.
List comprehensions and generator expressions are concise and powerful techniques for creating lists and generators, respectively. They improve code readability and can lead to more efficient code.
These techniques simplify code and make it more Pythonic.
Duck typing is a dynamic typing concept in Python where the type or class of an object is determined by its behavior rather than its explicit type. This enables flexible and versatile coding.
Mastery of duck typing and polymorphism allows you to write code that can work with diverse data structures and objects.
Metaclasses are advanced Python features used for class customization. They allow you to control the creation and behavior of classes.
Metaclasses empower you to shape class behavior and design patterns in Python.
With a solid understanding of these advanced Python concepts, you'll be well-equipped to tackle complex programming challenges and write more elegant and efficient Python code.
We'll explore various data structures and algorithms in Python, providing you with a deeper understanding of how to use them effectively.
Lists are one of the most commonly used data structures in Python. They are mutable and can hold a collection of items, making them versatile for various tasks.
Tuples are similar to lists but are immutable, making them suitable for situations where data should not change.
Dictionaries are key-value pairs that allow for efficient data retrieval based on keys. Here's what you should grasp:
Sets are unordered collections of unique elements, and frozensets are their immutable counterparts.
Stacks and queues are abstract data types used for managing data in a particular order.
Linked lists and trees are fundamental data structures that play a significant role in various algorithms and applications.
Efficient sorting and searching algorithms are essential for optimizing data processing.
Here are some of the most influential Python libraries and frameworks that empower developers to build a wide range of applications efficiently.
NumPy (Numerical Python) and SciPy (Scientific Python) are essential libraries for scientific computing and data analysis.
Pandas is a powerful library for data manipulation and analysis.
Matplotlib and Seaborn are essential tools for creating data visualizations. Explore how to make your data come to life:
Django and Flask are popular Python web frameworks, each with its unique strengths. Explore their features and use cases:
TensorFlow and PyTorch are leading libraries for machine learning and deep learning.
These libraries and frameworks play pivotal roles in different domains, from scientific research to web development and artificial intelligence. Familiarizing yourself with them can significantly enhance your Python programming capabilities and open doors to exciting career opportunities.
Finally, we'll explore advanced Python topics that will empower you to tackle complex challenges and develop more sophisticated applications.
Concurrency and parallelism are essential concepts for improving the performance and responsiveness of Python applications.
Efficient file handling and input/output operations are crucial for interacting with data.
Regular expressions (regex) are powerful tools for text pattern matching and manipulation.
Web scraping allows you to extract data from websites, making it a valuable skill.
Database interaction is a crucial aspect of many Python applications. Dive into SQLAlchemy, a powerful SQL toolkit:
These advanced topics will elevate your Python programming skills and enable you to tackle more complex projects and challenges with confidence. Whether you're optimizing performance, handling data, parsing text, scraping the web, or working with databases, mastering these concepts will make you a more versatile and capable Python developer.
Below are some best practices that will help you write clean, maintainable, and efficient Python code, ensuring that your projects are well-organized and easy to collaborate on.
Adhering to a consistent code style is crucial for readability and maintainability. Python has its own style guide known as PEP 8 (Python Enhancement Proposal 8).
Writing tests for your code is essential for catching and preventing bugs early in the development process.
Effective debugging is a valuable skill for every developer. There are various debugging techniques and tools:
Clear and concise documentation is essential for code maintainability and collaboration.
Version control is critical for tracking changes to your code, collaborating with others, and safely managing project versions.
Mastering these Python best practices will not only make your code more professional and maintainable but also enhance your collaboration with other developers and contribute to a smoother development process.
Mastering Advanced Python Interview Questions is the key to opening doors to exciting career opportunities in the world of Python programming. By honing your skills in Python fundamentals, data structures, algorithms, and best practices, you'll be well-prepared to tackle challenging interviews with confidence. Remember, it's not just about getting the right answers; it's about demonstrating your problem-solving prowess and showcasing your ability to thrive in advanced Python roles.
As you embark on your journey to excel in Python interviews, keep in mind that practice, preparation, and continuous learning are your best allies. With dedication and the knowledge gained from this guide, you'll be better equipped to navigate the intricate landscape of Python interviews, leaving a lasting impression on potential employers and taking significant steps towards achieving your career goals. So, go ahead, tackle those advanced Python interview questions, and seize the opportunities that await you in the dynamic field of Python development.