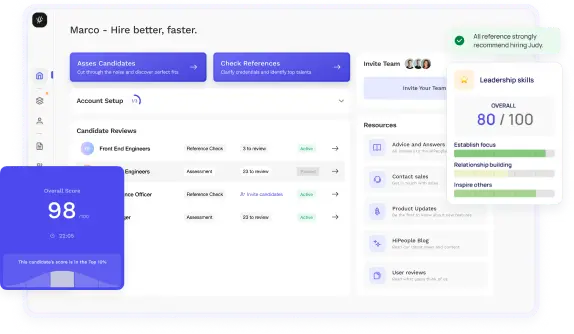
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
If you're preparing for a job interview that involves SQL, you've come to the right place. SQL (Structured Query Language) is a powerful language used for managing and manipulating relational databases, and having a solid understanding of SQL concepts is crucial for success in many technical roles.
In this guide, we'll cover everything you need to know to ace your SQL interview. We'll start with the basics, such as SQL syntax, retrieving data from databases, and working with functions and operators. Then, we'll delve into more advanced topics like query optimization, advanced SQL concepts, and interview question examples. By the end of this guide, you'll feel confident in your SQL skills and ready to tackle any interview question that comes your way.
Interviews can feel like a shot in the dark... unless you have the right data to guide you. Why waste time with generic questions and gut-feel decisions when you can unlock precision hiring with HiPeople?
Here’s how HiPeople changes the game:
Ready to transform your hiring process? 🚀 Book a demo today and see how HiPeople makes your interviews smarter, faster, and way more effective!
Before we dive into the interview questions, it's important to have a solid foundation in the basic concepts of SQL. This section will cover the fundamental principles and components of SQL, ensuring that you have a strong understanding of the language.
SQL, which stands for Structured Query Language, is a standard language used for managing and manipulating relational databases. It provides a set of commands and functions that allow you to interact with databases, perform various operations, and retrieve or modify data.
SQL is widely used in the industry for tasks such as querying databases, inserting, updating, and deleting data, creating and modifying database schemas, and more. It is a crucial skill for anyone working with databases, whether you're a database administrator, data analyst, or software developer.
To work effectively with SQL, it's important to understand the concept of relational databases. A relational database is a collection of related tables that store data in a structured manner. Each table represents a specific entity or concept, and the relationships between tables are defined by keys.
In a relational database, data is organized into rows and columns. Each row represents a record or instance of the entity, and each column represents a specific attribute or property of that entity. For example, in a database of employees, each row could represent an individual employee, while the columns would contain attributes like name, age, and department.
In SQL, data is stored in tables, which consist of rows and columns. Here's a breakdown of each component:
In SQL, data types define the type of data that can be stored in a column. Different database management systems (DBMS) support various data types, but here are some commonly used ones:
Constraints, on the other hand, are rules that define restrictions or conditions on the data stored in a table. Here are some commonly used constraints:
To interact with a database, SQL provides several statements for different operations. Here are the four fundamental SQL statements you'll encounter in interviews:
One of the primary tasks in SQL is retrieving data from a database. The ability to construct precise and efficient queries is essential for data analysis and reporting. In this section, we'll explore the SELECT statement and various techniques for filtering, sorting, and manipulating data.
The SELECT statement is the core of data retrieval in SQL. It allows you to specify which columns you want to retrieve from a table and apply conditions to filter the data. The basic syntax of a SELECT statement is as follows:
The WHERE clause is used to filter data based on specific conditions. It allows you to retrieve only the rows that meet the specified criteria. Here are some common operators used in the WHERE clause:
The ORDER BY clause is used to sort the retrieved data in a specified order. By default, it sorts data in ascending order. Here's an example of using the ORDER BY clause:
In some cases, you may want to limit the number of rows returned or retrieve data in chunks for pagination. SQL provides the LIMIT and OFFSET clauses for this purpose. Here's an example:
In many real-world scenarios, data is stored across multiple tables, and you'll often need to combine data from different tables to perform complex queries. SQL provides JOIN operations to accomplish this. Here are the most commonly used JOIN types:
To join tables, you need to specify the join condition using the ON keyword, which defines the columns used for the match.
SQL functions and operators allow you to perform calculations, manipulate strings, aggregate data, and work with dates and times. Understanding and utilizing these functions and operators effectively can enhance your ability to solve complex SQL problems. In this section, we'll explore the various categories of SQL functions and operators.
SQL functions can be categorized into different types based on their purpose. Here's an overview of the most common types:
To demonstrate the usage of SQL functions, let's consider some practical scenarios:
Calculating Total Sales: You can use the SUM function to calculate the total sales from a sales table. For example:
Formatting Names: You can use string functions to format names consistently. For example, to display names in uppercase:
Calculating Age: Using date functions, you can calculate someone's age based on their birth date. For example:
SQL provides various operators that allow you to perform arithmetic calculations, compare values, combine conditions, and manipulate strings. Here's an overview of the most commonly used operators:
Understanding how to use these operators effectively will enable you to build complex queries and solve intricate SQL problems.
To solve more complex problems, you often need to combine operators and functions within SQL queries. This allows you to perform calculations, apply conditions, and manipulate data in a single query. Here's an example of a complex query that involves multiple functions and operators:
In this example, we're combining the CONCAT function to merge the first and last names, the DATE_DIFF function to calculate age, and the logical AND operator to apply multiple conditions.
In addition to retrieving data, SQL allows you to manipulate data within tables using Data Manipulation Language (DML) statements. In this section, we'll explore the three primary DML statements: INSERT, UPDATE, and DELETE.
The INSERT statement is used to add new records into a table. It allows you to specify the values to be inserted into each column. Here's the basic syntax:
The UPDATE statement is used to modify existing data in a table. It allows you to update specific columns in one or more rows based on specified conditions. Here's an example:
The DELETE statement is used to remove records from a table. It allows you to delete specific rows based on specified conditions. Here's an example:
In addition to manipulating data, SQL allows you to modify the structure of database tables using the ALTER TABLE statement. Here are some common modifications:
When modifying table structures, it's important to be cautious and understand the potential impact on existing data and applications.
When manipulating data in SQL, it's crucial to follow best practices to ensure data integrity and performance. Here are some tips and considerations:
By adhering to these best practices, you can maintain data integrity, prevent data loss, and improve the efficiency of your data manipulation operations.
In addition to data manipulation, SQL provides a set of statements known as Data Definition Language (DDL) that allow you to create, modify, and delete database objects such as tables, indexes, and constraints. In this section, we'll explore the essential DDL statements and their applications.
To create a new database, you use the CREATE DATABASE statement. For example:
To create tables within a database, you use the CREATE TABLE statement. Here's an example:
To modify existing tables, you can use the following DDL statements:
Care should be taken when modifying table structures, as it can impact data integrity and the functionality of dependent applications.
Indexes play a vital role in optimizing query performance. They are data structures that provide quick access to specific rows in a table. By creating indexes on columns commonly used in search conditions or join operations, you can significantly improve query response times.
To create an index, you use the CREATE INDEX statement. Here's an example:
Indexes come with trade-offs, as they require additional storage space and incur overhead during data modification operations. Therefore, it's important to carefully analyze query patterns and select appropriate columns for indexing.
Constraints ensure data integrity and enforce rules on the values stored in tables. Here are some commonly used constraints:
To define constraints during table creation, you include them in the column definition. For example:
Constraints play a crucial role in maintaining data quality and preventing inconsistencies in the database.
Optimizing SQL queries and improving performance is essential for working with large datasets and ensuring efficient data retrieval. In this section, we'll explore the importance of query optimization, techniques to analyze query execution plans, indexing strategies, and optimization techniques.
Query optimization is the process of designing and structuring SQL queries to maximize efficiency and minimize response times. Optimized queries can significantly improve application performance, reduce resource consumption, and enhance user experience. It involves understanding the underlying database structure, analyzing query execution plans, and employing various optimization techniques.
To optimize SQL queries, it's essential to understand how the database executes them. Query execution plans provide insights into how the database engine processes a query and determines the most efficient way to retrieve data. By analyzing query execution plans, you can identify bottlenecks, inefficiencies, and potential areas for improvement.
Database management systems provide various tools and techniques to obtain query execution plans. For example, in MySQL, you can use the EXPLAIN keyword to retrieve the execution plan for a query. Other DBMS platforms have similar functionality.
Indexes play a critical role in query optimization. By creating indexes on columns used in search conditions or join operations, you can speed up data retrieval. Here are some indexing strategies and best practices to consider:
It's important to strike a balance between the number of indexes and the overhead they introduce. Creating too many indexes can lead to excessive disk space usage and increased maintenance costs.
In addition to indexes and execution plans, SQL provides query hints and optimization techniques to fine-tune query performance. Here are a few examples:
By employing these techniques and considering the specific characteristics of your database and queries, you can significantly improve query performance.
In this section, we'll explore advanced SQL concepts that are commonly encountered in interviews. These concepts, such as subqueries, common table expressions (CTEs), views, stored procedures, functions, and triggers, allow you to solve complex problems and build robust database systems.
A subquery, also known as an inner query or nested query, is a query nested within another query. Subqueries allow you to break down complex problems into smaller, more manageable parts and perform multiple operations within a single SQL statement.
Here are some common use cases for subqueries:
Subqueries can be used in SELECT, INSERT, UPDATE, and DELETE statements. They can also be used in combination with various clauses, such as WHERE, FROM, and HAVING.
Common Table Expressions (CTEs) provide a way to define temporary result sets within a SQL statement. CTEs are particularly useful when you need to reuse the result of a subquery multiple times or simplify complex queries.
Here's an example of using a CTE:
CTEs improve query readability, maintainability, and performance by allowing you to define reusable result sets and break down complex logic into smaller, more understandable parts.
A view is a virtual table derived from the result of a query. It allows you to encapsulate complex queries, define customized subsets of data, and present them as a single object. Views provide several benefits:
To create a view, you use the CREATE VIEW statement. For example:
Views are a powerful tool for improving the maintainability and security of your database system.
Stored procedures and functions are database objects that encapsulate a series of SQL statements and can be executed with a single call. They provide reusability, modularity, and code organization. Here's a breakdown of each:
Stored procedures and functions enhance code modularity, maintainability, and security by centralizing database logic and reducing redundancy.
Triggers are database objects that are automatically executed in response to specific events, such as insertions, updates, or deletions on a table. They enable you to enforce business rules, perform data validation, or maintain data integrity.
Triggers consist of three main parts:
Triggers can be powerful tools for enforcing data consistency, implementing complex business rules, and automating database operations.
In this section, we'll delve into SQL joins, which allow you to combine data from multiple tables based on a common column. Understanding different join types and the principles of relational algebra can significantly enhance your ability to work with complex datasets.
SQL supports different types of joins to combine data from multiple tables. Here are the four most commonly used join types:
By understanding these join types and their implications, you can efficiently combine data from multiple tables to retrieve meaningful information.
Relational algebra provides the theoretical foundation for SQL joins and database operations. It defines a set of operators to manipulate and combine relations (tables) to produce desired results.
Here are some key operators used in relational algebra:
These operators form the basis for SQL operations, including joins. Understanding relational algebra helps you grasp the underlying principles of SQL and effectively use its features.
To illustrate the practical applications of SQL joins, let's consider a few scenarios:
Retrieving Customer and Order Information: Suppose you have two tables, customers and orders, with a common column customer_id. You can use an INNER JOIN to retrieve customer information along with their corresponding orders:
Aggregating Data from Multiple Tables: Consider a scenario where you have a table products and a table sales with a common column product_id. You can use a LEFT JOIN and aggregate functions to retrieve the total sales for each product:
Combining Multiple Conditions: In some cases, you may need to combine multiple conditions in a join. For example, let's say you have a table employees and a table departments, and you want to retrieve employees from the "Sales" department who joined the company after a specific date:
By understanding SQL joins and how to use them effectively, you can retrieve and combine data from multiple tables to derive valuable insights.
How to Answer: Start by explaining that SQL (Structured Query Language) is a programming language used for managing and manipulating relational databases. Discuss its key features, such as:
Sample Answer: "SQL is a programming language designed for managing relational databases. Its key features include DDL for creating and modifying database objects, DML for querying and modifying data, DCL for managing permissions, data integrity enforcement, and transaction control. SQL ensures the efficient and secure management of structured data."
What to Look For: Look for a clear and concise explanation of SQL and its main features. The candidate should demonstrate a solid understanding of the language and its purpose in managing databases.
How to Answer: Explain the differences between these types of joins:
Discuss when to use each type based on the relationship between the tables and the desired result set.
Sample Answer: "An INNER JOIN returns rows with matching values in both tables, excluding non-matching rows. A LEFT JOIN returns all rows from the left table and matching rows from the right table, and a RIGHT JOIN does the opposite, returning all rows from the right table and matching rows from the left table. The choice of join type depends on the relationship between the tables and the desired result set."
What to Look For: Look for a clear explanation of the differences between the join types and a demonstration of when to use each type based on the context of the query.
How to Answer: Define a subquery as a nested query within another query and explain its purpose. Discuss common use cases, such as:
Highlight the importance of understanding subquery syntax and the potential impact on query performance.
Sample Answer: "A subquery is a query nested within another query. It allows us to filter data based on the results of another query, perform comparisons or calculations using subquery results, and create temporary result sets for further analysis. It's essential to understand subquery syntax and consider the potential impact on query performance."
What to Look For: Look for a clear explanation of subqueries and their purpose. The candidate should demonstrate an understanding of subquery syntax and discuss potential use cases.
How to Answer: Describe the steps to retrieve the top 5 highest-paid employees:
Highlight the importance of understanding the data and column structure before applying these steps.
Sample Answer: "To retrieve the top 5 highest-paid employees, I would use the ORDER BY clause to sort the employees by salary in descending order. Then, I would use the LIMIT clause to restrict the result set to the top 5 rows. It's crucial to understand the data and ensure the correct column is used for sorting."
What to Look For: Look for a logical and sequential explanation of the steps involved in retrieving the top 5 highest-paid employees. The candidate should demonstrate an understanding of the ORDER BY and LIMIT clauses and emphasize the importance of data understanding.
How to Answer: Explain the approach to identify duplicate records:
Emphasize the need to define the criteria for identifying duplicates based on the specific requirements of the table.
Sample Answer: "To find duplicate records in a table, I would use the GROUP BY clause to group rows based on the columns that may contain duplicates. Then, I would use the HAVING clause with a COUNT() function to filter groups with a count greater than 1. Finally, I would select the columns involved to retrieve the duplicate records. It's important to define the criteria for identifying duplicates based on the table's requirements."
What to Look For: Look for a clear explanation of the approach to finding duplicate records and an understanding of the GROUP BY and HAVING clauses. The candidate should highlight the importance of defining the criteria for identifying duplicates.
How to Answer: Describe the steps to calculate the average order value for each customer:
Highlight the importance of understanding the table structure and ensuring the correct column is used for grouping.
Sample Answer: "To calculate the average order value for each customer, I would use the GROUP BY clause to group rows by the customer column. Then, I would use the AVG() function to calculate the average order value for each group. Finally, I would retrieve the customer column and the calculated average. It's crucial to understand the table structure and ensure the correct column is used for grouping."
What to Look For: Look for a logical explanation of the steps involved in calculating the average order value. The candidate should demonstrate an understanding of the GROUP BY clause, AVG() function, and the importance of data understanding.
How to Answer: Describe the approach to explain complex SQL concepts or queries to non-technical individuals:
Emphasize the importance of tailoring explanations to the audience's knowledge level and providing relatable examples.
Sample Answer: "When explaining a complex SQL concept or query to a non-technical person, I would start with a high-level overview of its purpose. Then, I would use analogies or real-world examples to illustrate the concept or query. I would simplify technical terminology and provide clear explanations step-by-step. Throughout the explanation, I would encourage questions and actively listen to ensure understanding. It's important to tailor the explanation to the audience's knowledge level and provide relatable examples."
What to Look For: Look for an effective and empathetic approach to explaining complex SQL concepts or queries to non-technical individuals. The candidate should demonstrate clear communication skills and the ability to adapt explanations to the audience.
How to Answer: Outline the steps to handle a disagreement or conflict with a team member during a SQL project:
Highlight the importance of focusing on the project's success and maintaining positive working relationships.
Sample Answer: "If a disagreement or conflict arises with a team member during a SQL project, I would maintain a calm and respectful demeanor. I would actively listen to the team member's perspective and try to understand their point of view. I would seek a compromise or solution through open and constructive communication. If necessary, I would involve a mediator to help resolve the conflict. Throughout the process, I would prioritize the success of the project and strive to maintain positive working relationships."
What to Look For: Look for a mature and collaborative approach to handling conflicts within a team. The candidate should demonstrate effective communication skills, empathy, and a focus on problem-solving.
How to Answer: Describe the approach to explaining a complex SQL query or concept in a team meeting:
Emphasize the importance of clarity, engaging presentation skills, and fostering an inclusive environment for questions and discussion.
Sample Answer: "When explaining a complex SQL query or concept in a team meeting, I would prepare a concise and organized presentation. I would use visual aids, such as diagrams or examples, to enhance understanding. I would break down the query or concept into manageable parts and explain each part step-by-step. Throughout the presentation, I would encourage questions and provide clarification as needed. It's important to prioritize clarity, engage the team with effective presentation skills, and foster an inclusive environment for questions and discussion."
What to Look For: Look for a well-structured approach to explaining complex SQL queries or concepts in a team setting. The candidate should demonstrate effective presentation skills, clarity in communication, and the ability to foster a collaborative environment.
How to Answer: Outline the steps to optimize a slow-performing SQL query:
Highlight the importance of benchmarking and testing the optimized query to ensure improvements.
Sample Answer: "To optimize a slow-performing SQL query, I would start by analyzing the query execution plan to identify bottlenecks. Then, I would ensure appropriate indexing on columns used in the query's conditions and joins. I would optimize the query's structure by eliminating unnecessary operations and rewriting complex subqueries if possible. If applicable, I would consider caching or materializing intermediate results. Finally, I would benchmark and test the optimized query to ensure improvements. It's crucial to understand the query's performance characteristics and employ appropriate optimization techniques."
What to Look For: Look for a logical and systematic approach to optimizing slow-performing SQL queries. The candidate should demonstrate an understanding of query execution plans, indexing, query structure optimization, and the importance of testing.
How to Answer: Explain the approach to identify and resolve a data inconsistency issue in a database:
Highlight the importance of ensuring data integrity and conducting thorough testing after resolving the issue.
Sample Answer: "To identify and resolve a data inconsistency issue in a database, I would start by analyzing the nature and extent of the inconsistency. Then, I would identify the root cause, which could be erroneous data entry or a software bug. Based on the analysis, I would develop a plan to rectify the inconsistency, considering data dependencies and the impact on related records. Finally, I would implement the necessary data modifications or system fixes. It's important to ensure data integrity throughout the process and conduct thorough testing after resolving the issue."
What to Look For: Look for a structured approach to identifying and resolving data inconsistency issues. The candidate should demonstrate problem-solving skills, an understanding of data dependencies, and an emphasis on data integrity and testing.
How to Answer: Describe the steps to troubleshoot a database connectivity issue:
Highlight the importance of systematic troubleshooting, documentation, and collaboration with system administrators if necessary.
Sample Answer: "To troubleshoot a database connectivity issue, I would start by verifying network connectivity and ensuring that the database server is accessible. Then, I would check the database credentials and authentication settings to ensure they are correct. Next, I would test the database connection using command-line tools or connection libraries specific to the database system. If necessary, I would review the database logs and error messages for any relevant information. Throughout the troubleshooting process, I would follow a systematic approach, document my steps and findings, and collaborate with system administrators if needed."
What to Look For: Look for a logical and systematic troubleshooting approach to database connectivity issues. The candidate should demonstrate an understanding of network connectivity, authentication settings, database logs, and error message analysis.
How to Answer: Describe the methods you use to stay updated with the latest trends and advancements in SQL:
Highlight the importance of continuous learning and the ability to adapt to evolving technologies and best practices.
Sample Answer: "To stay updated with the latest trends and advancements in SQL, I regularly read industry blogs, forums, and publications. I follow SQL experts and thought leaders on social media to learn from their insights. I actively participate in online communities and forums related to SQL, where I can engage in discussions and share knowledge. Additionally, I make an effort to attend conferences, webinars, or workshops that focus on SQL and database technologies. Continuous learning is essential in the fast-paced world of technology, and staying updated allows me to adapt to evolving best practices and technologies."
What to Look For: Look for a proactive approach to staying updated with SQL trends and advancements. The candidate should demonstrate a passion for continuous learning, self-improvement, and adaptability.
How to Answer: Share an example of a challenging SQL problem you faced and describe how you solved it:
Highlight the problem-solving skills and critical thinking involved in finding a resolution.
Sample Answer: "One challenging SQL problem I encountered involved optimizing a complex query that was running slowly. To solve it, I began by analyzing the query execution plan and identified areas for improvement. I noticed that the query had several subqueries that were executing multiple times, leading to performance issues. I rewrote the query to eliminate redundant subqueries and utilized temporary tables to cache intermediate results. Additionally, I reviewed the table indexing and made necessary adjustments to improve query performance. The optimized query resulted in a significant reduction in execution time and improved overall system performance."
What to Look For: Look for a clear and concise description of a challenging SQL problem and a well-structured approach to finding a solution. The candidate should demonstrate problem-solving skills, critical thinking, and the ability to optimize queries for performance.
How to Answer: Describe your approach to learning new database management systems or technologies:
Highlight the importance of adaptability, resourcefulness, and the ability to apply existing knowledge to new technologies.
Sample Answer: "When faced with learning a new database management system or technology, I start by conducting research and gathering information about its features and capabilities. I leverage documentation, tutorials, and online resources to gain a foundational understanding. Additionally, I set up a personal sandbox environment where I can practice and experiment with the new system or technology. I find it beneficial to collaborate with colleagues or join user groups to engage in shared learning experiences. I believe in applying existing knowledge and experience to new technologies, which helps me quickly grasp concepts and adapt to the new environment."
What to Look For: Look for a proactive and adaptable approach to learning new database management systems or technologies. The candidate should demonstrate resourcefulness, a willingness to practice hands-on, and the ability to leverage existing knowledge for quicker understanding.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Congratulations! You've reached the end of the Ultimate Guide to SQL Interview Questions. Throughout this comprehensive guide, we've covered the fundamental concepts of SQL, retrieving data, manipulating data, query optimization, advanced SQL concepts, and provided numerous interview question examples with solutions.
By mastering the concepts and practicing with the examples provided, you'll be well-prepared to tackle any SQL interview question that comes your way. Remember to continue practicing and applying your SQL skills to real-world scenarios to further enhance your expertise.
Good luck with your SQL interviews, and may your database queries be optimized and your data be in perfect harmony!