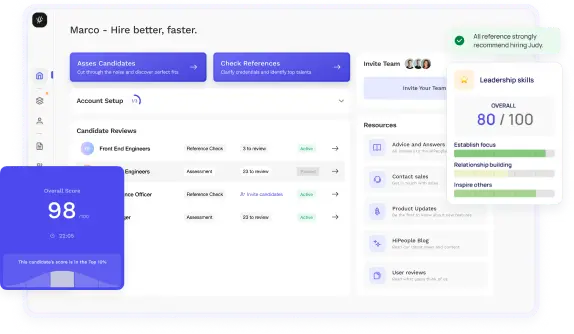
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to conquer Java coding interviews and showcase your programming prowess? Prepare to dive deep into the world of Java Coding Interview Questions, where we'll unravel the secrets to mastering these challenging assessments. Whether you're a seasoned Java developer looking to fine-tune your skills or a job seeker aspiring to land that dream tech role, this guide is your ticket to success. Let's demystify the interview process, explore key concepts, and equip you with the knowledge and confidence to ace your Java coding interviews.
A Java coding interview is a crucial step in the hiring process for software developers, engineers, and programmers. It serves as an assessment of a candidate's technical skills, problem-solving abilities, and coding proficiency using the Java programming language. These interviews are designed to evaluate how well candidates can apply their Java knowledge to real-world coding challenges and problems.
Java holds a prominent place in coding interviews for several reasons:
A typical Java coding interview follows a structured process that assesses various aspects of a candidate's abilities. While the specific format may vary, here's a general overview of the interview process:
Java coding interviews come in various formats and types, depending on the employer's preferences and the role's requirements. Common interview formats and types include:
Understanding the Java coding interview process, its importance, and the various formats will help you prepare effectively and increase your chances of success.
Let's dive deeper into the core Java concepts that are crucial for your success in coding interviews. We'll explore the nuances of data types, variables, operators, control flow structures, and essential object-oriented programming (OOP) principles.
In Java, data types define the kind of values a variable can hold. Understanding them is essential for efficient memory usage and error-free code.
int age = 30;
String name = "John";
Operators are the tools you use to manipulate data in Java. Understanding how they work is key to writing efficient code.
Control flow constructs determine the order in which your code executes. They are vital for decision-making and looping.
if (condition) {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
Loops (while, for, do-while): Loops are used to repeat code execution. They include:
Arrays and Strings are fundamental data structures in Java, and they require special attention due to their ubiquity.
Java is an object-oriented language, and mastering OOP concepts is crucial for interviews.
By mastering these Java fundamentals, you'll build a strong foundation for tackling more complex coding challenges in your interviews. Now, let's delve into Java collections and data structures, another critical area for your success.
To excel in Java coding interviews, it's essential to have a strong grasp of various data structures and collections. In this section, we'll explore the most commonly used data structures and how they can be leveraged in Java.
Java provides a versatile array of collection classes, each designed to serve specific needs. Understanding when and how to use ArrayList, LinkedList, and Vector can significantly enhance your coding skills.
Example Usage:
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
int firstNumber = numbers.get(0);
Example Usage:
LinkedList<String> names = new LinkedList<>();
names.add("Alice");
names.add("Bob");
String firstName = names.getFirst();
Example Usage:
Vector<String> vector = new Vector<>();
vector.add("Java");
vector.add("is");
vector.add("powerful");
Working with key-value pairs is a common requirement in Java programming. Understanding the differences between HashMap, HashSet, and HashTable is crucial for efficient data management.
Example Usage:
HashMap<String, Integer> ageMap = new HashMap<>();
ageMap.put("Alice", 25);
ageMap.put("Bob", 30);
int aliceAge = ageMap.get("Alice");
Example Usage:
HashSet<String> uniqueNames = new HashSet<>();
uniqueNames.add("Alice");
uniqueNames.add("Bob");
Stacks and queues are fundamental data structures that are widely used in various algorithms and applications.
Example Usage:
Stack<Integer> stack = new Stack<>();
stack.push(1);
stack.push(2);
int topElement = stack.pop();
Example Usage:
Queue<String> queue = new LinkedList<>();
queue.add("Task 1");
queue.add("Task 2");
String nextTask = queue.poll();
Trees and graphs are complex data structures used for various applications, from hierarchical data representation to solving intricate problems.
Example Usage:
class TreeNode {
int data;
TreeNode left;
TreeNode right;
}
By mastering these Java collections and data structures, you'll be better equipped to solve complex problems efficiently during coding interviews. These tools will empower you to tackle a wide range of algorithmic challenges with confidence.
How to Answer:Candidates should explain that String is immutable, while StringBuilder and StringBuffer are mutable. StringBuilder is not thread-safe, whereas StringBuffer is thread-safe due to synchronization.
Sample Answer:"In Java, String is an immutable class, meaning its content cannot be changed after creation. StringBuilder and StringBuffer are mutable and can be modified. The main difference between StringBuilder and StringBuffer is that StringBuilder is not thread-safe, making it faster in single-threaded environments, while StringBuffer is thread-safe due to synchronization. Use StringBuilder for better performance in non-threaded scenarios and StringBuffer when thread safety is required."
What to Look For:Look for candidates who can clearly explain the differences and understand the implications of immutability and thread safety in the context of these classes.
How to Answer:Candidates should explain that == compares object references, while .equals() compares object content. == is used to check if two references point to the same memory location, while .equals() is used to compare the values of objects.
Sample Answer:"In Java, == is used to compare object references, determining if two variables point to the same memory location. On the other hand, .equals() is a method that compares the content or values of objects. You should use == when you want to check if two references are the same, and .equals() when you want to compare the content of objects."
What to Look For:Ensure candidates understand the fundamental difference between reference comparison (==) and content comparison (.equals()) and can provide clear examples of when to use each.
How to Answer:Candidates should explain that an abstract class can have both abstract and concrete methods, while an interface only contains abstract methods. A class can implement multiple interfaces but inherit from only one abstract class.
Sample Answer:"In Java, an abstract class is a class that can have both abstract and concrete methods. It provides a partial implementation and can be extended by other classes using the extends keyword. An interface, on the other hand, is a contract that defines a set of abstract methods but does not provide any implementation. A class can implement multiple interfaces using the implements keyword, but it can inherit from only one abstract class using extends."
What to Look For:Look for candidates who can clearly articulate the differences between abstract classes and interfaces and understand when to use each in software design.
How to Answer:Candidates should explain that method overloading is when multiple methods in the same class have the same name but different parameters, while method overriding is when a subclass provides a specific implementation for a method defined in its superclass.
Sample Answer:"Method overloading in Java is the practice of defining multiple methods in the same class with the same name but different parameter lists. The compiler distinguishes these methods based on the number or types of parameters. Method overriding, on the other hand, occurs when a subclass provides a specific implementation for a method that is already defined in its superclass. This allows polymorphic behavior, where the appropriate method is called based on the actual object's type at runtime."
What to Look For:Ensure candidates can differentiate between method overloading and method overriding and understand their use cases in object-oriented programming.
How to Answer:Candidates should explain that checked exceptions are checked at compile time and must be either caught or declared, whereas unchecked exceptions (runtime exceptions) are not checked at compile time and can be handled optionally.
Sample Answer:"In Java, checked exceptions are exceptions that are checked by the compiler at compile time. They must be either caught using a try-catch block or declared in the method signature using the throws keyword. Unchecked exceptions, also known as runtime exceptions, are not checked by the compiler at compile time and can occur at runtime. Handling unchecked exceptions is optional, and they do not need to be declared."
What to Look For:Look for candidates who can explain the distinction between checked and unchecked exceptions and understand the implications for error handling.
How to Answer:Candidates should explain that the try-with-resources statement is used for automatic resource management in Java. It ensures that resources (such as file streams) are closed properly after use, reducing the risk of resource leaks and improving code readability.
Sample Answer:"The try-with-resources statement in Java is used to automatically manage resources that need to be closed after use, such as file streams, database connections, or sockets. It ensures that these resources are closed properly, even if an exception is thrown within the try block. You declare resources within the parentheses of the try statement, and Java takes care of closing them when the try block exits. It's important because it reduces the risk of resource leaks and makes code more readable by eliminating the need for explicit finally blocks to close resources."
What to Look For:Ensure candidates understand the purpose and benefits of the try-with-resources statement and can provide examples of its usage.
How to Answer:Candidates should explain that arrays have a fixed size and can hold elements of the same type, while ArrayLists are dynamic in size and can hold elements of any type. ArrayLists provide built-in methods for manipulation.
Sample Answer:"An array in Java is a fixed-size data structure that can hold elements of the same type. Once you define the size of an array, it cannot be changed. In contrast, an ArrayList is part of the Java Collections Framework and provides a dynamic, resizable array-like structure. ArrayLists can hold elements of any type and automatically resize themselves as elements are added or removed. They also offer built-in methods for easy manipulation, such as adding, removing, and searching for elements."
What to Look For:Look for candidates who can clearly describe the differences between arrays and ArrayLists and understand when to use each data structure.
How to Answer:Candidates should explain that a HashMap is a data structure that stores key-value pairs. The put() method is used to add or update values associated with keys, while the get() method is used to retrieve values based on keys.
Sample Answer:"A HashMap in Java is a data structure that stores key-value pairs. It uses a hash function to map keys to indices in an underlying array. The put() method is used to add or update values associated with keys. When you call put(key, value), the HashMap calculates the hash code of the key, determines the index in the array, and stores the value at that index. The get() method is used to retrieve values based on keys. It calculates the hash code of the key, finds the corresponding index, and returns the associated value if it exists. If multiple keys have the same hash code (collision), the HashMap uses a linked list or other data structure to handle collisions."
What to Look For:Ensure candidates understand the basic principles of how HashMaps work and can explain the roles of the get() and put() methods in HashMap operations.
How to Answer:Candidates should explain that a thread is a lightweight unit of execution within a process, while a process is a standalone program with its own memory space. Threads within the same process share memory and resources.
Sample Answer:"In Java, a thread is a lightweight unit of execution that runs within a process. Threads within the same process share the same memory space and resources, making them more efficient for tasks that require parallelism. A process, on the other hand, is a standalone program with its own memory space. Processes do not share memory with each other and are more heavyweight in terms of resource usage. Threads are suitable for tasks that require concurrency within a single program, while processes are typically used for separate, independent programs."
What to Look For:Look for candidates who can provide a clear distinction between threads and processes and understand the advantages of using threads for concurrency.
How to Answer:Candidates should explain that synchronization is the coordination of multiple threads to prevent race conditions and ensure data consistency. The synchronized keyword is used to create synchronized blocks or methods to achieve thread safety.
Sample Answer:"Synchronization in Java is the process of coordinating multiple threads to ensure data consistency and prevent race conditions. When multiple threads access shared resources concurrently, it can lead to unexpected behavior. The synchronized keyword is used to create synchronized blocks or methods that allow only one thread to access the synchronized code at a time. This ensures that the data is accessed and modified in a controlled manner, avoiding conflicts and maintaining thread safety."
What to Look For:Ensure candidates can explain the purpose of synchronization and demonstrate an understanding of how the synchronized keyword is used to achieve thread safety.
How to Answer:Candidates should explain that the JVM is a runtime environment for executing Java applications and is responsible for memory allocation and garbage collection. It manages memory by dividing it into different areas, such as the heap and the stack.
Sample Answer:"The Java Virtual Machine (JVM) is a runtime environment that executes Java applications. One of its key responsibilities is memory management. The JVM divides memory into different areas, including the heap, the stack, and the method area. The heap is where objects are allocated and deallocated during the program's execution. The stack is used for method call frames and stores local variables. The method area holds class metadata and bytecode. The JVM also employs a garbage collector to reclaim memory occupied by objects that are no longer reachable, freeing up resources for new objects. Understanding how the JVM manages memory is crucial for writing efficient and memory-safe Java applications."
What to Look For:Ensure candidates can describe the role of the JVM in memory management and have knowledge of memory areas and garbage collection in Java.
How to Answer:Candidates should explain that garbage collection is the automatic process of reclaiming memory occupied by objects that are no longer reachable or in use. It works by identifying and freeing up memory used by unreachable objects.
Sample Answer:"Garbage collection in Java is the automatic process of identifying and reclaiming memory occupied by objects that are no longer reachable or in use by the program. The Java Virtual Machine (JVM) employs various garbage collection algorithms to perform this task. The most common approach is the 'reachability analysis,' which starts from the root objects (e.g., references from main methods or currently executing threads) and traces through references to determine which objects are reachable. Objects that cannot be reached by any path from the root are considered 'garbage' and are subject to collection. The JVM then reclaims the memory used by these garbage objects, making it available for new allocations."
What to Look For:Look for candidates who can explain the concept of garbage collection in Java and understand the basic mechanisms and algorithms used by the JVM for memory management.
How to Answer:Candidates should explain that the Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. Implementing the Singleton pattern typically involves a private constructor and a static method to retrieve the single instance.
Sample Answer:"The Singleton design pattern in Java is used to ensure that a class has only one instance and provides a global point of access to that instance. This pattern is useful when you want to control access to a single shared resource, such as a database connection or a configuration manager. To implement the Singleton pattern, you typically make the constructor of the class private to prevent direct instantiation and provide a static method to retrieve the single instance. Here's an example implementation:
public class Singleton {
private static Singleton instance;
private Singleton() {
// Private constructor to prevent instantiation.
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
This ensures that there is only one instance of the Singleton class, and you can access it using Singleton.getInstance()."
What to Look For:Ensure candidates can explain the Singleton design pattern and provide a clear example of its implementation, including the use of a private constructor and a static method for instance retrieval.
How to Answer:Candidates should explain that the Factory Method pattern defines an interface for creating objects but allows subclasses to alter the type of objects that will be created. It is used when a class cannot anticipate the class of objects it needs to create.
Sample Answer:"The Factory Method design pattern in Java is a creational pattern that defines an interface for creating objects but allows subclasses to alter the type of objects that will be created. It provides a way to delegate the responsibility of instantiating objects to subclasses. This pattern is useful when a class cannot anticipate the class of objects it needs to create or when a class wants to delegate the responsibility of object creation to its subclasses. By using Factory Methods, you can achieve greater flexibility and decouple the client code from specific object creation details."
What to Look For:Look for candidates who can explain the purpose of the Factory Method pattern and its use cases in Java design. They should understand how it promotes flexibility and extensibility in object creation.
How to Answer:Candidates should explain that unit testing is the practice of testing individual units or components of a software system in isolation to ensure they work correctly. It is important for detecting and preventing defects early in the development process, improving code quality, and providing a safety net for future changes.
Sample Answer:"Unit testing in Java is the practice of testing individual units or components of a software system in isolation from the rest of the application. It involves writing test cases that exercise specific functions, methods, or classes to ensure they work as expected. Unit testing is crucial in software development for several reasons. First, it helps detect and prevent defects early in the development process, which reduces the cost and effort required to fix issues later. Second, it improves code quality by promoting modular and well-structured code that is easier to maintain. Third, it provides a safety net for future code changes, allowing developers to make enhancements or refactor code with confidence that existing functionality remains intact. Overall, unit testing contributes to the reliability and maintainability of software."
What to Look For:Look for candidates who can explain the importance of unit testing in software development and understand its benefits in terms of defect prevention, code quality, and maintainability. They should also be familiar with testing frameworks and tools commonly used for unit testing in Java.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Now, let's dive deep into the world of algorithmic problem-solving in Java. Coding interviews often present you with complex problems that require efficient solutions. To ace these challenges, you'll need a strong grasp of various algorithms and problem-solving techniques.
Recursion is a powerful technique where a function calls itself to solve a problem. It's particularly essential for solving problems involving repetitive structures, such as trees and graphs. Backtracking, on the other hand, is a strategy to find solutions incrementally and backtrack when a solution is not valid. Together, they form the foundation of many algorithmic solutions.
Sorting is a fundamental operation in computer science, and knowing different sorting algorithms and their efficiency is crucial for optimizing your code.
Searching is another fundamental operation, and different searching algorithms have various use cases and efficiency considerations.
Dynamic programming is a problem-solving technique that involves breaking down a problem into smaller overlapping subproblems and solving each subproblem only once to optimize overall efficiency.
Graphs are versatile data structures used to model various real-world scenarios. Understanding graph algorithms is crucial for solving complex network-related problems and optimizing data structures.
By mastering these algorithmic techniques and problem-solving strategies, you'll be well-prepared to tackle the most challenging coding interview questions with confidence and efficiency. Now, let's move on to Java coding best practices.
Now that you have a solid grasp of Java fundamentals and algorithmic problem-solving, it's time to focus on preparing for the coding interview itself. In this section, we'll explore various aspects of interview preparation, from building a portfolio of projects to fine-tuning your technical and behavioral skills.
Creating a portfolio of projects not only showcases your practical skills but also provides valuable talking points during interviews. Here's how you can approach it:
Practice makes perfect, and mock interviews are an invaluable tool for honing your skills. Here's how to make the most of them:
Coding interviews aren't just about technical skills; behavioral aspects play a significant role. Be ready to demonstrate your soft skills and professionalism:
Technical interview preparation goes beyond coding. It involves understanding the interview format, company culture, and expectations:
By dedicating time and effort to your interview preparation, you'll not only boost your confidence but also increase your chances of impressing potential employers during your Java coding interviews. In the next section, we'll explore Java coding best practices to ensure your code is not only functional but also maintainable and efficient.
To excel in Java coding interviews, it's not just about solving problems; it's about writing clean, efficient, and maintainable code. Employers value candidates who adhere to best practices. Here are essential Java coding best practices to keep in mind:
Interview day can be nerve-wracking, but proper preparation and effective strategies can help you perform at your best. Here are some tips and strategies to ace your Java coding interviews:
By incorporating these best practices and strategies, you'll be well-prepared for interview day. Remember that practice and preparation are key to your success.
Mastering Java coding interview questions is a journey that demands dedication and continuous learning. By thoroughly understanding Java fundamentals, algorithmic problem-solving, best practices, and interview strategies, you've equipped yourself with the tools to shine in the competitive world of tech interviews.
Remember, success in coding interviews isn't solely about finding the right answers; it's about demonstrating your problem-solving approach, clear communication, and adaptability. Keep practicing, seek feedback, and stay resilient. With the knowledge and skills gained from this guide, you're well-prepared to tackle Java coding interviews and embark on a rewarding career in the dynamic realm of software development.