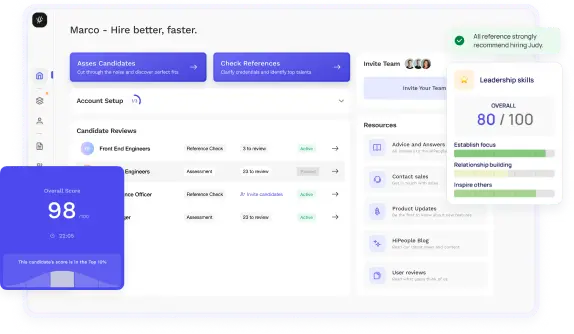
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you looking to kick-start your career as a React Native developer or aiming to switch jobs? Or are you an employer seeking to hire talented React Native developers? In either case, having a strong grasp of React Native interview questions is essential.
This guide covers everything you need to know to excel in React Native interviews, from fundamental concepts to advanced topics. We'll walk you through the core principles, essential APIs, debugging techniques, cross-platform development, testing, and much more!
Before diving into the technical aspects, let's understand the significance of React Native and why being well-prepared for interviews is crucial for your success as a React Native developer.
React Native has revolutionized mobile app development by allowing developers to build cross-platform applications using the same codebase. It's an open-source framework developed by Facebook that combines the power of React, a popular JavaScript library for web development, with native app development capabilities. With React Native, you can create high-performance and visually appealing mobile apps for both iOS and Android platforms, resulting in faster development cycles and cost savings.
As React Native continues to gain popularity in the mobile app development landscape, companies are actively seeking skilled React Native developers. Interviewing for React Native roles requires a deep understanding of the framework's concepts, best practices, and how to tackle real-world challenges. For employers, asking the right questions helps identify candidates with the expertise needed to contribute effectively to their development teams.
Before we dive into the technical aspects, let's equip you with some invaluable tips to prepare for your React Native interviews:
We'll start by covering the foundational concepts of React Native that you need to grasp before moving on to more advanced topics.
React Native is a JavaScript framework used for building native mobile applications. It allows developers to use React components to create a UI for both Android and iOS platforms using a single codebase. Some key points about React Native are:
Although React and React Native share similarities, there are essential differences that you should be aware of:
React Native utilizes a Virtual DOM, which is a lightweight representation of the actual DOM. When state changes occur, React Native creates a new Virtual DOM, performs a diffing process, and updates only the changed components on the actual native view. This process results in efficient UI updates and enhanced app performance.
Components are the building blocks of a React Native application. They are reusable, self-contained pieces of UI that can be combined to create the entire user interface. Understanding components and their lifecycle methods is crucial for efficient app development.
React Native uses a bridge to communicate between JavaScript and native modules. JavaScript code runs in a separate thread called the "JS thread," while native code runs on the main UI thread. The bridge handles communication and synchronization between these threads, allowing developers to access native APIs and create native modules to extend React Native's functionality.
As with any technology, React Native comes with its strengths and weaknesses. Understanding these will help you make informed decisions when choosing React Native for your app development:
Pros:
Cons:
Let's explore the core concepts that every React Native developer should be familiar with.
JSX is a syntax extension for JavaScript that allows developers to write XML-like code directly within JavaScript. It provides a more concise and expressive way to create React components. In React Native, you'll often find JSX used to define the UI components and their structure.
Here's how JSX works:
import React from 'react';
import { View, Text } from 'react-native';
const MyComponent = () => {
return (
<View>
<Text>Hello, World!</Text>
</View>
);
};
export default MyComponent;
Props and state are essential concepts in React Native, as they determine how data flows within the application and how the UI responds to changes.
Handling user interactions is crucial in any app. React Native provides a straightforward way to handle events, such as button clicks, text input, and more.
Here's how you can handle a button press event in React Native:
import React from 'react';
import { View, Button, Alert } from 'react-native';
const MyComponent = () => {
const handleButtonPress = () => {
Alert.alert('Button Pressed!');
};
return (
<View>
<Button title="Press Me" onPress={handleButtonPress} />
</View>
);
};
export default MyComponent;
Styling in React Native uses a flexbox-based system, similar to web development with CSS flexbox. Flexbox makes it easy to create responsive and adaptive layouts for different screen sizes and orientations.
Key points about styling in React Native:
Here's an example of using flexbox for layout:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const MyComponent = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, Flexbox!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 20,
fontWeight: 'bold',
},
});
export default MyComponent;
Navigation is a crucial aspect of any mobile app. React Native offers various navigation libraries to handle routing and transitions between screens. The most popular ones include React Navigation and React Native Navigation.
React Navigation is a JavaScript-based library that allows you to define your app's navigation structure using a declarative approach. It provides a stack navigator, tab navigator, drawer navigator, and more.
React Native Navigation, on the other hand, uses native navigation controllers for each platform, resulting in smoother transitions and improved performance.
Each library has its pros and cons, so choose the one that best suits your project requirements.
React Native provides a vast set of APIs and third-party libraries to enhance your app's functionality and access platform-specific features. Let's dive into some key APIs and libraries you should be familiar with.
One of the key strengths of React Native is its ability to interact with native code through native modules and native UI components.
Mobile apps often require data persistence to store user preferences, settings, or cached data. React Native provides two popular options for data persistence:
Fetching data from a server is a common requirement in mobile apps. React Native's built-in Fetch API makes it easy to perform HTTP requests.
Here's an example of making a simple GET request:
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((data) => {
// Process the data here
})
.catch((error) => {
console.error('Error fetching data:', error);
});
Redux is a popular state management library for React and React Native applications. It helps manage the state of your app in a predictable and centralized way.
Here's a high-level overview of how Redux works in a React Native app:
Integrating Redux in your React Native project can lead to more maintainable and scalable code.
React Native's vast ecosystem includes a plethora of third-party libraries that can boost your app's functionality. Whether you need UI components, navigation solutions, animations, or backend integrations, there's likely a library available for your specific needs.
When choosing a third-party library, consider factors like the library's popularity, maintenance, and community support. Be cautious of using outdated or unmaintained libraries, as they may cause compatibility issues or security vulnerabilities.
Congratulations on mastering the core concepts of React Native! Now, let's dive into more advanced topics that will further enhance your React Native development skills.
Smooth and fluid animations are essential for creating engaging user experiences. React Native provides powerful APIs for handling animations and gestures.
Here are some key concepts related to animations and gestures:
Making your app accessible is not only essential for reaching a broader audience but also the right thing to do. React Native offers built-in support for accessibility by providing components with accessible properties and roles.
To make your app accessible:
If your app needs to support multiple languages and locales, internationalization is crucial. React Native offers solutions for internationalizing your app and handling translations.
One popular library for i18n in React Native is react-i18next, which simplifies the process of translating strings and handling language changes.
Efficiently optimizing the performance of your React Native app is vital for a smooth user experience and positive app reviews. Some strategies for optimizing performance include:
Memory leaks can lead to app crashes and performance issues. React Native provides tools for debugging and monitoring memory usage.
Use the Memory Profiler in React Native's developer menu to identify memory leaks and optimize memory usage.
For larger applications, code-splitting and lazy loading can significantly improve the app's initial load time and reduce the bundle size. React Native supports dynamic imports for lazy loading, making it easier to load modules on-demand.
Cross-platform development is one of the key advantages of React Native. It allows you to write code once and deploy it on both Android and iOS platforms.
Cross-platform development offers several benefits, including:
Although React Native enables cross-platform development, you may encounter situations where platform-specific code is necessary. For instance, certain APIs or UI components may behave differently on Android and iOS.
React Native provides a platform module named Platform that allows you to detect the current platform and conditionally render components or apply platform-specific styles.
import { View, Text, Platform, StyleSheet } from 'react-native';
const MyComponent = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>
{Platform.OS === 'ios' ? 'Running on iOS' : 'Running on Android'}
</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 20,
fontWeight: 'bold',
},
});
export default MyComponent;
React Native provides a wide range of APIs for accessing device-specific features, such as camera, location services, and sensors. However, these APIs may not be available or behave differently on every device.
To ensure smooth functionality across various devices:
Remember to test your app on different devices and emulators to ensure compatibility.
Cross-platform development also means that your app should adapt well to various screen sizes and resolutions. To achieve this:
Ensuring that your React Native app works flawlessly and delivering it to users are crucial steps in the development process.
Testing is vital to validate your app's functionality and identify potential bugs. There are various testing strategies you can employ:
Jest is a popular testing framework that comes pre-installed with React Native projects. It provides a simple and efficient way to write and run unit tests.
Enzyme, on the other hand, is a testing utility for React that complements Jest by providing additional features for testing React components' rendering and behavior.
To write a basic unit test using Jest and Enzyme:
import React from 'react';
import { shallow } from 'enzyme';
import MyComponent from './MyComponent';
it('renders correctly', () => {
const wrapper = shallow(<MyComponent />);
expect(wrapper).toMatchSnapshot();
});
Detox is a powerful E2E testing framework specifically designed for React Native apps. It allows you to simulate user interactions and verify the app's behavior on real devices and emulators.
To run E2E tests with Detox:
Continuous integration (CI) and continuous delivery (CD) are crucial practices for maintaining code quality and ensuring smooth app deployments.
With CI/CD pipelines in place:
Popular CI/CD platforms like Jenkins, CircleCI, and GitHub Actions can be integrated into your React Native project to set up CI/CD workflows.
Congratulations! Your React Native app is ready for deployment. There are two main ways to distribute your app to users:
Remember to test your app thoroughly on different devices and OS versions before submitting it to the app stores.
How to Answer: Start by explaining that React Native is a JavaScript framework for building cross-platform mobile applications. Highlight its ability to use a single codebase to target both iOS and Android platforms. Compare it to React, emphasizing that while React is for web development and renders to the DOM, React Native renders to native components.
Sample Answer: "React Native is a JavaScript framework used for building cross-platform mobile applications. It allows developers to use a single codebase to create apps for both iOS and Android platforms. In contrast, React is a JavaScript library for web development, rendering components to the DOM. React Native, on the other hand, renders components to native views, resulting in a native-like user experience."
What to Look For: Look for candidates who can clearly articulate the differences between React and React Native and understand the advantages of using React Native for mobile app development.
How to Answer: Explain that React Native uses a bridge to communicate between JavaScript and native modules. JavaScript code runs on a separate thread called the "JS thread," while native code runs on the main UI thread. The bridge handles communication and synchronization between these threads, allowing developers to access native APIs.
Sample Answer: "React Native achieves cross-platform compatibility through a bridge that connects JavaScript and native modules. JavaScript runs on the JS thread, while native code runs on the main UI thread. The bridge facilitates communication between these threads, enabling developers to access platform-specific features using native modules."
What to Look For: Seek candidates who can provide a clear explanation of how the React Native bridge works and its role in achieving cross-platform compatibility.
How to Answer: Explain that functional components are simpler and easier to read, as they are written as pure JavaScript functions. Class components, on the other hand, are more feature-rich, supporting state, lifecycle methods, and more. Mention that with the introduction of React Hooks, functional components can now handle state and lifecycle similar to class components.
Sample Answer: "Functional components are written as pure JavaScript functions, making them simpler and easier to read. Class components, on the other hand, are JavaScript classes with additional features like state and lifecycle methods. However, with the introduction of React Hooks, functional components can now handle state and lifecycle just like class components."
What to Look For: Look for candidates who understand the differences between functional and class components, as well as their similarities with the advent of React Hooks.
How to Answer: Explain that data is passed from parent to child components through props. The parent component sets attributes on the child component, which are then accessible as props inside the child component.
Sample Answer: "In React Native, data is passed from a parent component to a child component through props. The parent component sets attributes on the child component, and those attributes are accessible as props inside the child component."
What to Look For: Seek candidates who understand the concept of props and can explain how data flows from parent to child components.
How to Answer: Mention that the component lifecycle methods in React Native allow developers to perform actions at specific stages of a component's life. These methods include componentDidMount, componentDidUpdate, and componentWillUnmount.
Sample Answer: "The component lifecycle methods in React Native allow developers to perform actions at specific stages of a component's life. For example, componentDidMount is called after a component is rendered to the screen, componentDidUpdate is called when the component's props or state change, and componentWillUnmount is called just before a component is removed from the screen."
What to Look For: Look for candidates who can provide a basic understanding of the core component lifecycle methods and when they are invoked.
How to Answer: Explain that state represents mutable data that belongs to a component and can be changed using setState. In contrast, props are immutable data passed from parent to child components.
Sample Answer: "In React Native, state represents mutable data that belongs to a component and can be changed using the setState method. On the other hand, props are immutable data passed from parent to child components, allowing the parent to communicate with its children."
What to Look For: Seek candidates who understand the difference between state and props and can explain their respective roles in React Native applications.
How to Answer: Explain that Redux is a state management library that provides a centralized store to manage the application's state. It solves the problem of state management complexity and helps in maintaining a predictable and consistent application state.
Sample Answer: "Redux is a state management library that provides a centralized store to manage the application's state. It solves the problem of state management complexity by offering a single source of truth for the entire application. With Redux, components can access the application state more efficiently, leading to a more predictable and consistent user experience."
What to Look For: Look for candidates who can explain the purpose of Redux and its benefits in managing the application state effectively.
How to Answer: Mention that the process involves using the connect function from the react-redux library. The connect function connects the component to the Redux store, allowing it to access state and dispatch actions.
Sample Answer: "To connect a React Native component to the Redux store, we use the connect function from the react-redux library. The connect function maps the state and action creators to the component's props, enabling the component to access state and dispatch actions."
What to Look For: Seek candidates who understand the steps involved in connecting a React Native component to the Redux store and can explain the role of the connect function.
How to Answer: Explain that performance optimization in React Native involves various techniques such as using PureComponent or memo to prevent unnecessary re-renders, implementing code-splitting to load components on-demand, and utilizing the VirtualizedList component for efficient rendering of large lists.
Sample Answer: "Performance optimization in React Native can be achieved through various techniques. Using PureComponent or memo helps prevent unnecessary re-renders, reducing the workload on the app. Implementing code-splitting and lazy loading allows components to be loaded on-demand, leading to faster initial load times. Additionally, using the VirtualizedList component efficiently renders large lists, optimizing the app's performance when handling extensive data."
What to Look For: Look for candidates who can identify and explain various techniques to optimize the performance of a React Native app.
How to Answer: Explain that code-splitting is the process of splitting the JavaScript bundle into smaller chunks to load only the necessary code on demand. This reduces the initial bundle size and improves the app's load time.
Sample Answer: "Code-splitting is the practice of breaking the JavaScript bundle into smaller chunks. By loading only the required code when needed, code-splitting reduces the initial bundle size, leading to faster load times and improved app performance."
What to Look For: Seek candidates who can provide a clear explanation of code-splitting and its role in improving app performance.
How to Answer: Explain that React Native provides the Platform module to detect the current platform and apply platform-specific code. Use conditional rendering or create platform-specific files using file extensions (e.g., .ios.js and .android.js) to manage platform-specific code.
Sample Answer: "React Native offers the Platform module, allowing developers to detect the current platform and apply platform-specific code. We can use conditional rendering or create platform-specific files (e.g., .ios.js and .android.js) to manage platform-specific code. This ensures that the appropriate code is executed based on the user's device platform."
What to Look For: Look for candidates who can demonstrate an understanding of handling platform-specific code in React Native and can provide examples of conditional rendering or file separation for different platforms.
How to Answer: Explain that integrating a native module involves writing platform-specific code in native languages (Objective-C/Swift for iOS, Java/Kotlin for Android) and using the React Native bridge to communicate with JavaScript. The module is then imported and used like any other JavaScript module in the app.
Sample Answer: "To integrate a native module into a React Native app, we need to write platform-specific code in native languages (Objective-C/Swift for iOS, Java/Kotlin for Android). We then use the React Native bridge to communicate with JavaScript. Once the module is set up, we can import and use it like any other JavaScript module in our app."
What to Look For: Seek candidates who understand the process of integrating native modules and can explain the role of the React Native bridge in the communication between JavaScript and native code.
How to Answer: Mention that testing strategies for React Native apps include unit testing, integration testing, and end-to-end testing. Unit testing involves testing individual components, integration testing verifies interactions between components, and end-to-end testing ensures the app functions as expected from a user's perspective.
Sample Answer: "For React Native apps, we use various testing strategies. Unit testing involves testing individual components in isolation to ensure they work correctly. Integration testing verifies that components interact as expected. Additionally, we perform end-to-end testing to ensure the app functions as intended from a user's perspective."
What to Look For: Look for candidates who can describe different testing strategies used in React Native development and their respective purposes.
How to Answer: Explain that React Native provides tools like React Developer Tools and Remote Debugging for debugging JavaScript code. For Android, developers can use adb logcat for viewing logs. For iOS, Xcode's debugger can be used to debug the app on the simulator or a physical device.
Sample Answer: "For debugging React Native apps, we can use tools like React Developer Tools and Remote Debugging to debug JavaScript code. For Android, we can view logs using adb logcat. On the iOS platform, Xcode's debugger allows us to debug the app on the simulator or a physical device."
What to Look For: Seek candidates who are familiar with the debugging tools available for React Native apps on both Android and iOS platforms.
How to Answer: Encourage candidates to share a specific challenge they faced while developing a React Native app. Ask them to describe the actions they took to address the issue, whether it involved seeking help from team members, researching, or finding alternative solutions.
Sample Answer: "During a React Native project, I faced a performance issue when rendering a large list of items. After analyzing the problem, I decided to implement the VirtualizedList component, which significantly improved the list's rendering performance. I also collaborated with team members to review the implementation and gather feedback, ensuring that the solution met the project's requirements."
What to Look For: Look for candidates who can share a real-life challenge they encountered during React Native development and demonstrate problem-solving abilities and collaboration skills in resolving the issue.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
As you approach your React Native job interviews, it's crucial to be well-prepared and confident in showcasing your skills and experiences.
React Native interviews may follow different formats, such as:
Preparing for coding exercises involves:
Behavioral questions assess your soft skills, teamwork, and problem-solving approach. Prepare for these questions by:
Your React Native projects and portfolio are essential aspects of your job interview. Prepare by:
Before your interview, review your React Native projects to ensure you can discuss them confidently:
By preparing thoroughly and showcasing your skills effectively, you'll set yourself up for success in your React Native job interviews.
To further enhance your understanding of React Native, let's explore real-world React Native projects and case studies.
Project Overview: TravelPlanner is a mobile app that helps users plan and organize their trips. It allows users to search for destinations, find places of interest, create itineraries, and collaborate with fellow travelers.
Challenges and Solutions:
Key Takeaways: This project demonstrates skills in integrating APIs, offline data handling, and real-time collaboration using Firebase.
Project Overview: HealthHub is a fitness tracking app that helps users monitor their health and fitness goals. It provides features like tracking workouts, monitoring calorie intake, and setting fitness targets.
Challenges and Solutions:
Key Takeaways: This project demonstrates expertise in UI design, Redux state management, and API integration.
Project Overview: EcoFootprint is an app that helps users track and reduce their environmental impact. It provides features for calculating carbon footprints, setting sustainability goals, and suggesting eco-friendly alternatives.
Challenges and Solutions:
Key Takeaways: This project demonstrates skills in data visualization, local data storage, and internationalization.
React Native continues to evolve, and the React Native community is actively contributing to its growth. As you prepare for your React Native interviews, consider the following trends and future developments:
React Native's development team continuously works on optimizing the framework's performance and improving the Virtual DOM diffing algorithm. Expect future updates to result in faster rendering and improved app performance.
Native modules and integrations will likely play a more significant role in the future of React Native. As developers seek to access more platform-specific features, the community will continue to develop new and improved native modules.
The React Native ecosystem is driven by its vibrant community and the availability of third-party libraries. As more developers contribute to the ecosystem, you can expect a wider range of libraries to address various use cases and challenges.
React Native for the web is gaining traction, allowing developers to build web applications using React Native components. Additionally, Expo, a popular set of tools and services for React Native development, will continue to expand its capabilities, simplifying the development process even further.
As emerging technologies like augmented reality (AR) and machine learning gain momentum, React Native is likely to integrate with these technologies through the use of native modules and third-party libraries.
Congratulations! You've now mastered the essential concepts, advanced topics, and real-world applications of React Native. By following the tips and strategies in this guide, you'll be well-prepared for your React Native job interviews and equipped to tackle real-world React Native development challenges.
Remember to keep honing your skills, stay updated with the latest trends, and continue building exciting React Native projects. Good luck with your React Native journey, and may your coding adventures be successful and rewarding! Happy coding!