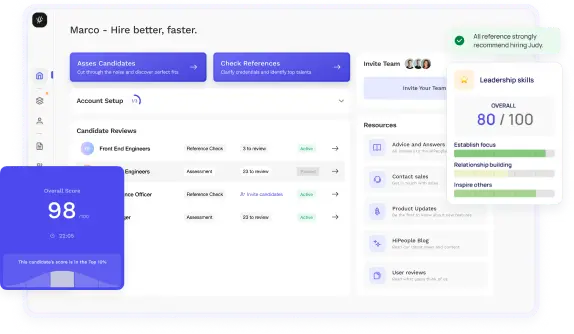
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Welcome to the ultimate guide on acing your Unity game development interviews! Whether you're a seasoned developer or just starting your journey in game development, this guide will equip you with the knowledge and skills to tackle even the most challenging Unity interview questions. Get ready to impress potential employers with your expertise in Unity's core concepts, scripting, performance optimization, and much more.
Unity is a popular and powerful cross-platform game development engine used by developers worldwide. It provides a user-friendly interface and a robust set of tools to create stunning games for various platforms, including PC, consoles, mobile, and even AR/VR devices. With a strong community and extensive documentation, Unity has become a go-to choice for both indie developers and large studios.
Unity's versatility, ease of use, and extensive asset store make it an essential tool for game development. Its real-time development capabilities enable developers to iterate quickly and efficiently, leading to faster game development cycles. Understanding Unity's role in the game development ecosystem is crucial for any aspiring game developer.
Before diving into interview-specific topics, it's essential to grasp Unity's fundamental concepts:
Interviews can take various formats, including technical assessments, coding challenges, and behavioral questions. Being aware of the format will help you prepare effectively. Here's what you might encounter:
Your portfolio is your opportunity to showcase your skills and creativity. Make sure to include:
Revisit essential game development concepts to strengthen your foundation:
Sharpen your problem-solving abilities with coding exercises and challenges:
In Unity, GameObjects serve as the building blocks of the scene, representing anything from characters and objects to lights and cameras. Understanding Transforms is essential as they define an object's position, rotation, and scale in 3D space. Unity provides several ways to manipulate GameObjects and Transforms, such as through code or the editor interface.
Unity Components enhance GameObject functionality. Here are some essential components:
C# is the primary scripting language used in Unity. Familiarize yourself with the following C# concepts:
MonoBehaviour is the base class for scripts in Unity. Unity's execution lifecycle involves specific event functions that get called at different stages:
Mastering common coding patterns can improve code organization and readability:
Unity's built-in physics engine facilitates realistic interactions between objects. The physics system considers factors like gravity, friction, and collision response.
Animations bring life to characters and objects in the game. The Animation system in Unity includes:
Character Controllers handle player movement and input. Some popular approaches include:
The Unity UI system is used to create interactive user interfaces. Key components include:
Ensure a smooth user experience by handling user input effectively:
Unity offers various networking options to create multiplayer experiences:
In multiplayer games, ensuring smooth synchronization is vital:
Optimizing game performance is crucial for delivering a smooth and enjoyable experience to players. Some optimization techniques include:
Profiling allows you to identify performance bottlenecks. Unity provides built-in tools to help you profile and debug your game:
To prevent memory leaks and optimize memory usage, follow these best practices:
ScriptableObjects are a powerful way to store data in Unity:
Custom shaders allow you to create unique visual effects:
Procedural generation adds variety and replayability to your games:
Expand your game's capabilities by incorporating third-party assets and plugins:
Explore Unity's capabilities in AR and VR development:
Design patterns help solve common game development challenges:
Create engaging game levels with well-designed flow and pacing:
Implementing compelling game mechanics is essential for captivating gameplay:
Be ready to discuss obstacles you encountered during previous game development endeavors and how you overcame them. Discuss topics like performance bottlenecks, bug fixing, and handling tight deadlines.
Game development is often a team effort, and effective communication is vital. Be prepared to share experiences of working with teams, resolving conflicts, and collaborating on game projects.
Games often involve making design and development decisions that impact the player experience. Discuss how you approach decision-making and consider factors like player feedback and design goals.
Optimize your game for various platforms to reach a broader audience:
Mobile game development has its unique challenges:
The Unity Asset Store offers a wealth of assets, scripts, and plugins to speed up development:
Version control is essential for collaborative game development:
How to Answer:Explain that GameObjects are the fundamental building blocks in Unity, representing any entity in the scene. Emphasize that they are containers for Components, such as scripts, colliders, and rigidbodies. Describe how GameObjects enable developers to create and manipulate entities, ranging from characters and objects to lights and cameras.
Sample Answer:"In Unity, GameObjects are essential elements that serve as the backbone of any scene. They act as containers for various Components, which define their behavior and appearance. For example, a GameObject representing a player character could have a script for movement, a collider for detecting collisions, and a mesh renderer for displaying its 3D model. GameObjects allow us to create and control entities in the game world, making them a crucial aspect of Unity game development."
What to Look For:Look for candidates who demonstrate a clear understanding of GameObjects and how they function as containers for Components. Strong candidates should also be able to articulate the importance of GameObjects in organizing and managing game entities.
How to Answer:Explain that scripts are written in C# and control the behavior and interactions of GameObjects. Mention how scripts are attached to GameObjects as Components. Discuss common coding patterns like the Singleton pattern for ensuring a single instance of a class or Object Pooling for reusing objects to optimize performance.
Sample Answer:"In Unity, scripts are written in C# and serve as the brains of GameObjects. They allow us to define the logic and behavior of various game elements. We attach scripts to GameObjects as Components to execute code during specific events, like Start, Update, and OnCollisionEnter. When it comes to coding patterns, I often use the Singleton pattern to ensure only one instance of critical classes, and Object Pooling to reuse objects and reduce instantiation overhead, resulting in better performance."
What to Look For:Look for candidates who demonstrate a good understanding of scripting in Unity and can explain common coding patterns they use. Strong candidates should be able to provide clear examples of how they've applied these patterns in their projects to improve code organization and performance.
How to Answer:Explain that Unity's built-in physics engine handles realistic interactions between objects. Discuss how Rigidbody components enable GameObjects to interact with physics forces. Describe collision detection modes (e.g., Continuous, Discrete) and how collision layers and masks manage object interactions.
Sample Answer:"Physics and collision detection are vital aspects of Unity game development. Unity's physics engine handles object interactions, taking into account gravity, friction, and collision responses. To enable physics interactions, we attach Rigidbody components to GameObjects. Additionally, Unity offers various collision detection modes, like Continuous and Discrete, to handle different collision scenarios. By utilizing collision layers and masks, we can control which GameObjects can collide with each other, ensuring efficient and accurate collision detection."
What to Look For:Candidates should demonstrate a solid understanding of Unity's physics system and how to implement collision detection effectively. Look for examples of how they've handled physics-related challenges in their projects.
How to Answer:Candidates should discuss techniques such as object pooling, level of detail (LOD), and texture atlasing. They should explain how these techniques improve performance by reducing draw calls and memory usage.
Sample Answer:"Optimizing performance in Unity is crucial to ensure a smooth and enjoyable gaming experience. One technique I often use is object pooling, which reuses objects instead of instantiating and destroying them frequently, significantly reducing performance overhead. Additionally, I implement LOD to switch between different levels of detail for distant objects, conserving processing power. Texture atlasing is another technique I utilize to combine multiple textures into one, reducing draw calls and optimizing memory usage."
What to Look For:Candidates should demonstrate a strong understanding of performance optimization techniques and their impact on game performance. Look for examples of how they've successfully implemented these techniques in their projects and the results they achieved.
How to Answer:Candidates should define ScriptableObjects as custom data containers that can be saved as assets. They should explain how ScriptableObjects allow for data-driven development and how they're used to create customizable game elements.
Sample Answer:"ScriptableObjects are powerful data containers in Unity that allow us to create custom data structures and save them as assets. They are excellent for data-driven development, as they provide a way to create reusable and customizable game elements. For example, we can use ScriptableObjects to define different types of enemies or items, each with its own set of attributes and behaviors. This data-centric approach streamlines game development and allows for easier iteration and balancing."
What to Look For:Candidates should demonstrate a clear understanding of ScriptableObjects and their applications in data-driven game development. Look for examples of how they've utilized ScriptableObjects to create modular and customizable game elements in their projects.
How to Answer:Candidates should explain that Unity's UI system involves using the Event System to detect user input events like clicks and touches. They should discuss how to implement button interactions and validate user input to ensure a smooth user experience.
Sample Answer:"Handling user input and interactivity is vital for creating engaging user interfaces in Unity. I typically use Unity's Event System to detect input events, such as clicks and touches, on UI elements. Implementing button interactions is straightforward, as I attach functions to button click events to trigger actions in the game. Additionally, I ensure input validation to prevent errors and provide meaningful feedback to users, contributing to a seamless user experience."
What to Look For:Candidates should demonstrate competence in Unity's UI system and how they implement interactivity. Look for examples of user interfaces they've developed and how they've handled input validation to create user-friendly experiences.
How to Answer:Candidates should describe the process of importing assets from the Unity Asset Store and third-party plugins. They should discuss how these assets and plugins enhance their projects and save development time.
Sample Answer:"Integrating external assets and plugins is a common practice in Unity game development. The Unity Asset Store offers a vast collection of assets and scripts that I can easily import into my projects. I often use third-party plugins to add specific functionalities, such as analytics or ad networks, without having to build them from scratch. These assets and plugins save development time and improve the overall quality of my projects."
What to Look For:Candidates should demonstrate familiarity with importing assets from the Unity Asset Store and integrating third-party plugins. Look for examples of how they've used these resources to enhance their projects and streamline development.
How to Answer:Candidates should discuss how they adapt their games for different platforms, such as PC, consoles, and mobile devices. They should mention platform-specific features and performance tuning for each platform.
Sample Answer:"Cross-platform development is essential to reach a broader audience with our games. To optimize games for different platforms, I first identify platform-specific features and capabilities. For example, on mobile devices, I design intuitive touch controls to enhance gameplay. For PC and console platforms, I focus on leveraging high-quality graphics and supporting various input methods. Additionally, I adjust performance settings to ensure smooth gameplay on each platform, taking into account hardware specifications and limitations."
What to Look For:Candidates should demonstrate a comprehensive understanding of cross-platform development and the considerations involved in optimizing games for different platforms. Look for examples of how they've adapted their projects to run smoothly on various devices.
How to Answer:Candidates should explain their experience with version control systems and how they use them to manage project files, collaborate with team members, and track changes over time.
Sample Answer:"Version control is a crucial part of my Unity development workflow. I use Git to track changes, manage different project versions, and collaborate with team members. By committing code regularly, I ensure that the project history is well-documented and easily accessible. I also create branches to work on specific features or bug fixes separately, reducing the risk of conflicts during collaboration."
What to Look For:Candidates should demonstrate proficiency in using version control systems for Unity projects. Look for examples of how they've effectively managed and collaborated on projects using version control.
How to Answer:Candidates should explain how they plan and design game levels, considering factors like gameplay objectives, difficulty progression, and visual storytelling. They should emphasize creating a seamless game flow to keep players engaged.
Sample Answer:"Level design is a critical aspect of game development that requires careful planning and consideration. I begin by defining clear gameplay objectives for each level and ensuring a balanced difficulty curve. Visual storytelling is essential to immerse players in the game world and convey the game's narrative subtly. To maintain a cohesive game flow, I focus on seamless transitions between levels, minimizing interruptions and maintaining player engagement."
What to Look For:Candidates should demonstrate a thoughtful and strategic approach to level design. Look for examples of their level design work and how they've achieved a smooth game flow in their projects.
How to Answer:Candidates should discuss their process for implementing game mechanics, such as movement, combat, and inventory systems. They should explain how they design and integrate gameplay systems to create an immersive gaming experience.
Sample Answer:"Implementing game mechanics is an exciting part of game development. I start by breaking down each mechanic into manageable components and design the underlying systems to support them. For example, for a character movement mechanic, I create scripts to handle user input, apply forces to the character's Rigidbody, and manage animations. For more complex systems like inventory management, I use data-driven approaches and ScriptableObjects to create a flexible and customizable system for players to interact with items."
What to Look For:Candidates should demonstrate a creative and systematic approach to implementing game mechanics and systems. Look for examples of how they've designed and integrated various gameplay elements in their projects.
How to Answer:Candidates should share a specific challenge they encountered during a previous project and how they approached the problem-solving process to find a solution. They should highlight their critical thinking and troubleshooting skills.
Sample Answer:"In one of my past projects, I faced a performance issue with a complex particle system that caused significant frame rate drops. To address the problem, I used Unity's Profiler to identify the performance bottleneck and found that the particle system's overdraw was the main culprit. I optimized the particle material, reduced the particle count, and implemented object pooling to manage particles efficiently. These optimizations significantly improved performance and allowed the game to run smoothly."
What to Look For:Candidates should demonstrate their ability to identify and solve complex issues in Unity projects. Look for examples of how they've effectively used Unity tools like Profiler to optimize performance.
How to Answer:Candidates should explain how they communicate and collaborate with team members, ensuring effective coordination and alignment throughout the development process.
Sample Answer:"Team collaboration and communication are essential for a successful game development process. I believe in clear and frequent communication with team members, whether through meetings or project management tools. I actively listen to team feedback and provide constructive input to improve the project. I also believe in setting realistic goals and deadlines, ensuring that everyone is on the same page and motivated to achieve our objectives."
What to Look For:Candidates should demonstrate strong interpersonal and communication skills, along with the ability to work effectively in a team environment. Look for examples of their past team collaboration experiences and how they've contributed to successful projects.
How to Answer:Candidates should explain their decision-making process, considering factors like player feedback, design goals, and technical feasibility. They should demonstrate adaptability and flexibility in handling design changes or pivots during development.
Sample Answer:"In game development, decision-making is a balancing act that considers various aspects, including player feedback, design goals, and technical constraints. I regularly gather feedback from team members and playtesters to make informed decisions about the game's direction. When facing design changes or pivots, I remain flexible and collaborate with the team to evaluate the best course of action. It's essential to embrace changes and view them as opportunities for improvement rather than obstacles."
What to Look For:Candidates should demonstrate their ability to make informed decisions and adapt to changes during game development. Look for examples of how they've handled design changes and how these adaptations led to positive outcomes.
How to Answer:Candidates should highlight their unique strengths and experiences that differentiate them from other Unity developers. They should demonstrate their commitment to continuous learning and professional growth through self-improvement and staying updated on industry trends.
Sample Answer:"As a Unity developer, my strong grasp of scripting and performance optimization techniques allows me to create efficient and polished games. Additionally, I pride myself on my ability to collaborate effectively with teams and communicate complex technical concepts to non-technical stakeholders. To continue improving my skills, I actively participate in online communities, attend workshops and conferences, and read industry publications. Staying up-to-date with the latest Unity features and industry trends is crucial in the rapidly evolving world of game development."
What to Look For:Candidates should showcase their unique strengths and passion for self-improvement in the Unity development field. Look for examples of how they've sought opportunities for growth and how they stay informed about the latest developments in Unity.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Diversify your knowledge of potential job roles in the game development industry:
Create an online presence through platforms like GitHub, LinkedIn, and personal websites. Showcase your work, share knowledge through blog posts, and engage with the game development community.
Prepare to communicate your ideas clearly and concisely. Highlight your projects and experiences with enthusiasm, and explain complex concepts in simple terms.
Feeling nervous is natural, but proper preparation can help you feel more confident. Practice mock interviews with friends or mentors to reduce anxiety.
Prepare a few questions to ask the interviewer about the company culture, team dynamics, or specific projects they are working on. This demonstrates your interest and enthusiasm.
This guide on Unity interview questions equips you with the knowledge and insights to excel in your next Unity game development interview. From understanding fundamental Unity concepts and components to mastering scripting and performance optimization, you now have the tools to showcase your expertise confidently.
The provided sample answers serve as valuable references, offering inspiration on how to structure and articulate your responses. Remember to demonstrate problem-solving skills, creativity, and adaptability as you tackle real-world challenges in game development.
When preparing for your Unity interview, emphasize your experience with cross-platform development, integration of external assets and plugins, and a strong understanding of game design principles. Additionally, showcase your ability to collaborate with teams and communicate effectively, highlighting your unique contributions as a Unity developer.
By incorporating the tips and strategies shared in this guide, you'll stand out as a qualified and confident Unity developer, ready to take on the exciting and ever-evolving world of game development. Best of luck on your journey to success in Unity interviews and beyond!