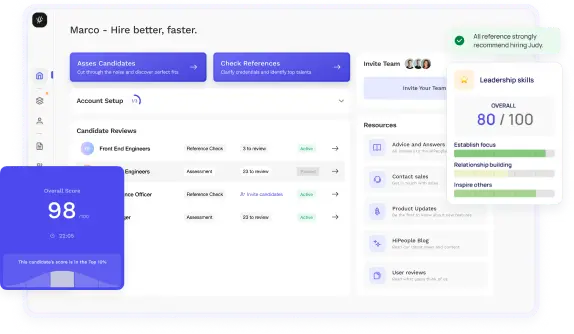
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to conquer the challenges of Tricky Java Interview Questions? Whether you're aiming for that dream job or seeking to level up your Java skills, this guide has you covered.
Dive into the world of complex Java queries, unravel their intricacies, and emerge as a confident and proficient Java developer. Let's unravel the secrets to mastering those tricky interview questions!
Tricky Java interview questions are not your typical run-of-the-mill inquiries. These questions are designed to test your in-depth knowledge of Java, problem-solving skills, and ability to think critically under pressure. They often go beyond the basics and challenge you to demonstrate a deep understanding of the language, its nuances, and its application in real-world scenarios.
Java interviews hold significant importance for both job seekers and employers.
Preparing for tricky Java interviews requires a structured and focused approach. Here are some steps to help you get ready:
Java interviews come in various formats, each with its own focus and objectives. Here's an overview of common interview formats you might encounter:
Understanding these interview formats can help you tailor your preparation to the specific requirements of your upcoming Java interview.
Let's dive into the fundamental concepts of Java that are crucial for acing those tricky interviews.
Object-oriented programming (OOP) is the backbone of Java. It's essential to understand how objects and classes work together.
In Java, everything revolves around classes and objects. A class is a blueprint for creating objects, while objects are instances of classes. Here's a simple example:
class Car {
String brand;
int year;
void start() {
System.out.println("Car started!");
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.brand = "Toyota";
myCar.year = 2020;
myCar.start();
}
}
In this example, we define a Car class with attributes brand and year, along with a start method. We then create an instance of the Car class and use it to access the class's members.
Inheritance allows one class to inherit the attributes and methods of another class. Polymorphism enables objects to take on multiple forms.
class Animal {
void makeSound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Woof!");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.makeSound(); // Outputs "Woof!"
}
}
Here, the Dog class inherits from the Animal class and overrides the makeSound method to provide a specific implementation.
Java supports various data types, which determine the kind of data that can be stored in a variable.
int age = 25;
double salary = 55000.50;
char grade = 'A';
boolean isJavaFun = true;
Reference data types hold references (memory addresses) to objects. Common reference data types include arrays, strings, and user-defined classes.
String name = "John";
int[] numbers = {1, 2, 3, 4, 5};
Control flow statements allow you to control the execution of your Java code.
Conditional statements, like if, else if, and else, help you make decisions in your code.
int score = 85;
if (score >= 90) {
System.out.println("Excellent!");
} else if (score >= 70) {
System.out.println("Good job!");
} else {
System.out.println("Keep practicing.");
}
Looping statements, including for, while, and do-while, allow you to repeat tasks.
for (int i = 1; i <= 5; i++) {
System.out.println("Iteration " + i);
}
int count = 0;
while (count < 3) {
System.out.println("Count: " + count);
count++;
}
Exception handling in Java is crucial for robust and error-free code.
try {
// Code that may throw an exception
int result = 10 / 0; // This will throw an ArithmeticException
} catch (ArithmeticException e) {
// Handle the exception
System.out.println("An error occurred: " + e.getMessage());
}
You can create custom exceptions to handle specific error scenarios effectively.
class MyCustomException extends Exception {
public MyCustomException(String message) {
super(message);
}
}
public class Main {
public static void main(String[] args) {
try {
throw new MyCustomException("This is a custom exception.");
} catch (MyCustomException e) {
System.out.println("Caught custom exception: " + e.getMessage());
}
}
}
Multithreading allows Java programs to execute multiple threads concurrently.
Input and output operations are essential for interacting with external data sources.
How to Answer:Candidates should explain that == compares object references for equality, while .equals() is a method used to compare the content or value of objects. It's essential to mention that .equals() can be overridden by custom classes to provide a meaningful comparison based on object attributes.
Sample Answer:"== compares object references, checking if they point to the same memory location. In contrast, .equals() compares the actual content of objects. For instance, when comparing strings, .equals() checks if the character sequences are identical."
What to Look For:Look for candidates who understand the fundamental distinction between reference equality and content equality. They should also demonstrate knowledge of when and how to override the .equals() method in custom classes.
How to Answer:Candidates should highlight that ArrayList is backed by an array, provides fast random access, but has slower insertions and deletions. LinkedList uses a doubly linked list, excels in insertions/deletions, but is slower for random access. They should discuss use cases for each data structure.
Sample Answer:"ArrayList is based on an array, allowing fast random access. However, insertions and deletions are slower due to array resizing. LinkedList uses a doubly linked list, making insertions and deletions faster, but accessing elements sequentially is more efficient."
What to Look For:Look for candidates who can articulate the trade-offs between ArrayList and LinkedList and demonstrate when to use each data structure based on specific requirements.
How to Answer:Candidates should describe that static denotes class-level members (variables or methods) that belong to the class, not instances. They should mention that static members can be accessed without creating an object of the class and are shared across all instances of the class.
Sample Answer:"The static keyword is used to define class-level members in Java. These members belong to the class itself, not to instances of the class. This means you can access static members without creating objects of the class, and changes to static variables are shared among all instances."
What to Look For:Look for candidates who can clearly explain the concept of static and provide examples of when and why it's useful, such as for constants or utility methods.
How to Answer:Candidates should explain that an abstract class can have both abstract (unimplemented) and concrete (implemented) methods, while an interface can only have abstract methods. They should also mention that a class can implement multiple interfaces but inherit from only one abstract class.
Sample Answer:"An abstract class can have both abstract and concrete methods, while an interface can only contain abstract methods. A class can implement multiple interfaces, but it can inherit from only one abstract class."
What to Look For:Look for candidates who can clearly articulate the distinctions between abstract classes and interfaces, understand their use cases, and explain when to prefer one over the other.
How to Answer:Candidates should discuss that Java supports multiple inheritance through interfaces, allowing a class to implement multiple interfaces. They should explain the "diamond problem" as a naming conflict when a class inherits methods with the same signature from multiple interfaces and how Java resolves it using interface method implementation.
Sample Answer:"Java supports multiple inheritance through interfaces, where a class can implement multiple interfaces. The 'diamond problem' occurs when a class inherits methods with the same signature from multiple interfaces. Java resolves this by requiring the implementing class to provide its implementation for the conflicting method."
What to Look For:Look for candidates who can explain how Java handles multiple inheritance and understand the challenges and solutions associated with the "diamond problem."
How to Answer:Candidates should list and describe the four main access modifiers in Java: public, private, protected, and default (no modifier). They should explain their visibility and accessibility rules within and outside the class hierarchy.
Sample Answer:"In Java, there are four access modifiers: public (accessible from anywhere), private (only within the same class), protected (within the same package and subclasses), and default (package-private, within the same package). They control the visibility and accessibility of class members."
What to Look For:Look for candidates who can clearly define access modifiers and provide examples of when and why each one is used.
How to Answer:Candidates should explain that checked exceptions are compile-time exceptions that must be handled using try-catch or declared with throws in the method signature. Unchecked exceptions, on the other hand, are runtime exceptions and do not require explicit handling.
Sample Answer:"Checked exceptions are exceptions that the compiler checks at compile-time, and you must either handle them using try-catch or declare them with throws in the method signature. Unchecked exceptions, also known as runtime exceptions, do not require explicit handling."
What to Look For:Look for candidates who can distinguish between checked and unchecked exceptions, understand their implications, and provide examples of each.
How to Answer:Candidates should explain that the finally block is used to ensure that a specific block of code is always executed, regardless of whether an exception is thrown or not. They should emphasize that it's commonly used for cleanup tasks like closing resources.
Sample Answer:"The finally block in Java ensures that a particular block of code is executed, whether an exception occurs or not. It's commonly used for tasks like closing files or releasing resources to guarantee proper cleanup."
What to Look For:Look for candidates who can articulate the purpose of the finally block and demonstrate an understanding of its role in exception handling and resource management.
How to Answer:Candidates should explain that synchronization in Java is used to control access to shared resources by multiple threads. They should mention that it prevents race conditions and ensures data consistency. Additionally, they should discuss synchronization mechanisms like synchronized methods and blocks.
Sample Answer:"Synchronization in Java is essential for controlling access to shared resources by multiple threads. It prevents race conditions and ensures data consistency. We achieve synchronization using synchronized methods or blocks to protect critical sections of code."
What to Look For:Look for candidates who can describe the importance of synchronization in multithreaded environments, provide examples of synchronization, and discuss potential issues like deadlock and how to avoid them.
How to Answer:Candidates should describe that wait() is used by a thread to release the lock and enter a waiting state until another thread calls notify() or notifyAll() to wake it up. notify() wakes up one waiting thread, while notifyAll() wakes up all waiting threads.
Sample Answer:"wait() is used by a thread to release the lock and enter a waiting state until another thread calls notify() or notifyAll(). notify() wakes up one waiting thread, while notifyAll() wakes up all waiting threads, allowing them to compete for the lock."
What to Look For:Look for candidates who can explain the purpose and differences between wait(), notify(), and notifyAll() methods and demonstrate an understanding of their use in thread synchronization.
How to Answer:Candidates should explain that the Java Memory Model (JMM) defines how threads interact with memory when reading and writing shared data. It's crucial for ensuring thread safety and preventing data inconsistencies in multithreaded programs.
Sample Answer:"The Java Memory Model (JMM) defines how threads interact with memory when reading and writing shared data. It's vital for ensuring thread safety and preventing data inconsistencies in multithreaded programs by specifying the order of memory operations."
What to Look For:Look for candidates who can articulate the significance of the Java Memory Model in multithreading and how it helps maintain data consistency.
How to Answer:Candidates should explain that Java supports automatic memory management through garbage collection. They should mention the types of garbage collectors in Java, such as the Serial, Parallel, and G1 collectors, and briefly describe their characteristics.
Sample Answer:"Java supports automatic memory management through garbage collection. There are various garbage collectors in Java, including the Serial, Parallel, and G1 collectors. Each has its own characteristics and is suitable for different scenarios."
What to Look For:Look for candidates who can discuss Java's garbage collection mechanisms, their advantages, and when to use specific types of garbage collectors.
How to Answer:Candidates should explain that the JVM is responsible for executing Java bytecode. They should describe the steps involved in the execution process, such as class loading, bytecode verification, and Just-In-Time (JIT) compilation.
Sample Answer:"The Java Virtual Machine (JVM) executes Java programs by loading classes, verifying bytecode, and then executing it. It uses Just-In-Time (JIT) compilation to convert bytecode into machine code for efficient execution."
What to Look For:Look for candidates who can provide a clear overview of the JVM's role in executing Java programs and explain key steps in the execution process.
How to Answer:Candidates should describe that the reflection API in Java allows inspection and manipulation of classes, methods, fields, and objects at runtime. They should mention use cases, such as dynamic code generation, serialization, and frameworks like Spring.
Sample Answer:"Java's reflection API enables runtime inspection and manipulation of classes, methods, fields, and objects. It's used in scenarios like dynamic code generation, serialization, and in frameworks like Spring for dependency injection."
What to Look For:Look for candidates who can explain the purpose of the reflection API, provide examples of its use cases, and discuss its advantages and potential drawbacks.
How to Answer:Candidates should describe that Java annotations are metadata annotations that provide additional information about classes, methods, fields, or other program elements. They should discuss practical applications, such as code documentation, code generation, and framework configuration.
Sample Answer:"Java annotations are metadata annotations used to provide additional information about program elements. They have practical applications in code documentation, code generation, and configuring frameworks like Hibernate or Spring."
What to Look For:Look for candidates who can explain the concept of Java annotations, offer examples of their use in real-world scenarios, and discuss their benefits in code organization and automation.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Now, let's dive into some advanced Java topics that will set you apart in those challenging interviews.
Understanding the internal workings of the Java Virtual Machine (JVM) is crucial to write efficient and optimized Java code.
Optimizing JVM performance involves configuring memory settings, garbage collection algorithms, and thread management. Profiling tools like VisualVM and JConsole can help identify bottlenecks.
Java automatically manages memory through garbage collection (GC). Understanding GC mechanisms is vital for efficient memory usage.
By adjusting GC settings, you can optimize memory usage and reduce application pauses. Common parameters include heap size, GC algorithm selection, and thread counts.
Java reflection allows you to inspect and manipulate classes, methods, fields, and constructors at runtime.
You can access class metadata, inspect annotations, and invoke methods dynamically. This is especially useful for frameworks like Spring.
Class<?> clazz = MyClass.class;
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
System.out.println("Method Name: " + method.getName());
}
Reflection is employed in frameworks, libraries, and testing frameworks like JUnit. It enables features like dependency injection and dynamic loading.
The Stream API introduced in Java 8 simplifies data processing and manipulation.
Streams provide a concise way to filter, transform, and aggregate data in collections.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.filter(n -> n % 2 == 0)
.mapToInt(Integer::intValue)
.sum();
Operations like filter, map, reduce, and collect make complex data operations more readable and efficient.
Lambda expressions introduce functional programming concepts to Java, making code more concise and expressive.
Lambda expressions are defined with a parameter list, an arrow ->, and a body.
// Traditional approach
Runnable runnable = new Runnable() {
public void run() {
System.out.println("Hello, World!");
}
};
// Lambda expression
Runnable lambdaRunnable = () -> System.out.println("Hello, World!");
Functional interfaces define a single abstract method and are crucial for using lambda expressions.
Functional programming (FP) principles emphasize immutability, pure functions, and higher-order functions.
FP can lead to more concise, predictable, and maintainable code. It's particularly useful in parallel and asynchronous programming.
Now, it's time to put your Java skills to the test with tricky coding exercises. These exercises will help you develop problem-solving abilities and apply your knowledge effectively.
Before jumping into coding challenges, let's establish a problem-solving approach that will serve as your foundation.
Now, let's explore some common Java coding challenges and their solutions. These examples will help you apply your problem-solving skills and Java knowledge effectively.
Problem: Write a Java program to find the maximum number in an array.
public class MaxInArray {
public static int findMax(int[] arr) {
if (arr == null || arr.length == 0) {
throw new IllegalArgumentException("Array is empty or null.");
}
int max = arr[0];
for (int num : arr) {
if (num > max) {
max = num;
}
}
return max;
}
public static void main(String[] args) {
int[] numbers = { 23, 45, 12, 67, 8, 56 };
int maxNumber = findMax(numbers);
System.out.println("The maximum number is: " + maxNumber);
}
}
In this example, we define a findMax method that iterates through the array and keeps track of the maximum value. The main method demonstrates how to use this method.
Problem: Write a Java program to reverse a string.
public class ReverseString {
public static String reverse(String input) {
if (input == null) {
throw new IllegalArgumentException("Input string is null.");
}
StringBuilder reversed = new StringBuilder();
for (int i = input.length() - 1; i >= 0; i--) {
reversed.append(input.charAt(i));
}
return reversed.toString();
}
public static void main(String[] args) {
String original = "Hello, World!";
String reversedString = reverse(original);
System.out.println("Reversed string: " + reversedString);
}
}
In this example, the reverse method takes an input string and builds a reversed version of it using a StringBuilder.
When solving coding exercises, it's essential to analyze the time and space complexity of your solutions.
By understanding these complexities, you can optimize your code and choose the most efficient solutions for different scenarios.
As you tackle more coding exercises and analyze their complexities, you'll become better equipped to solve challenging Java interview questions.
Congratulations on mastering the core Java concepts, exploring advanced topics, and honing your coding skills! Now, let's focus on essential tips and strategies to ensure you shine in those tricky Java interviews.
Interview success isn't just about what you know; it's also about how you present yourself and approach the interview.
Effective communication is vital during interviews to convey your ideas clearly and confidently.
Behavioral questions assess your past experiences and how you've handled specific situations.
Prepare for ethical and moral questions by considering your values and ethical principles. Be honest and transparent about how you would handle such situations.
Whiteboard coding can be daunting, but with practice and the right approach, you can excel.
Don't forget the importance of post-interview etiquette.
By following these tips and strategies, you'll not only demonstrate your technical prowess but also showcase your professionalism and interpersonal skills, setting you on the path to success in your tricky Java interviews.
Navigating tricky Java interview questions is a journey that demands preparation, practice, and a passion for learning. By mastering core Java concepts, embracing advanced topics, and honing your coding skills, you've equipped yourself with the tools needed to excel in challenging interviews.
Remember, interview success isn't just about what you know—it's about how you present yourself, communicate effectively, and handle pressure. With the right strategies and a commitment to continuous improvement, you're well-prepared to tackle any tricky Java interview that comes your way.