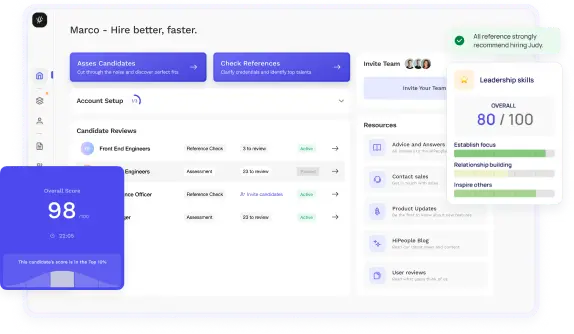
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to conquer the challenging world of C# interviews? Dive into our guide on Tricky C# Interview Questions, where we'll equip you with the knowledge and strategies needed to tackle even the toughest technical and behavioral questions with confidence.
Whether you're a seasoned developer looking to level up your skills or a newcomer preparing for your first C# interview, this guide has you covered from the fundamentals to the advanced topics and everything in between.
A Tricky C# Interview is a job interview for a C# developer or software engineer role that presents complex, thought-provoking questions and scenarios. These interviews aim to assess a candidate's deep understanding of C# programming concepts, problem-solving skills, and ability to apply knowledge in real-world situations. Tricky C# interviews often go beyond basic technical questions, delving into advanced topics and assessing a candidate's adaptability and creativity in finding solutions.
C# interviews hold immense significance for both candidates and employers:
Preparing for Tricky C# Interviews requires a systematic approach:
By dedicating time and effort to prepare for Tricky C# Interviews, you can boost your confidence, impress potential employers, and embark on a successful career journey in C# development.
First, we'll delve deeper into the core concepts of C# programming. Understanding these fundamentals is essential for acing your C# interview.
In C#, data types define the kind of data that a variable can hold. Here's a closer look at some commonly used data types:
int myInteger = 42;
string myString = "Hello, World!";
bool isTrue = true;
Understanding how to declare variables is crucial. Variables can be declared like this:
int myVariable; // Declaration
myVariable = 10; // Initialization
C# differentiates between value types and reference types.
int a = 10;
int b = a; // 'b' gets a copy of 'a'
b = 20; // 'a' remains 10
MyClass obj1 = new MyClass();
MyClass obj2 = obj1; // Both 'obj1' and 'obj2' reference the same object
obj2.MyProperty = 42; // Changes 'obj1' as well
Conditional statements in C# help you make decisions in your code. Here are some essential constructs:
if (condition)
{
// Code to execute if the condition is true
}
else
{
// Code to execute if the condition is false
}
switch (variable)
{
case value1:
// Code for value1
break;
case value2:
// Code for value2
break;
default:
// Code if none of the cases match
break;
}
Looping constructs are used to execute a block of code repeatedly.
for (int i = 0; i < 5; i++)
{
// Code to execute repeatedly
}
while (condition)
{
// Code to execute as long as the condition is true
}
do
{
// Code to execute at least once
} while (condition);
C# is an object-oriented language, and mastering OOP is crucial.
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
Person person = new Person();
person.Name = "John";
person.Age = 30;
public class Animal
{
public void Eat() { /* ... */ }
}
public class Dog : Animal
{
public void Bark() { /* ... */ }
}
Animal myAnimal = new Dog(); // Polymorphism
myAnimal.Eat(); // Accessing the Eat method of Dog
Exception handling is crucial for writing robust C# code.
try
{
// Code that might throw an exception
}
catch (Exception ex)
{
// Handle the exception
Console.WriteLine($"An error occurred: {ex.Message}");
}
Creating custom exceptions can be useful to handle specific error scenarios in your applications.
public class CustomException : Exception
{
public CustomException(string message) : base(message)
{
}
}
By mastering these core C# concepts, you'll build a strong foundation for more advanced topics. Next, we'll explore the world of multithreading and asynchronous programming in C#.
How to Answer: Begin by discussing the fundamental differences between string and StringBuilder, such as immutability and mutability. Highlight scenarios where one is more suitable than the other, and provide examples.
Sample Answer: "In C#, a string is immutable, meaning that once created, it cannot be modified. On the other hand, StringBuilder is mutable and allows for efficient string manipulation. For example, if you need to perform numerous concatenations or modifications to a string in a loop, using StringBuilder is more efficient due to reduced memory overhead."
What to Look For: Look for candidates who can clearly articulate the differences between string and StringBuilder and demonstrate a good understanding of when to use each in practical scenarios.
How to Answer: Explain that IEnumerable represents a sequence of objects that can be enumerated, while IEnumerator is used to iterate through the elements of an IEnumerable. Discuss how they work together and their roles in implementing custom collection classes.
Sample Answer: "IEnumerable is an interface that allows you to iterate over a collection of items, providing a single method GetEnumerator(), which returns an IEnumerator. IEnumerator is used to move through the collection one element at a time with methods like MoveNext() and Current. Together, they enable efficient iteration over collections."
What to Look For: Evaluate candidates based on their ability to explain the purpose and relationship of IEnumerable and IEnumerator, as well as their knowledge of implementing custom iterators.
How to Answer: Start by defining delegates as function pointers and discuss their usage in event handling and callbacks. Highlight the key differences between delegates and interfaces, such as the ability to hold multiple methods and their role in achieving loose coupling.
Sample Answer: "Delegates in C# are type-safe function pointers that allow you to reference methods and call them indirectly. They are often used in event handling scenarios. Unlike interfaces, delegates can hold references to multiple methods, making them powerful for implementing callbacks. Interfaces define a contract for classes to implement, enforcing a specific set of methods and properties."
What to Look For: Assess candidates' understanding of delegates, including their practical applications and distinctions from interfaces. Look for examples demonstrating the use of delegates in event-driven programming.
How to Answer: Define extension methods as static methods that allow you to add new functionality to existing types without modifying their source code. Provide a real-world scenario where extension methods enhance code readability and maintainability.
Sample Answer: "Extension methods in C# enable us to add methods to existing classes without altering their source code. For instance, in a scenario where we have a custom Person class but want to calculate a person's age based on their birthdate, we can create an extension method like CalculateAge() for the DateTime type. This enhances code readability and keeps the logic separate from the Person class."
What to Look For: Seek candidates who can clearly explain what extension methods are, their purpose, and how they can improve code organization and reusability.
How to Answer: Start by defining each concept. Discuss Dispose() as a method for explicitly releasing unmanaged resources, Finalize() as a method for cleanup during garbage collection, and Garbage Collection as the automatic process of releasing memory.
Sample Answer: "Dispose() is a method used to release unmanaged resources explicitly and implement the IDisposable interface. Finalize() is a method used for final cleanup during garbage collection, and it is not deterministic. Garbage Collection is an automatic process in C# that reclaims memory by identifying and releasing objects that are no longer reachable."
What to Look For: Evaluate candidates based on their understanding of resource management in C#, including the role of Dispose(), Finalize(), and the garbage collector. Look for an emphasis on proper resource cleanup.
How to Answer: Explain that the using statement is used for resource management and ensures that Dispose() is called on objects implementing IDisposable. Provide a practical example of using the using statement to manage resources.
Sample Answer: "The using statement in C# is used to automatically call the Dispose() method on objects that implement the IDisposable interface. For instance, when working with file streams, you can use using to ensure the stream is properly closed after use, like this:
using (FileStream fileStream = new FileStream("example.txt", FileMode.Open))
{
// Perform file operations here
} // Dispose() is automatically called here
What to Look For: Assess candidates' understanding of resource management and their ability to demonstrate the correct usage of the using statement for automatic disposal of resources.
How to Answer: Define a deadlock as a situation where two or more threads are unable to proceed due to circular dependencies on resources. Discuss strategies for deadlock prevention, such as using a predefined order for acquiring locks.
Sample Answer: "A deadlock in multithreading occurs when two or more threads are stuck, each waiting for a resource that the other thread holds. To prevent deadlocks, you can establish a predefined order for acquiring locks and ensure that all threads adhere to this order. Additionally, timeouts and resource allocation hierarchies can be used to avoid deadlocks."
What to Look For: Evaluate candidates' knowledge of multithreading challenges, including their ability to explain deadlocks and propose effective prevention strategies.
How to Answer: Start by defining both Task and Thread. Discuss the differences, including higher-level abstractions provided by Task, better resource utilization, and simplified exception handling.
Sample Answer: "Task is a higher-level abstraction in C# that represents an asynchronous operation. It offers better resource utilization and simplifies exception handling compared to directly working with threads. Thread, on the other hand, represents an OS-level thread and provides low-level control over threading."
What to Look For: Look for candidates who can distinguish between Task and Thread and understand the benefits of using Task for managing asynchronous operations.
How to Answer: Explain that the try block is used to enclose code that may throw exceptions, the catch block handles exceptions, and the finally block ensures cleanup code is executed, regardless of whether an exception is thrown.
Sample Answer: "In C#, the try block encloses code that may raise exceptions. If an exception occurs, control is transferred to the catch block, which handles the exception. The finally block is used for cleanup operations and is executed whether an exception occurs or not, ensuring resources are properly released."
What to Look For: Assess candidates' understanding of exception handling constructs and their ability to explain the roles of try, catch, and finally blocks.
How to Answer: Define checked exceptions as exceptions that must be explicitly caught or declared, and unchecked exceptions as exceptions that don't require explicit handling. Discuss scenarios where each type of exception is appropriate.
Sample Answer: "Checked exceptions in C# are exceptions that must be either caught using a try-catch block or declared in the method signature using the throws keyword. Unchecked exceptions, on the other hand, do not require explicit handling. Checked exceptions are typically used for recoverable errors, while unchecked exceptions are used for unrecoverable errors."
What to Look For: Look for candidates who can differentiate between checked and unchecked exceptions and provide examples of when to use each type.
How to Answer: Explain that LINQ (Language Integrated Query) is a feature in C# that allows for querying data from different data sources using a consistent syntax. Discuss how LINQ simplifies data manipulation and retrieval.
Sample Answer: "LINQ, or Language Integrated Query, is a powerful feature in C# that enables developers to write queries against various data sources, such as collections, databases, and XML, using a consistent and SQL-like syntax. It simplifies data querying by providing a unified way to retrieve, filter, and manipulate data, making code more expressive and readable."
What to Look For: Assess candidates' understanding of LINQ and their ability to explain its purpose in simplifying data querying tasks.
How to Answer: Define deferred execution as the concept in LINQ where query operations are not executed immediately but deferred until the results are actually enumerated. Explain the benefits of deferred execution, such as optimized query execution.
Sample Answer: "Deferred execution in LINQ means that query operations are not executed immediately when you define them but are deferred until the results are enumerated. This allows for optimized query execution, as LINQ providers can perform optimizations like lazy loading and query reordering to minimize data retrieval and processing until necessary."
What to Look For: Look for candidates who understand the concept of deferred execution in LINQ and can articulate its importance for query optimization.
How to Answer: Describe the Singleton design pattern as a pattern that ensures a class has only one instance and provides a global point of access to that instance. Provide a code example of a Singleton implementation in C#.
Sample Answer: "The Singleton design pattern in C# ensures that a class has only one instance throughout the application's lifetime and provides a global point of access to that instance. Here's a sample implementation of a Singleton in C#:
public class Singleton
{
private static Singleton instance;
private Singleton() { }
public static Singleton Instance
{
get
{
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
}
What to Look For: Assess candidates' understanding of the Singleton design pattern and their ability to provide a correct and concise implementation.
How to Answer: Define the Repository pattern as a structural pattern that separates data access logic from the rest of the application. Explain the benefits of using the Repository pattern for better code organization, testability, and maintainability.
Sample Answer: "The Repository pattern in C# is a structural pattern that abstracts and separates data access logic from the rest of the application. It provides a clean and standardized interface for interacting with data storage, such as databases or external services. By using the Repository pattern, developers can achieve better code organization, testability, and maintainability, as data access concerns are decoupled from the application's business logic."
What to Look For: Look for candidates who can explain the purpose and advantages of the Repository pattern in C# and its role in improving application architecture.
How to Answer: Discuss various performance optimization techniques, such as caching, asynchronous programming, code profiling, and database optimization. Highlight the importance of measuring and profiling to identify bottlenecks.
Sample Answer: "To improve the performance of a C# application, you can implement techniques like caching frequently used data, using asynchronous programming to handle I/O-bound operations efficiently, optimizing database queries and indexes, and employing code profiling tools to identify performance bottlenecks. It's crucial to measure and profile the application to pinpoint areas that need optimization."
What to Look For: Assess candidates' knowledge of performance optimization techniques in C# and their ability to suggest appropriate strategies for enhancing application performance.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Now, let's dive into the advanced C# topics that can set you apart in interviews and real-world coding scenarios.
Delegates in C# allow you to treat methods as first-class citizens, enabling powerful event handling and callback mechanisms.
public delegate void MyDelegate(string message);
public class Example
{
public void DisplayMessage(string message)
{
Console.WriteLine(message);
}
}
Events are a way to encapsulate delegates, providing a mechanism for one class to notify others when something of interest happens.
public class Publisher
{
public event MyDelegate OnMessagePublished;
public void PublishMessage(string message)
{
OnMessagePublished?.Invoke(message);
}
}
Generics enable you to write flexible and reusable code by allowing the creation of classes, structures, methods, and interfaces with type parameters.
public class MyGenericClass<T>
{
public T Value { get; set; }
}
var intContainer = new MyGenericClass<int>();
intContainer.Value = 42;
var stringContainer = new MyGenericClass<string>();
stringContainer.Value = "Hello, Generics!";
LINQ is a powerful feature in C# that allows you to query and manipulate data from various sources in a declarative and type-safe manner.
var numbers = new List<int> { 1, 2, 3, 4, 5 };
var evenNumbers = numbers.Where(n => n % 2 == 0);
Reflection allows you to inspect and manipulate types, objects, and assemblies at runtime. It's commonly used in frameworks like ASP.NET and Entity Framework.
Type type = typeof(MyClass);
MethodInfo methodInfo = type.GetMethod("MyMethod");
Attributes are used to add metadata to your code. They play a significant role in aspects like serialization, validation, and custom behaviors.
[Serializable]
public class MySerializableClass
{
// ...
}
DI is a design pattern that promotes loose coupling between classes by injecting their dependencies instead of creating them internally.
public class OrderService
{
private readonly IOrderRepository _repository;
public OrderService(IOrderRepository repository)
{
_repository = repository;
}
public void PlaceOrder(Order order)
{
_repository.Save(order);
}
}
Inversion of Control (IoC) containers like Unity, Autofac, and Ninject help manage and resolve dependencies in your application.
var container = new ContainerBuilder();
container.RegisterType<OrderRepository>().As<IOrderRepository>();
container.RegisterType<OrderService>();
var serviceProvider = container.Build();
var orderService = serviceProvider.Resolve<OrderService>();
Mastering these advanced C# topics will not only impress interviewers but also equip you with powerful tools for writing efficient and maintainable code.
To become a proficient C# developer and excel in your interviews, it's essential to follow industry best practices. These guidelines ensure your code is clean, maintainable, and performs well.
Consistency in code style and naming conventions is crucial for readability and collaboration. Here are some key aspects to consider:
public class MyClassName
{
public void MyMethodName() { /* ... */ }
public string MyPropertyName { get; set; }
}
int myVariable = 42;
/// <summary>
/// This is a sample class.
/// </summary>
public class SampleClass
{
/// <summary>
/// This is a sample method.
/// </summary>
/// <param name="value">The input value.</param>
/// <returns>The result.</returns>
public int SampleMethod(int value)
{
// Implementation details
return value * 2;
}
}
Robust error handling is essential for preventing unexpected crashes and ensuring a positive user experience.
Efficient code execution is critical for a smooth-running application. Consider these optimization techniques:
Effective memory management ensures your application uses system resources efficiently.
By adhering to these best practices, you'll not only write code that's easier to maintain and collaborate on but also code that performs optimally and is more robust in handling errors and resource management.
Preparing for a C# interview involves not only technical knowledge but also strategies for effective communication, managing stress, and following up after the interview.
Remember that interviews are not just about proving your technical prowess but also demonstrating your problem-solving abilities, communication skills, and cultural fit within the organization. With thorough preparation and a positive mindset, you can increase your chances of acing those tricky C# interviews.
Mastering Tricky C# Interview Questions is within your grasp. By understanding the core concepts, practicing coding challenges, and honing your communication skills, you can confidently face interviews. Remember to stay calm, seek feedback, and never stop learning. Interviews may be challenging, but with the right preparation and mindset, you can excel and pave the way to a rewarding career in C# development.
So, take a deep breath, stay positive, and embrace the opportunities that come your way. Whether you're aspiring to land your first job or aiming for that dream position, your dedication to C# expertise will be your key to success. Keep coding, keep learning, and keep reaching for your goals.