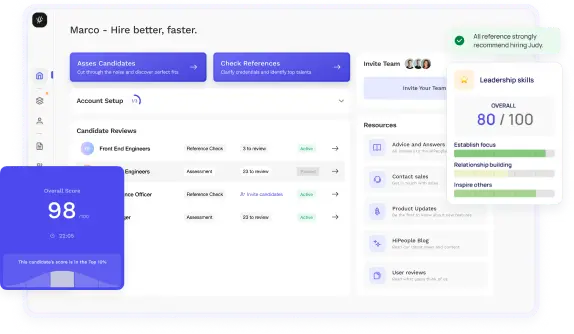
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to prove your expertise in Advanced Java? Prepare to tackle a diverse range of challenging questions and explore in-depth concepts in our comprehensive guide on Advanced Java Interview Questions. Whether you're a seasoned Java developer looking to advance your career or a job seeker aiming for a role that demands top-notch Java skills, this guide will equip you with the knowledge and confidence needed to excel in your next advanced Java interview.
Advanced Java interviews are a specialized category of job interviews conducted to assess the proficiency and expertise of candidates in advanced Java programming and related technologies. These interviews are typically geared towards positions that require in-depth knowledge of Java, Java EE, frameworks like Spring and Hibernate, and other advanced concepts.
Advanced Java interviews hold significant importance for both employers and candidates for the following reasons:
Advanced Java interviews serve as a critical evaluation point for both employers and candidates. They help identify top talent and ensure that the right individuals are entrusted with challenging and critical roles in the Java development landscape.
Preparing for Java interviews, especially for advanced roles, requires a combination of technical knowledge, behavioral readiness, and effective interview strategies. In this section, we will explore how to prepare for Java interviews comprehensively.
Java interviews often include questions related to core concepts and best practices. Here are some common areas to focus on:
Behavioral questions assess your soft skills, communication abilities, and teamwork. Be prepared to answer questions like:
Preparing for a technical interview requires focused effort. Here are some tips to excel:
Mock interviews are a valuable tool for interview preparation. Consider the following:
Remember, interview preparation is not just about knowing the answers; it's about demonstrating your problem-solving skills, your ability to communicate effectively, and your enthusiasm for the role. Stay confident, stay focused, and keep learning throughout your journey. Good luck!
How to Answer: Explain that abstract classes can have both abstract and concrete methods, while interfaces only have abstract methods. Discuss when to use each and provide examples.
Sample Answer: "An abstract class is a class that can have both abstract (unimplemented) and concrete (implemented) methods, while an interface is a collection of abstract methods only. Abstract classes are used when you want to provide a common base for multiple related classes, and interfaces are used to define a contract that multiple classes must adhere to."
What to Look For: Look for an understanding of when to use abstract classes and interfaces, and how they are implemented in Java.
How to Answer: Explain that == compares object references, while equals() compares the contents of objects. Provide examples to illustrate the differences.
Sample Answer: "The == operator in Java compares object references, checking if two references point to the same memory location. On the other hand, the equals() method compares the contents of objects to determine if they are equal. It's important to note that equals() can be overridden by classes to provide custom comparison logic."
What to Look For: Look for a clear explanation of the differences between == and equals(), along with the ability to provide examples.
How to Answer: Describe that the static keyword is used to create class-level variables and methods that can be accessed without creating an instance of the class. Provide examples of static variables and methods.
Sample Answer: "The static keyword in Java is used to create class-level variables and methods. Class-level variables are shared among all instances of the class, while class-level methods can be called without creating an instance of the class. For example, the Math class in Java has static methods like Math.sqrt() that can be called directly without creating a Math object."
What to Look For: Look for a clear understanding of how the static keyword is used and its purpose in Java.
How to Answer: Explain that checked exceptions are checked at compile-time and must be either caught or declared, while unchecked exceptions (runtime exceptions) are not checked at compile-time. Provide examples of each.
Sample Answer: "Checked exceptions are exceptions that are checked at compile-time, which means they must be either caught using a try-catch block or declared using the throws keyword in the method signature. Examples include IOException and SQLException. Unchecked exceptions, also known as runtime exceptions, are not checked at compile-time and include exceptions like NullPointerException and ArrayIndexOutOfBoundsException."
What to Look For: Look for a clear distinction between checked and unchecked exceptions, along with examples.
How to Answer: Describe that when an exception is thrown in a method, it can be propagated up the call stack until it is caught or the program terminates. Explain how this propagation can be controlled using try-catch blocks.
Sample Answer: "Exception propagation in Java occurs when an exception is thrown in a method and is then passed up the call stack to find an appropriate try-catch block that can handle it. If no suitable catch block is found, the program terminates. Developers can control exception propagation by using try-catch blocks to catch and handle exceptions at different levels of the call stack."
What to Look For: Look for an understanding of how exceptions propagate in Java and how to control it using try-catch blocks.
How to Answer: Explain that a thread is the smallest unit of execution within a process, while a process is a separate instance of a program with its own memory space. Discuss the advantages of using threads for concurrent programming.
Sample Answer: "A thread in Java is the smallest unit of execution within a process. It represents an independent path of execution and shares the same memory space as other threads in the same process. In contrast, a process is a separate instance of a program with its own memory space. Threads are used for concurrent programming in Java to take advantage of multiple cores and achieve parallelism."
What to Look For: Look for a clear explanation of threads, their relationship to processes, and the benefits of using threads for concurrent programming.
How to Answer: Describe the various thread states, such as NEW, RUNNABLE, BLOCKED, WAITING, TIMED_WAITING, and TERMINATED. Explain how a thread transitions between these states.
Sample Answer: "A Java thread can be in one of several states, including NEW, RUNNABLE, BLOCKED, WAITING, TIMED_WAITING, and TERMINATED. A thread transitions between these states based on its execution and synchronization. For example, a new thread is in the NEW state, and when it starts executing, it moves to the RUNNABLE state. If it's waiting for a lock, it can be in the BLOCKED state. Understanding these states and transitions is essential for effective multithreading."
What to Look For: Look for a clear explanation of thread states and transitions, demonstrating knowledge of multithreading concepts.
How to Answer: Explain that ArrayList is implemented as a dynamic array, while LinkedList is implemented as a doubly-linked list. Discuss the advantages and disadvantages of each.
Sample Answer: "In Java, ArrayList is implemented as a dynamic array, which means it provides fast random access but slower insertions and deletions. LinkedList, on the other hand, is implemented as a doubly-linked list, which makes it efficient for insertions and deletions but slower for random access. Choosing between them depends on the specific use case and the operations you need to perform."
What to Look For: Look for a clear distinction between ArrayList and LinkedList and an understanding of their trade-offs.
How to Answer: Describe that HashMap is a key-value pair data structure that allows fast retrieval of values based on their keys. Explain how it uses hash codes and buckets to store and retrieve elements.
Sample Answer: "In Java, HashMap is a data structure that stores key-value pairs. It uses a hashing mechanism to map keys to hash codes and then stores these key-value pairs in buckets based on their hash codes. This allows for fast retrieval of values based on their keys. HashMap is widely used for efficient data storage and retrieval in Java."
What to Look For: Look for a clear explanation of how HashMap works and its benefits in Java collections.
How to Answer: Explain that the Singleton design pattern ensures a class has only one instance and provides a global point of access to that instance. Discuss scenarios where it is appropriate to use the Singleton pattern.
Sample Answer: "The Singleton design pattern in Java ensures that a class has only one instance and provides a global point of access to that instance. It is used when you want to restrict the instantiation of a class to a single object, such as a database connection pool or a configuration manager. The Singleton pattern guarantees that there's only one instance of the class, and it can be accessed from anywhere in the application."
What to Look For: Look for a clear explanation of the Singleton pattern and an understanding of when it is suitable to use.
How to Answer: Describe that the Observer pattern defines a one-to-many relationship between objects, where one object (the subject) maintains a list of its dependents (observers) and notifies them of state changes. Provide an example of its usage.
Sample Answer: "The Observer design pattern in Java defines a one-to-many relationship between objects. One object, known as the subject, maintains a list of its dependents, known as observers, and notifies them of state changes. This pattern is often used in event handling systems and GUI frameworks. For example, in a weather application, multiple weather displays can be observers of a weather station subject. When the weather changes, all displays are notified and updated."
What to Look For: Look for a clear explanation of the Observer pattern and an example of its application.
How to Answer: Explain that Java provides classes like FileInputStream, FileOutputStream, BufferedReader, and BufferedWriter for file I/O operations. Describe how to read and write data to files using these classes.
Sample Answer: "Java provides classes like FileInputStream and FileOutputStream for reading and writing binary data to files, and classes like BufferedReader and BufferedWriter for reading and writing text data. To read from a file, you can create an FileInputStream and use it to read bytes from the file. For writing to a file, you can create a FileOutputStream and use it to write bytes to the file. Additionally, using BufferedReader and BufferedWriter makes it more efficient to read and write text data."
What to Look For: Look for an understanding of how Java handles file I/O operations and the use of relevant classes.
How to Answer: Describe that serialization is the process of converting an object into a byte stream, which can be saved to a file or sent over a network. Explain its importance in data persistence and communication.
Sample Answer: "Serialization in Java is the process of converting an object into a byte stream. This byte stream can be saved to a file, sent over a network, or stored in a database. Serialization is used for data persistence, allowing objects to be saved and retrieved later. It's also crucial for communication between distributed systems, as objects can be serialized and deserialized to pass data between them."
What to Look For: Look for an explanation of serialization and its significance in Java.
How to Answer: Explain that Spring Framework is a comprehensive and modular framework for building enterprise applications in Java. Describe its core modules and their purposes.
Sample Answer: "Spring Framework is a widely used framework in Java for building enterprise applications. Its core modules include the Spring Core Container, which provides dependency injection and bean lifecycle management, the Spring AOP module for aspect-oriented programming, the Spring Data Access/Integration module for database access, and the Spring Web module for building web applications. Spring simplifies application development by providing robust support for various aspects of application development."
What to Look For: Look for a description of Spring Framework and an overview of its core modules.
How to Answer: Describe that Hibernate is an object-relational mapping (ORM) framework that simplifies database interaction in Java applications. Explain how it maps Java objects to database tables.
Sample Answer: "Hibernate is an object-relational mapping (ORM) framework in Java that simplifies database interaction. It allows developers to map Java objects to database tables and provides a high-level, object-oriented approach to database operations. Hibernate handles the underlying SQL and database-specific details, making it easier to work with databases in Java applications."
What to Look For: Look for an explanation of Hibernate and its role in Java persistence.
These advanced Java interview questions cover a range of topics, including core Java concepts, exception handling, multithreading, Java collections, design patterns, Java I/O, and Java frameworks. Candidates who can provide clear and comprehensive answers to these questions demonstrate a strong understanding of advanced Java concepts and are well-prepared for advanced Java interviews.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Object-oriented programming (OOP) is the foundation of Java's design. It simplifies complex software development by modeling real-world entities as objects. Here's a deeper dive into OOP principles:
Encapsulation is about bundling data (attributes) and the methods (functions) that operate on the data into a single unit known as a class. It helps you achieve data hiding and restricts access to certain parts of your code.
Example:
class BankAccount {
private double balance;
public void deposit(double amount) {
// Deposit logic
}
public double getBalance() {
return balance;
}
}
Inheritance allows one class to inherit the properties and behaviors of another class. It promotes code reuse and hierarchical modeling.
Example:
class Vehicle {
void start() {
// Start logic
}
}
class Car extends Vehicle {
void drive() {
// Drive logic
}
}
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables you to write flexible and extensible code.
Example:
interface Shape {
void draw();
}
class Circle implements Shape {
void draw() {
// Draw circle
}
}
class Square implements Shape {
void draw() {
// Draw square
}
}
Abstraction is the process of simplifying complex systems by breaking them into smaller, more manageable parts. Abstract classes and interfaces provide a blueprint for classes to implement.
Example:
abstract class Shape {
abstract void draw();
}
Effective exception handling is vital for robust Java applications. It prevents unexpected errors from crashing your program and helps you handle failures gracefully. Let's delve deeper:
Exceptions in Java are categorized into two main types: checked and unchecked exceptions. Checked exceptions must be explicitly handled, while unchecked exceptions need not be.
A try-catch block is used to catch and handle exceptions gracefully. It consists of a try block where you place the code that might throw an exception and one or more catch blocks that specify how to handle specific exceptions.
Example:
try {
// Code that may throw an exception
} catch (ExceptionType e) {
// Handle the exception
}
The finally block is used to specify code that should always run, regardless of whether an exception occurs or not. It's typically used for resource cleanup.
Example:
try {
// Code that may throw an exception
} catch (ExceptionType e) {
// Handle the exception
} finally {
// Cleanup code
}
Multithreading is a powerful concept in Java that enables concurrent execution of tasks, improving performance and responsiveness. Let's explore it further:
Multithreading allows a program to run multiple threads concurrently, sharing the same resources like memory and CPU. It's especially useful for tasks that can be parallelized.
In Java, you can create threads by extending the Thread class or implementing the Runnable interface. Both approaches have their advantages.
Example (Using Runnable):
class MyRunnable implements Runnable {
public void run() {
// Code to be executed in the thread
}
}
When multiple threads access shared resources, synchronization is essential to prevent data corruption and race conditions. The synchronized keyword and locks help achieve synchronization.
Example:
class SharedResource {
private int count;
public synchronized void increment() {
count++;
}
}
Understanding and addressing issues like deadlock, race conditions, and thread interference is crucial for effective multithreading.
The Java Collections Framework provides a set of classes and interfaces for storing, manipulating, and processing data efficiently. Let's explore this further:
The framework includes core interfaces such as List, Set, and Map, each serving a specific purpose.
Java offers several implementations of these interfaces, each with its strengths and use cases.
You can iterate through collections using iterators or the enhanced for loop. Additionally, Java 8 introduced the Stream API, providing powerful tools for processing collections using functional programming.
Input and output operations are essential for interacting with files, networks, and external devices. Let's dive deeper into Java I/O:
Streams are the backbone of Java I/O. They represent the flow of data between a program and an I/O device. Java provides InputStreams and OutputStreams for byte-level operations and Readers and Writers for character-level operations.
Java provides classes like FileInputStream, FileOutputStream, FileReader, and FileWriter for reading and writing files. Proper exception handling is crucial when dealing with I/O operations.
Example (Reading from a File):
try (FileInputStream fis = new FileInputStream("example.txt")) {
int data;
while ((data = fis.read()) != -1) {
// Process the data
}
} catch (IOException e) {
// Handle the exception
}
Serialization allows objects to be converted into a byte stream, which can be saved to a file or transmitted over a network. It's essential for persistence and interprocess communication.
Example (Serialization):
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("object.ser"))) {
MyObject obj = new MyObject();
oos.writeObject(obj);
} catch (IOException e) {
// Handle the exception
}
Generics in Java allow you to create classes, interfaces, and methods that operate on parameterized types. Let's explore this concept in more detail:
A generic class is a class that can work with different types. You define a generic class by specifying a type parameter in angle brackets (<>).
Example (Generic Class):
class Box<T> {
private T value;
public void setValue(T value) {
this.value = value;
}
public T getValue() {
return value;
}
}
Wildcards allow you to write more flexible and reusable code when working with generic types. There are three types of wildcards: ?, ? extends T, and ? super T.
Example (Using Wildcards):
List<? extends Number> numbers = new ArrayList<>();
You can also create generic methods within non-generic classes. Generic methods allow you to parameterize methods independently of the class.
Example (Generic Method):
public <T> T getFirst(List<T> list) {
if (list.isEmpty()) {
return null;
}
return list.get(0);
}
Understanding and mastering these core Java concepts will significantly boost your confidence and success in advanced Java interviews. These fundamentals serve as the building blocks for more complex topics you'll encounter in your Java journey.
Java Enterprise Edition (Java EE) is a platform for building robust and scalable enterprise-level applications. In this section, we'll explore the key components and concepts of Java EE in detail.
Java EE is a set of specifications that extends the Java SE (Standard Edition) to provide a comprehensive platform for developing enterprise-level applications. It includes a variety of APIs and services tailored for enterprise development.
Servlets are Java classes that extend the functionality of web servers. They handle HTTP requests and responses, making them the backbone of web applications.
JSP allows you to embed Java code within HTML to generate dynamic web pages.
Enterprise JavaBeans (EJB) is a component architecture for building scalable and distributed enterprise applications.
Java Persistence API (JPA) is a specification for object-relational mapping (ORM) in Java applications.
JPA allows you to map Java objects to database tables and perform CRUD (Create, Read, Update, Delete) operations.
Java Messaging Service (JMS) is a Java-based messaging system for building asynchronous and reliable communication between components.
JavaServer Faces (JSF) is a framework for building user interfaces in Java web applications.
JSF provides a rich set of UI components that simplify building web interfaces, including buttons, forms, tables, and more.
JSF follows a well-defined lifecycle, including phases like initialization, validation, and rendering.
You can handle user interactions and events easily using JSF's event-driven model.
JSF supports navigation between pages and managing the flow of your web application.
The Spring Framework is a comprehensive framework for building Java applications. It offers a wide range of modules and features that simplify various aspects of application development.
Spring is an open-source framework that provides a lightweight and flexible way to develop Java applications. It simplifies the development of enterprise and web applications.
DI is at the core of Spring. It enables you to inject dependencies into classes rather than hardcoding them.
Spring's IoC container manages the creation and configuration of objects.
AOP allows you to separate cross-cutting concerns from your application's core logic.
Spring offers various modules for data access, web development, messaging, and more, such as Spring Data, Spring MVC, and Spring Security.
Spring Boot simplifies the development of standalone Java applications by providing sensible defaults and auto-configuration.
Spring Data simplifies data access and provides a consistent programming model for various data sources, including databases and NoSQL stores.
Spring Data Repositories offer a high-level abstraction for data access, reducing boilerplate code.
Spring Security is a powerful framework for securing Java applications.
Spring Integration enables seamless integration with various messaging systems, databases, and external services.
Spring Integration includes adapters for connecting to various systems, including JMS, FTP, and email servers.
By understanding and mastering the concepts and components of Java EE and the Spring Framework, you'll be well-prepared to build and maintain enterprise-grade Java applications with ease and efficiency. These technologies empower developers to create scalable, reliable, and feature-rich software solutions.
Hibernate is a powerful and widely-used object-relational mapping (ORM) framework in the Java ecosystem. It simplifies the interaction between Java applications and relational databases. In this section, we will explore various aspects of Hibernate in detail.
Hibernate is an ORM framework that enables Java applications to interact with relational databases using object-oriented principles. It abstracts the database interaction and provides a high-level, Java-centric API for managing database operations.
HQL is a powerful query language provided by Hibernate for querying data from the database using object-oriented syntax.
Caching is a crucial performance optimization technique in Hibernate. It reduces the number of database queries and enhances application responsiveness.
Hibernate offers several advanced features that cater to complex scenarios in application development:
Hibernate allows you to define and use custom data types, enabling mapping of complex or non-standard data structures.
Hibernate provides mechanisms for efficiently processing large datasets by using batch insert, update, and delete operations.
You can implement auditing and versioning of entities to track changes and maintain historical data.
Mastering advanced Java interview questions is your ticket to success in the competitive world of Java development. With a strong grasp of core concepts, frameworks, and best practices, you'll be well-prepared to impress potential employers and secure high-impact roles in the field. Remember to practice, stay up-to-date with industry trends, and approach each interview with confidence. Your advanced Java skills are your passport to a rewarding and fulfilling career in software development.
As you embark on your journey to conquer advanced Java interviews, remember that learning is an ongoing process. Stay curious, keep exploring new horizons, and never stop enhancing your skills. Your dedication to mastering advanced Java will not only open doors to exciting career opportunities but also enable you to make a meaningful impact in the world of technology.