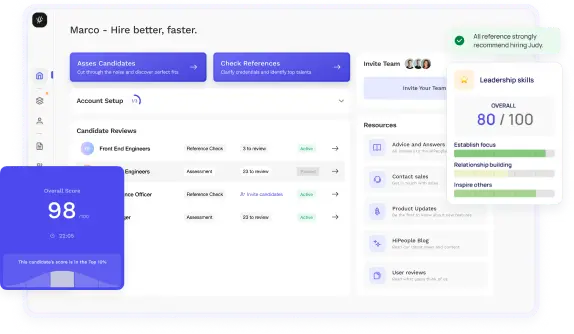
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Have you ever wondered how to approach those complex coding interview questions that seem to demand a mix of technical prowess and creative problem-solving? In this guide, we'll unravel the mystery behind coding interview questions, equipping you with the strategies, insights, and practical knowledge to not just solve these challenges, but to excel at them.
From mastering fundamental data structures and algorithms to fine-tuning your communication skills, get ready to embark on a journey that transforms these intricate puzzles into opportunities for growth and success.
Coding interviews are a crucial part of the hiring process for technical roles, especially in the realm of software development and engineering. These interviews assess a candidate's problem-solving skills, coding proficiency, algorithmic knowledge, and ability to think critically under pressure.
During a coding interview, candidates are presented with coding challenges that range from algorithmic puzzles to real-world coding problems. The goal is not only to arrive at a correct solution but to demonstrate a clear thought process, efficient coding practices, and effective communication of one's approach.
Coding interviews offer employers insight into a candidate's technical abilities and their compatibility with the company's work culture and team dynamics.
Coding interviews serve as a window for employers to gauge your problem-solving prowess and how well you can apply your technical knowledge to real-world scenarios. They help companies identify candidates who can contribute to their development teams and solve complex challenges. Mastering coding interviews increases your chances of standing out among other applicants.
Coding interviews come in various formats. The two main types are:
Coding interviews evaluate a range of skills, including:
Preparation is key to succeeding in coding interviews. Here's a roadmap to guide your preparation:
Before you dive into technical and behavioral preparation, it's crucial to understand the interview process itself. Let's explore what you can expect during coding interviews and how you can navigate each stage effectively.
Technical interviews often involve solving coding problems under pressure. To excel, keep these tips in mind:
Behavioral interviews assess your soft skills, cultural fit, and how well you communicate. Here's how to prepare:
Technical interviews can take place on a whiteboard or online platform. Each has its nuances:
Remember, the key to mastering the interview process is consistent practice, continuous learning, and honing your problem-solving and communication skills.
Let's dive deeper into technical interview preparation, starting with understanding essential data structures and algorithms. Get ready to strengthen your foundation in these crucial concepts.
Data structures are the building blocks of problem-solving. They determine how we store and manipulate data efficiently. Let's explore some fundamental data structures:
Arrays are collections of elements, each identified by an index or key. Strings are sequences of characters. They are versatile and form the basis for many algorithms.
Example: Finding the maximum element in an array.
def find_max_element(arr):
max_element = arr[0]
for element in arr:
if element > max_element:
max_element = element
return max_element
Linked lists consist of nodes, where each node contains data and a reference to the next node. Linked lists are essential for dynamic data structures and tasks like representing social networks.
Example: Reversing a linked list.
class Node:
def __init__(self, data):
self.data = data
self.next = None
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
Stacks (Last In, First Out) and queues (First In, First Out) are linear data structures that operate on a principle of order. They are crucial for tasks like managing function calls and breadth-first search.
Example: Implementing a stack.
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
Trees have a hierarchical structure with nodes and edges. Graphs, more general than trees, consist of nodes connected by edges. Trees are used in data storage, while graphs model relationships.
Example: Implementing a binary search tree.
class TreeNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, key):
self.root = self._insert_recursive(self.root, key)
def _insert_recursive(self, root, key):
if not root:
return TreeNode(key)
if key < root.key:
root.left = self._insert_recursive(root.left, key)
else:
root.right = self._insert_recursive(root.right, key)
return root
Hash tables use hash functions to map keys to values, allowing efficient data retrieval. They are used for tasks like caching, indexing, and implementing dictionaries.
Example: Implementing a basic hash table.
class HashTable:
def __init__(self, size):
self.size = size
self.table = [None] * size
def hash_function(self, key):
return key % self.size
def insert(self, key, value):
index = self.hash_function(key)
self.table[index] = value
def get(self, key):
index = self.hash_function(key)
return self.table[index]
Algorithms are step-by-step instructions for solving problems. Here are essential algorithmic techniques:
Searching algorithms find a specific element, while sorting algorithms arrange elements in a specific order.
Example: Binary search algorithm.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = left + (right - left) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
Recursion involves solving problems by breaking them into smaller instances of the same problem. Backtracking is a specific form of recursion used for exhaustive searching.
Example: Solving the Tower of Hanoi problem using recursion.
def tower_of_hanoi(n, source, auxiliary, target):
if n > 0:
tower_of_hanoi(n - 1, source, target, auxiliary)
print(f"Move disk {n} from {source} to {target}")
tower_of_hanoi(n - 1, auxiliary, source, target)
Dynamic programming involves breaking a problem into smaller subproblems and caching their solutions to avoid redundant calculations.
Example: Calculating the nth Fibonacci number using dynamic programming.
def fibonacci(n):
fib = [0, 1]
for i in range(2, n + 1):
fib.append(fib[i - 1] + fib[i - 2])
return fib[n]
Greedy algorithms make locally optimal choices at each step to find a global optimum.
Example: The coin change problem solved using a greedy algorithm.
def coin_change_greedy(coins, amount):
coins.sort(reverse=True)
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
if amount == 0:
return count
return -1
Graph algorithms traverse and analyze graphs to solve various problems.
Example: Implementing breadth-first search (BFS) in a graph.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
while queue:
node = queue.popleft()
if node not in visited:
visited.add(node)
print(node, end=" ")
queue.extend(graph[node] - visited)
Before jumping into coding, it's essential to have a structured approach to problem-solving:
Writing clean, efficient, and readable code not only impresses interviewers but also helps you maintain and debug your solutions effectively. Let's explore essential coding best practices.
Readable code is easy to understand and maintain. Follow these practices:
Analyzing the time and space complexity of your code helps you understand its efficiency. The Big O notation is a common way to express complexity:
Test-driven development (TDD) involves writing tests before writing the actual code. This approach ensures that your code meets requirements and avoids regressions:
Modular and reusable code promotes efficient development and maintenance:
By adopting these coding best practices, you'll not only impress interviewers but also become a more proficient and efficient coder.
Before jumping into coding, it's essential to have a structured approach to problem-solving:
Behavioral interviews assess your soft skills, communication, and cultural fit. Let's delve into strategies for mastering this crucial aspect of the interview.
Prepare for common behavioral questions like:
The STAR method (Situation, Task, Action, Result) helps structure your answers:
Behavioral interviews gauge your soft skills. Showcase qualities like adaptability, leadership, teamwork, and problem-solving through real examples from your experiences.
Technical challenges during interviews can be daunting. Let's explore strategies for dealing with difficult problems and handling mental blocks.
Break down complex problems into smaller components. Focus on solving one part at a time. If you're stuck, move on to another problem and return later with a fresh perspective.
Mental blocks are normal. If you encounter one, take a deep breath and step back. Revisit the problem from a different angle or tackle an easier problem to regain confidence.
Don't hesitate to ask interviewers for clarification if you're unsure about a problem. They value your thought process, and clarifications demonstrate your commitment to solving the problem correctly.
How to Answer: To solve this question, you can use the formula to find the sum of all integers from 1 to n. Then subtract the sum of the given array. The difference is the missing number.
Sample Answer:
def find_missing_number(nums):
n = len(nums) + 1
total_sum = (n * (n + 1)) // 2
actual_sum = sum(nums)
missing_number = total_sum - actual_sum
return missing_number
What to Look For: Look for candidates who can efficiently calculate the missing number using mathematical principles. Pay attention to their use of appropriate formulas and coding techniques.
How to Answer: Candidates should compare the sorted versions of two strings to determine if they are permutations of each other. If the sorted strings match, the strings are permutations.
Sample Answer:
def check_permutation(str1, str2):
return sorted(str1) == sorted(str2)
What to Look For: Evaluate candidates' understanding of string manipulations and sorting. Strong candidates will implement the comparison correctly and optimize their code for efficiency.
How to Answer: Implement Floyd's cycle-finding algorithm, also known as the "tortoise and hare" algorithm. Move one pointer (tortoise) one step at a time and another pointer (hare) two steps at a time. If they meet, there's a cycle.
Sample Answer:
class ListNode:
def __init__(self, val):
self.val = val
self.next = None
def has_cycle(head):
tortoise = head
hare = head
while hare and hare.next:
tortoise = tortoise.next
hare = hare.next.next
if tortoise == hare:
return True
return False
What to Look For: Assess candidates' familiarity with linked list manipulation and cycle detection algorithms. Look for clear and well-structured implementations.
How to Answer: Perform an in-order traversal of the binary tree. The sequence of values should be in ascending order for a valid BST.
Sample Answer:
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def is_valid_BST(root):
def in_order_traversal(node, prev):
if not node:
return True
if not in_order_traversal(node.left, prev):
return False
if node.val <= prev[0]:
return False
prev[0] = node.val
return in_order_traversal(node.right, prev)
prev = [-float('inf')]
return in_order_traversal(root, prev)
What to Look For: Evaluate candidates' understanding of binary trees and in-order traversal. Look for their ability to correctly validate the BST properties.
How to Answer: Merge the two sorted arrays by comparing elements and placing them in the correct order.
Sample Answer:
def merge_sorted_arrays(nums1, nums2):
merged = []
i = j = 0
while i < len(nums1) and j < len(nums2):
if nums1[i] < nums2[j]:
merged.append(nums1[i])
i += 1
else:
merged.append(nums2[j])
j += 1
merged.extend(nums1[i:])
merged.extend(nums2[j:])
return merged
What to Look For: Look for candidates who can effectively merge two sorted arrays in linear time. Pay attention to their coding style and handling of edge cases.
How to Answer: Use a recursive approach to generate all valid combinations of parentheses. Keep track of the open and close parentheses count to ensure validity.
Sample Answer:
def generate_parentheses(n):
def backtrack(s, left, right):
if len(s) == 2 * n:
combinations.append(s)
return
if left < n:
backtrack(s + '(', left + 1, right)
if right < left:
backtrack(s + ')', left, right + 1)
combinations = []
backtrack('', 0, 0)
return combinations
What to Look For: Evaluate candidates' ability to use recursion and backtracking to solve complex combinatorial problems. Look for clean code and correct handling of parentheses balance.
How to Answer: Use dynamic programming to calculate the number of ways to reach the top of the stairs. Each step can be reached from the previous two steps.
Sample Answer:
def climb_stairs(n):
if n <= 2:
return n
dp = [0] * (n + 1)
dp[1] = 1
dp[2] = 2
for i in range(3, n + 1):
dp[i] = dp[i - 1] + dp[i - 2]
return dp[n]
What to Look For: Assess candidates' grasp of dynamic programming concepts and their ability to solve problems with optimal substructure. Look for a clear understanding of memoization.
How to Answer: Use a greedy algorithm to select the largest coin denomination that fits within the remaining amount. Repeat until the amount becomes zero.
Sample Answer:
def coin_change(coins, amount):
coins.sort(reverse=True)
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
What to Look For: Evaluate candidates' ability to apply greedy algorithms to optimization problems. Look for clear logic and handling of edge cases.
How to Answer: Use Breadth-First Search (BFS) to find the shortest path in a binary matrix. Traverse neighboring cells and keep track of visited cells.
Sample Answer:
def shortest_path_binary_matrix(grid):
n = len(grid)
if grid[0][0] == 1 or grid[n - 1][n - 1] == 1:
return -1
queue = [(0, 0, 1)]
visited = set([(0, 0)])
while queue:
x, y, steps = queue.pop(0)
if x == n - 1 and y == n - 1:
return steps
for dx, dy in [(1, 0), (-1, 0), (0, 1), (0, -1), (1, 1), (1, -1), (-1, 1), (-1, -1)]:
nx, ny = x + dx, y + dy
if 0 <= nx < n and 0 <= ny < n and grid[nx][ny] == 0 and (nx, ny) not in visited:
visited.add((nx, ny))
queue.append((nx, ny, steps + 1))
return -1
What to Look For: Look for candidates who can implement graph traversal algorithms like BFS efficiently. Evaluate their understanding of grid-based problems and pathfinding.
How to Answer: When designing a parking lot, start by defining the essential components such as parking spots, vehicles, and payment systems. Consider factors like the layout, capacity, vehicle types, and payment methods. Outline the interactions between these components to create a comprehensive system.
Sample Answer:Designing a parking lot involves several key components. We need to define the parking spots, which can be categorized based on vehicle size. Each spot should have a status (occupied or vacant) and possibly a location identifier. The parking lot should have an entrance and exit with barriers or gates that can be controlled electronically.
For payment systems, customers can pay using mobile apps, credit cards, or cash at automated kiosks. The system should be able to track the duration of parking and calculate fees based on hourly rates. Additionally, there should be a management interface for administrators to monitor occupancy and revenue.
What to Look For: Evaluate candidates' ability to break down complex systems into manageable components. Look for their understanding of system design principles and the consideration of various user interactions.
How to Answer: Implement Dijkstra's algorithm to find the shortest path between two nodes in a weighted graph. Maintain a priority queue to explore nodes with the smallest distance first.
Sample Answer:
from heapq import heappop, heappush
def shortest_path(graph, start, end):
distances = {node: float('inf') for node in graph}
distances[start] = 0
heap = [(0, start)]
while heap:
current_distance, current_node = heappop(heap)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heappush(heap, (distance, neighbor))
return distances[end]
What to Look For: Assess candidates' understanding of graph theory and their ability to implement graph algorithms like Dijkstra's algorithm.
How to Answer: Discuss the architecture of a social media feed. Consider how to gather, organize, and display posts to users based on relevance and chronological order.
Sample Answer:Designing a social media feed involves multiple components. We need to fetch posts from users' connections, apply an algorithm to prioritize posts based on relevance and user interactions, and display them in a user-friendly manner. A content recommendation engine can consider factors like user preferences, engagement history, and post popularity to curate the feed.
To handle scalability, we can employ distributed databases to manage user data and posts. Caching mechanisms can enhance feed retrieval speed. Real-time updates can be achieved through WebSocket connections, ensuring users receive new posts as they are created.
What to Look For: Evaluate candidates' ability to design complex systems, considering data retrieval, algorithms, and real-time updates.
How to Answer: Discuss the design of an online shopping cart system. Define classes for products, cart items, and users. Implement methods for adding/removing items, calculating totals, and processing orders.
Sample Answer:Designing an online shopping cart involves several classes. We can have a Product class with attributes like name, price, and inventory. The CartItem class holds a reference to a product and quantity. Users can add and remove items from the cart, and the cart should calculate the total cost.
When processing orders, we can create an Order class that contains cart items and user details. This class can calculate the final order total and handle payment processing. Additionally, we can implement inventory management to update product quantities after successful orders.
What to Look For: Look for candidates who can design object-oriented systems and implement relationships between classes.
How to Answer: Use dynamic programming to find the longest palindromic substring in a given string. Create a 2D table to track whether substrings are palindromic.
Sample Answer:
def longest_palindromic_substring(s):
n = len(s)
dp = [[False] * n for _ in range(n)]
start = max_length = 0
for i in range(n):
dp[i][i] = True
max_length = 1
for length in range(2, n + 1):
for i in range(n - length + 1):
j = i + length - 1
if s[i] == s[j]:
if length == 2 or dp[i + 1][j - 1]:
dp[i][j] = True
if length > max_length:
max_length = length
start = i
return s[start:start + max_length]
What to Look For: Evaluate candidates' understanding of dynamic programming and their ability to apply it to string manipulation problems.
How to Answer: Given an array where every element appears twice except for one, find the single element. Use XOR operation to cancel out pairs.
Sample Answer:
def single_number(nums):
result = 0
for num in nums:
result ^= num
return result
What to Look For: Assess candidates' grasp of bit manipulation techniques and their ability to efficiently solve XOR-related problems.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
On-site interviews provide a unique opportunity to interact with interviewers and teams. Here's how to prepare effectively.
Know the interview location, schedule, and any required documentation. Familiarize yourself with the company culture to adapt your behavior accordingly.
Dress professionally to make a positive impression. Respectful behavior and active engagement during the interview showcase your professionalism.
Engage with interviewers and team members with enthusiasm and curiosity. Ask thoughtful questions to demonstrate your genuine interest in the company and role.
The journey doesn't end after the interview. Here's how to navigate the crucial post-interview phase.
Take time to reflect on the interview. Consider what went well and areas where you can improve. Reflecting enhances your self-awareness and growth.
Evaluate your performance objectively. Celebrate your successes and learn from any mistakes you made. This analysis guides your preparation for future interviews.
Coding interviews are a learning process. Continue practicing, refining your skills, and seeking feedback. Every interview experience contributes to your growth.
With these strategies, you're well-equipped to master coding interviews. Remember that practice, preparation, and perseverance are key to your success.
In the journey to mastering coding interviews, you've embarked on a path of continuous growth, learning, and preparation. By delving into the intricacies of various technical concepts, problem-solving strategies, and behavioral skills, you've equipped yourself with the tools needed to confidently tackle any coding interview that comes your way. Remember that success in coding interviews isn't just about memorizing solutions; it's about understanding the underlying principles and approaches that allow you to navigate complex challenges with creativity and innovation.
Through the exploration of fundamental data structures and algorithms, you've gained the ability to break down intricate problems into manageable components and devise efficient solutions. Moreover, by honing your soft skills, you're prepared to communicate your thought processes, work collaboratively in team projects, and demonstrate your adaptability and growth mindset. As you move forward in your coding interview journey, don't forget that every challenge you encounter is an opportunity for growth. Embrace each experience as a chance to refine your skills, gain insights, and demonstrate your unique problem-solving abilities.
With determination, practice, and the knowledge you've gained from this guide, you're well on your way to excelling in coding interviews and realizing your full potential as a skilled developer.