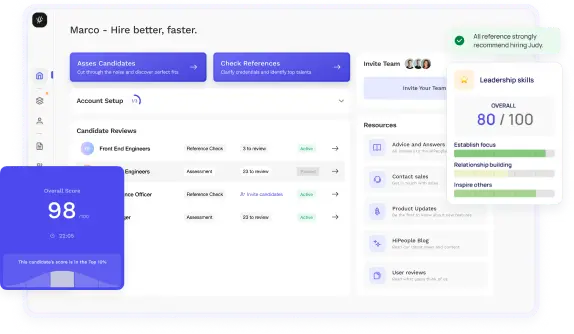
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Whether you're a beginner or an experienced Selenium professional, this guide will equip you with the knowledge and confidence to excel in your next interview. We'll cover the fundamentals of Selenium, dive into essential concepts, explore advanced topics, and provide a collection of commonly asked interview questions. So, let's get started!
Selenium, an open-source automation testing framework, plays a crucial role in the software testing process. Its ability to automate web browsers and perform functional testing across different platforms makes it highly valuable in ensuring the quality and reliability of web applications. As Selenium is widely used in the industry, interviewers often ask questions related to Selenium to assess a candidate's proficiency and understanding of web automation testing.
During interviews for software testing roles, interviewers frequently include Selenium-related questions to evaluate the candidate's knowledge, practical experience, problem-solving abilities, and familiarity with industry best practices. By asking these questions, interviewers aim to gauge your understanding of Selenium concepts, your ability to apply them in real-world scenarios, and your troubleshooting skills when facing challenges.
In this section, we'll establish a solid foundation by covering the fundamental aspects of Selenium. We'll explore what Selenium is, its various components, the programming languages it supports, and the different types of automation testing it enables.
Selenium is an open-source automation testing framework widely used for web application testing. It provides a suite of tools and libraries that allow testers to automate web browsers, interact with web elements, and perform functional testing across multiple platforms and browsers.
Selenium consists of several components that work together to enable web automation testing:
Selenium supports multiple programming languages, empowering you to write test scripts in a language of your choice. The commonly supported languages include:
Selenium supports various types of automation testing, allowing you to address different testing requirements:
With a solid understanding of Selenium fundamentals, you're now ready to explore the power of Selenium WebDriver, which forms the core of Selenium automation. Let's delve into WebDriver and learn how to automate browser actions and interact with web elements effectively.
Selenium WebDriver is a powerful tool that provides a programming interface for automating browser actions and interacting with web elements. In this section, we'll cover the essentials of WebDriver, including its architecture, element locating techniques, performing actions, handling frames and windows, and synchronization.
WebDriver acts as a bridge between your test scripts and web browsers, allowing you to automate browser actions programmatically. It provides a rich set of methods to interact with web elements, simulate user actions, and perform verifications.
The WebDriver architecture consists of three main components:
To interact with web elements, you need to locate them within the HTML structure of a web page. WebDriver provides various methods to locate elements based on different strategies:
WebDriver enables simulating user actions on web elements. You can perform various actions, such as clicking buttons, entering text into input fields, selecting options from dropdowns, and more. Here are some commonly used actions in WebDriver:
Web pages often contain frames (or iframes) and multiple windows. WebDriver provides methods to switch between frames and windows, allowing you to interact with elements within them. Here's how you can handle frames and windows with WebDriver:
Synchronization is crucial in Selenium automation to ensure that web elements are loaded and ready for interaction. WebDriver provides implicit and explicit waits to handle synchronization issues effectively:
By mastering Selenium WebDriver, you've acquired the ability to automate browser actions, interact with web elements, and handle synchronization effectively. Next, we'll explore how to handle different types of web elements in Selenium, such as textboxes, dropdowns, alerts, and more.
Interacting with various web elements is a fundamental aspect of Selenium automation. In this section, we'll dive deep into handling different types of web elements using Selenium WebDriver. We'll cover textboxes, dropdowns, radio buttons, checkboxes, alerts, uploading and downloading files, and handling dynamic elements.
Textboxes and input fields are commonly used elements on web forms. Selenium provides methods to interact with these elements effectively. Here's how you can perform actions on textboxes and input fields:
Dropdown menus and select boxes allow users to choose options from a list. Selenium enables you to interact with dropdowns programmatically. Here's how you can handle dropdowns and select boxes:
Radio buttons and checkboxes are used to make single or multiple selections. Selenium provides methods to interact with these elements efficiently. Here's how you can handle radio buttons and checkboxes:
Web applications often display alerts or pop-ups to provide important information or prompt user interactions. Selenium allows you to handle these alerts and pop-ups effectively. Here's how you can handle alerts and pop-ups using WebDriver:
Web applications frequently require file upload or download operations. Selenium provides mechanisms to handle these scenarios. Here's how you can handle file upload and download using WebDriver:
Dynamic elements on a web page change their properties, attributes, or positions based on user interactions or underlying application behavior. Selenium allows you to handle dynamic elements by implementing suitable strategies:
With a strong understanding of handling different web elements, you possess the skills to interact with textboxes, dropdowns, radio buttons, checkboxes, alerts, file uploads, downloads, and dynamic elements using Selenium WebDriver. Next, let's explore how to handle various browser actions to enhance your Selenium automation capabilities.
In Selenium automation, you often need to perform various browser-specific actions to simulate user interactions or manipulate browser settings. In this section, we'll cover how to handle different browser actions using Selenium WebDriver. We'll explore browser navigation, cookie handling, JavaScript alerts, taking screenshots and capturing videos, and executing JavaScript code.
Selenium WebDriver provides methods to navigate through web pages and control browser history. Here's how you can perform browser navigation and history-related actions:
Cookies are small pieces of data stored by websites on a user's computer. Selenium allows you to manipulate cookies during your automation tasks. Here's how you can handle cookies using WebDriver:
JavaScript alerts, confirmations, and prompts are common elements in web applications. Selenium WebDriver enables you to handle JavaScript-based pop-ups effectively. Here's how you can interact with JavaScript alerts and pop-ups:
Visual evidence is essential in test automation for debugging and reporting purposes. Selenium WebDriver allows you to capture screenshots and even record videos of your test execution. Here's how you can take screenshots and capture videos:
Selenium WebDriver enables you to execute JavaScript code directly in the browser. This functionality provides flexibility and allows you to interact with web elements or manipulate the DOM. Here's how you can execute JavaScript code using WebDriver:
With the knowledge of handling different browser actions, you now possess the skills to navigate through web pages, manage cookies, handle JavaScript alerts and pop-ups, capture screenshots, and execute JavaScript code using Selenium WebDriver. Next, let's explore Selenium Grid, which enables distributed and parallel test execution across multiple machines.
Selenium Grid is a powerful feature that allows you to run tests in parallel across multiple machines and browsers, enhancing test execution efficiency. In this section, we'll cover the essentials of Selenium Grid, including its introduction, setting up a Selenium Grid environment, configuring hub and nodes, and running tests on a Selenium Grid.
Selenium Grid enables you to distribute your test execution on different machines, operating systems, and browsers simultaneously. By leveraging Selenium Grid, you can reduce test execution time significantly, increase test coverage, and improve overall efficiency.
To set up a Selenium Grid environment, you need to configure a hub and one or more nodes. The hub acts as the central point for distributing tests, while the nodes are the machines that execute the tests. Here are the steps to set up a Selenium Grid environment:
When configuring the hub and nodes in Selenium Grid, you have several options to customize the setup based on your requirements. Here are some important configurations you can consider:
Once you have your Selenium Grid environment set up, you can execute your tests by targeting the hub. Selenium WebDriver provides capabilities to connect to the hub and run tests on the available nodes. Here's how you can run tests on a Selenium Grid:
By leveraging Selenium Grid, you can achieve parallel and distributed test execution, effectively utilizing multiple machines and browsers. This helps you optimize test execution time and improve the scalability of your automation suite. Next, let's explore TestNG, a popular testing framework, and its integration with Selenium.
TestNG is a robust testing framework that provides advanced test execution, reporting, and configuration capabilities. In this section, we'll explore TestNG and its integration with Selenium. We'll cover the introduction to TestNG, using TestNG annotations in Selenium, working with TestNG assertions, and implementing data-driven testing using TestNG.
TestNG is a testing framework that simplifies the automation of test scenarios, enabling better control over test execution and improved reporting. TestNG offers a wide range of features, including parallel test execution, data-driven testing, dependency management, and test configuration flexibility.
TestNG leverages annotations to define the behavior and characteristics of test methods. Selenium seamlessly integrates with TestNG, allowing you to utilize annotations to enhance your test scripts. Here are some commonly used TestNG annotations and their usage in Selenium:
TestNG provides a range of assertion methods to verify expected conditions during test execution. These assertions help you validate the behavior of your application and provide precise failure information. Here are some commonly used TestNG assertions in Selenium:
TestNG also offers robust reporting capabilities that generate detailed test reports, including test execution summaries, failure details, and logs. These reports assist in identifying issues and analyzing test results effectively.
Data-driven testing allows you to execute the same test case with different sets of test data, enhancing test coverage and flexibility. TestNG simplifies data-driven testing by integrating seamlessly with external data sources. Here's how you can implement data-driven testing using TestNG:
By integrating Selenium with TestNG, you can leverage TestNG's advanced features to enhance your test automation suite. TestNG's annotations, assertions, reporting, and data-driven testing capabilities empower you to create robust and efficient test scripts. Next, let's explore Selenium frameworks, which provide structure and best practices for organizing your Selenium test code.
Selenium frameworks offer structure and best practices for organizing and maintaining your Selenium test code. In this section, we'll explore popular Selenium frameworks, including the Page Object Model (POM), Keyword-driven framework, Data-driven framework, Hybrid framework, and discuss best practices for building Selenium frameworks.
The Page Object Model (POM) is a design pattern that promotes maintainability and reusability in Selenium test automation. POM divides web pages into separate classes, called Page Objects, where each class represents a specific web page or a component within a page. Here's how the POM works:
The Keyword-driven framework allows testers to create tests using a set of keywords and associated actions or methods. It separates test script logic from the underlying implementation, making test maintenance easier. Here's how the Keyword-driven framework operates:
The Data-driven framework enables testers to separate test data from test scripts. It allows you to define test scenarios in a tabular format, where each row represents a test case with different input data. Here's how the Data-driven framework works:
The Hybrid framework combines the strengths of multiple frameworks, incorporating the best practices from each. It offers flexibility and scalability while maintaining code organization and reusability. The Hybrid framework allows you to choose the most suitable framework components based on your project requirements.
When building Selenium frameworks, it's essential to follow best practices to ensure maintainability, reusability, and scalability. Here are some best practices to consider:
By implementing Selenium frameworks like the Page Object Model, Keyword-driven, Data-driven, or a Hybrid approach, you can organize your Selenium test code effectively, enhance reusability, and improve the maintainability of your test suite. With a strong foundation in Selenium frameworks, you're now equipped to tackle more advanced concepts in Selenium automation.
In this section, we'll explore advanced concepts in Selenium automation, covering implicit and explicit waits, handling multiple windows and tabs, mouse and keyboard actions using Actions class, handling iframes and nested frames, managing SSL certificates and insecure websites, and addressing browser-specific functionalities.
Selenium provides implicit and explicit waits to handle synchronization issues effectively. These waits allow your test script to wait for a certain condition to be met before proceeding further. Here's how you can use implicit and explicit waits in Selenium:
Web applications often involve multiple windows or tabs, requiring you to switch focus between them during test execution. Selenium provides methods to handle multiple windows and tabs effectively. Here's how you can manage multiple windows and tabs using WebDriver:
Selenium WebDriver enables you to perform advanced mouse and keyboard actions using the Actions class. This class provides methods to simulate complex user interactions. Here are some actions you can perform using the Actions class:
Web pages often contain iframes (inline frames) or nested frames, which require special handling during test automation. Selenium WebDriver provides methods to interact with elements inside iframes effectively. Here's how you can handle iframes and nested frames:
Some web applications use SSL certificates for secure communication. However, during testing, you may encounter scenarios where you need to handle SSL certificates or interact with insecure websites. Selenium WebDriver provides options to address these situations. Here's how you can handle SSL certificates and insecure websites:
Different browsers offer unique functionalities or behaviors that may require special handling during test automation. Selenium WebDriver allows you to address browser-specific functionalities by utilizing browser-specific capabilities. Here are some examples:
By understanding advanced Selenium concepts like implicit and explicit waits, handling multiple windows and tabs, performing mouse and keyboard actions, managing iframes, addressing SSL certificates and insecure websites, and handling browser-specific functionalities, you have acquired the skills to tackle complex test scenarios and overcome common challenges in Selenium automation.
How to Answer: Explain that Selenium is an open-source automation testing tool used for web application testing. Discuss its components, including Selenium WebDriver, Selenium IDE, and Selenium Grid. Describe how Selenium interacts with browsers using the WebDriver API to automate user actions.
Sample Answer: "Selenium is a popular open-source automation testing tool used for testing web applications. It consists of different components, such as Selenium WebDriver for browser automation, Selenium IDE for record and playback, and Selenium Grid for parallel test execution. Selenium WebDriver interacts with browsers using the WebDriver API, allowing testers to automate user actions like clicking buttons, filling forms, and validating results."
What to Look For: Look for a clear and concise explanation of Selenium and its components. Candidates should demonstrate a solid understanding of how Selenium interacts with browsers and its role in web application testing.
How to Answer: Highlight the key advantages of Selenium, such as its open-source nature, cross-browser compatibility, multi-language support, and robust community support. Discuss its flexibility, scalability, and the ability to integrate with other frameworks and tools.
Sample Answer: "Selenium offers several advantages over other testing tools. First, it is open-source, which means it is free to use and has a vast community of contributors. Second, Selenium supports multiple programming languages like Java, Python, and C#, allowing testers to use their preferred language. Third, it provides cross-browser compatibility, enabling tests to be executed on different browsers. Finally, Selenium can be integrated with various frameworks and tools, making it flexible and scalable for different test automation needs."
What to Look For: Look for candidates who can articulate the advantages of Selenium clearly and highlight its strengths compared to other testing tools. Candidates should emphasize the benefits of open-source, language support, cross-browser compatibility, and integration capabilities.
How to Answer: Explain that Selenium WebDriver is a powerful tool for browser automation, providing a programming interface to interact with web elements. Differentiate it from Selenium RC by highlighting that WebDriver uses native browser automation techniques, has a simpler architecture, and offers better performance.
Sample Answer: "Selenium WebDriver is a tool in the Selenium suite that allows testers to automate web browsers using a programming interface. It provides a more intuitive and straightforward approach compared to Selenium RC. WebDriver uses the native automation capabilities of each browser, allowing it to interact with web elements directly. This results in faster and more reliable test execution. Unlike Selenium RC, WebDriver has a simpler architecture and supports multiple programming languages, making it a popular choice for web application testing."
What to Look For: Look for candidates who can clearly differentiate Selenium WebDriver from Selenium RC and highlight the advantages of using WebDriver. They should demonstrate knowledge of WebDriver's native browser automation approach and its impact on performance and reliability.
How to Answer: Explain the different methods to locate elements, including by ID, name, class name, tag name, link text, partial link text, CSS selector, and XPath. Emphasize the importance of choosing appropriate locators based on the element's unique attributes and the efficiency of the locator strategy.
Sample Answer: "Selenium WebDriver provides various methods to locate elements on a web page. We can locate elements by their ID, name, class name, tag name, link text, partial link text, CSS selector, or XPath. The choice of locator depends on the uniqueness of the element and the efficiency of the locator strategy. It's essential to select locators that are stable and unlikely to change with future modifications to the web page."
What to Look For: Look for candidates who can demonstrate a solid understanding of different locator strategies in Selenium WebDriver. They should be able to explain the various methods and emphasize the importance of choosing appropriate locators based on element uniqueness and stability.
How to Answer: Explain that WebDriver provides methods to switch focus between frames and windows. Describe how to switch to a frame using the switchTo().frame() method by index, name, or element. Discuss switching to a new window using the switchTo().window() method by handle or title.
Sample Answer: "To handle frames in Selenium WebDriver, we use the switchTo().frame() method. We can switch to a frame by index, name, or element. For example, driver.switchTo().frame(0) switches to the first frame on the page. To handle windows, we use the switchTo().window() method. We can switch to a window by its handle or title. For instance, driver.switchTo().window("windowHandle") switches to a window with a specific handle."
What to Look For: Look for candidates who can demonstrate proficiency in handling frames and windows using Selenium WebDriver. They should explain the methods to switch focus, mention the available options like index, name, element, handle, or title, and provide clear examples.
How to Answer: Explain that Selenium provides the sendKeys() method to enter text into textboxes and input fields. Emphasize the importance of clearing existing text using the clear() method before entering new text. Highlight that text can be retrieved from textboxes using the getAttribute() method.
Sample Answer: "To interact with textboxes and input fields in Selenium, we use the sendKeys() method. It allows us to enter text by simulating keyboard input. Before entering new text, it's crucial to clear any existing text in the field using the clear() method. We can retrieve the text from a textbox or input field using the getAttribute() method with the 'value' attribute."
What to Look For: Look for candidates who can explain the essential methods to interact with textboxes and input fields in Selenium. They should highlight the sendKeys() method for entering text, mention the need to clear existing text, and discuss retrieving text using the getAttribute() method.
How to Answer: Explain that Selenium provides methods to select options from dropdowns and select boxes. Describe using the selectByVisibleText(), selectByValue(), and selectByIndex() methods. Highlight that for multi-select dropdowns, options can be deselected using the deselectAll() or deselectBy...() methods.
Sample Answer: "To handle dropdowns and select boxes in Selenium, we use methods from the Select class. We can select options based on visible text using the selectByVisibleText() method. Alternatively, we can select options by their values using selectByValue(), or by their indexes using selectByIndex(). For multi-select dropdowns, we can deselect options using the deselectAll() method or deselectBy...() methods."
What to Look For: Look for candidates who can explain the methods to handle dropdowns and select boxes in Selenium. They should mention the selectByVisibleText(), selectByValue(), and selectByIndex() methods, and discuss deselecting options for multi-select dropdowns.
How to Answer: Explain that Selenium provides methods to interact with radio buttons and checkboxes. Describe using the click() method to select radio buttons or checkboxes. Emphasize the importance of verifying the selected state using the isSelected() method.
Sample Answer: "To select radio buttons and checkboxes using Selenium, we can use the click() method. It simulates a user click on the element, thereby selecting it. After selecting the element, we can verify its selected state using the isSelected() method. It returns a boolean value indicating whether the element is selected or not."
What to Look For: Look for candidates who can explain the methods to select radio buttons and checkboxes using Selenium. They should mention the click() method, highlight the need to verify the selected state, and discuss using the isSelected() method.
How to Answer: Explain that Selenium provides methods to handle alerts and pop-ups. Describe using the switchTo().alert() method to switch to an alert and interact with it using the accept(), dismiss(), or sendKeys() methods. Highlight the importance of handling alerts gracefully using try-catch blocks.
Sample Answer: "To handle alerts and pop-ups in Selenium, we use the switchTo().alert() method. It allows us to switch the WebDriver's focus to the alert and interact with it using methods like accept(), dismiss(), or sendKeys() for entering text. It's essential to handle alerts gracefully by using try-catch blocks to catch any exceptions that may occur."
What to Look For: Look for candidates who can explain the methods to handle alerts and pop-ups in Selenium. They should discuss the switchTo().alert() method and mention the available methods like accept(), dismiss(), or sendKeys(). Candidates should also emphasize the need to handle alerts gracefully using try-catch blocks.
How to Answer: Explain that Selenium cannot directly interact with the OS-level file upload and download dialogs. Describe approaches to handle file uploads, such as using the sendKeys() method to enter the file path into the file input field. Discuss handling file downloads by modifying browser settings or using third-party libraries or tools.
Sample Answer: "Selenium cannot interact with the OS-level file upload and download dialogs directly. For file uploads, we can use the sendKeys() method to enter the file path into the file input field on the web page. However, for file downloads, Selenium doesn't provide built-in capabilities. We can modify browser settings to specify the desired download location or use third-party libraries or tools to handle file downloads."
What to Look For: Look for candidates who can explain the limitations of Selenium in handling file uploads and downloads. They should discuss the approach of using sendKeys() for file uploads and mention alternatives for handling file downloads, such as modifying browser settings or utilizing external libraries or tools.
How to Answer: Explain that Selenium WebDriver provides methods to navigate through different pages. Describe using the get() method to open a specific URL, the navigate().to() method to navigate to a new page, the navigate().back() method to go back, and the navigate().forward() method to go forward.
Sample Answer: "To navigate through different pages using Selenium WebDriver, we have several methods at our disposal. We can use the get() method to open a specific URL, the navigate().to() method to navigate to a new page, the navigate().back() method to go back to the previous page, and the navigate().forward() method to move forward to the next page in the browsing history."
What to Look For: Look for candidates who can explain the methods to navigate through different pages using Selenium WebDriver. They should mention the get(), navigate().to(), navigate().back(), and navigate().forward() methods and provide a clear understanding of their purposes.
How to Answer: Explain that Selenium WebDriver provides methods to handle cookies. Describe using the addCookie() method to add a new cookie, the getCookieNamed() method to retrieve a specific cookie, and the deleteCookieNamed() method to delete a cookie by name.
Sample Answer: "Selenium WebDriver allows us to handle cookies using methods like addCookie(), getCookieNamed(), and deleteCookieNamed(). We can use the addCookie() method to add a new cookie, providing the necessary details like name, value, domain, and path. The getCookieNamed() method retrieves a specific cookie based on its name, while the deleteCookieNamed() method allows us to delete a cookie by providing its name."
What to Look For: Look for candidates who can explain the methods to handle cookies in Selenium WebDriver. They should discuss the addCookie(), getCookieNamed(), and deleteCookieNamed() methods, and highlight their purposes and usage.
How to Answer: Explain that Selenium WebDriver provides methods to handle JavaScript alerts and pop-ups. Describe using the switchTo().alert() method to switch to an alert and interact with it using the accept(), dismiss(), or sendKeys() methods. Emphasize the importance of handling alerts gracefully using try-catch blocks.
Sample Answer: "To handle JavaScript alerts and pop-ups in Selenium WebDriver, we can use the switchTo().alert() method. It allows us to switch the WebDriver's focus to the alert and interact with it using methods like accept(), dismiss(), or sendKeys() for entering text. It's essential to handle alerts gracefully by using try-catch blocks to catch any exceptions that may occur."
What to Look For: Look for candidates who can explain the methods to handle JavaScript alerts and pop-ups in Selenium WebDriver. They should discuss the switchTo().alert() method and mention the available methods like accept(), dismiss(), or sendKeys(). Candidates should also emphasize the need to handle alerts gracefully using try-catch blocks.
How to Answer: Explain that Selenium WebDriver provides methods to capture screenshots but does not provide direct video capture capabilities. Describe using the getScreenshotAs() method to capture screenshots of the current browser window. Mention that video recording can be achieved using third-party libraries or tools while executing WebDriver tests.
Sample Answer: "Selenium WebDriver allows us to capture screenshots of the current browser window using the getScreenshotAs() method. However, WebDriver does not provide direct video capture capabilities. If video recording is required during test execution, we can utilize third-party libraries or tools specifically designed for recording videos while executing WebDriver tests."
What to Look For: Look for candidates who can explain the method to capture screenshots using Selenium WebDriver. They should mention the getScreenshotAs() method and clarify that video recording is not directly supported by WebDriver but can be achieved using external libraries or tools.
How to Answer: Explain that Selenium WebDriver allows the execution of JavaScript code using the executeScript() method. Describe how this method enables running JavaScript directly within the browser context, allowing interactions with DOM elements and leveraging browser-specific functionalities.
Sample Answer: "Selenium WebDriver provides the executeScript() method, which allows us to execute JavaScript code within the browser context. This method enables interactions with DOM elements, manipulation of web page elements, and accessing browser-specific functionalities that are not directly supported by WebDriver. It provides the flexibility to extend test capabilities by utilizing JavaScript in test automation scenarios."
What to Look For: Look for candidates who can explain the executeScript() method in Selenium WebDriver. They should emphasize its ability to execute JavaScript code within the browser context and highlight the advantages of leveraging JavaScript for extending test capabilities.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
We've covered the fundamentals of Selenium, delved into essential concepts, explored advanced topics, and provided a collection of commonly asked interview questions. By understanding and practicing the concepts discussed in this guide, you're well-equipped to excel in your Selenium interviews.
Remember, preparation and hands-on experience are key to success. Keep practicing your automation skills, explore real-world scenarios, and stay up-to-date with the latest trends and advancements in Selenium and test automation. Good luck with your interviews, and may your Selenium journey be filled with success!