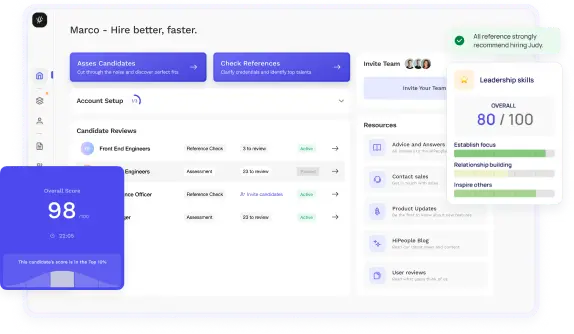
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
If you're preparing for a job interview or seeking to expand your knowledge of React, you've come to the right place. In this comprehensive guide, we'll cover everything you need to know to excel in React-related interviews.
Before diving into the technical aspects, let's understand why React is such a crucial technology in today's web development landscape. React, developed and maintained by Facebook, is an open-source JavaScript library for building user interfaces. Its popularity stems from its simplicity, reusability, and the ability to efficiently update and render components with the help of the Virtual DOM.
The purpose of this guide is to equip you with the knowledge and skills to tackle React interview questions with confidence. Whether you're a beginner or an experienced developer, these insights will prove invaluable in showcasing your expertise during interviews.
To maximize your success, keep the following tips in mind:
Now, let's delve into the core concepts of React and the essential topics you need to master.
React is a JavaScript library that allows developers to build interactive and dynamic user interfaces for web applications. It follows a component-based architecture, breaking the user interface into reusable building blocks called components. React's declarative approach makes it easy to describe how your UI should look based on the application's state.
Comparing React with other popular JavaScript frameworks, such as Angular and Vue.js, can provide insights into React's strengths and use cases.
With a solid understanding of React's fundamentals, let's explore the core concepts in more detail.
The Virtual DOM is a crucial concept in React, providing a performance boost by reducing direct manipulation of the actual DOM. Here's how it works:
Components are the building blocks of a React application. They can be classified into two types: functional components and class components.
Props, short for properties, are the mechanism by which data is passed from parent components to child components. They are immutable and help maintain a unidirectional flow of data.
React components can have state, which represents data that can change over time. State is essential for creating dynamic and interactive user interfaces.
React provides lifecycle methods that allow you to hook into various stages of a component's lifecycle:
Each lifecycle method serves a specific purpose in managing component behavior throughout its existence.
Great! Now that you have a solid foundation of React's core concepts, let's explore React Hooks, a powerful feature introduced in React version 16.8.
React Hooks revolutionized how developers manage state and side effects in functional components. Before Hooks, developers had to use class components to access state and lifecycle methods. With Hooks, you can use stateful logic in functional components, making your code more concise and easier to reason about.
Hooks are functions that let you "hook into" React state and lifecycle features from functional components. They provide a way to use state and other React features without writing a class. Some of the popular React Hooks are:
const [count, setCount] = useState(0);
useEffect(() => {
// Side effect code here
return () => {
// Cleanup code here
};
}, [dependency]);
const value = useContext(MyContext);
const inputRef = useRef(null);
function useCustomHook() {
// Custom logic here
return customValue;
}
React Hooks have several advantages over class components:
Hooks are widely adopted in modern React development, and mastering them is vital for your success in React interviews.
In React, handling events is similar to handling events in native JavaScript, with a few key differences. React's event system uses synthetic events, which are a cross-browser wrapper around the browser's native event.
In React, you attach event handlers to JSX elements using camelCase syntax. For example:
function ButtonComponent() {
const handleClick = () => {
console.log("Button clicked!");
};
return <button onClick={handleClick}>Click Me</button>;
}
React's synthetic events provide a consistent interface across different browsers. They are pooled to improve performance, so accessing event properties asynchronously (e.g., in setTimeout) can lead to unexpected behavior.
Event pooling in React allows the library to reuse synthetic event objects. Thus, you cannot access the event asynchronously because it may be reused by React. If you need to access event properties asynchronously, use event.persist().
function FormComponent() {
const handleSubmit = (event) => {
event.preventDefault();
// Handle form data
};
const handleInputChange = (event) => {
// Handle input changes
};
return (
<form onSubmit={handleSubmit}>
<input type="text" onChange={handleInputChange} />
<button type="submit">Submit</button>
</form>
);
}
function ParentComponent() {
const handleParentClick = () => {
console.log("Parent clicked!");
};
return (
<div onClick={handleParentClick}>
<ChildComponent />
</div>
);
}
function ChildComponent() {
const handleChildClick = (event) => {
event.stopPropagation();
console.log("Child clicked!");
};
return <button onClick={handleChildClick}>Click Me</button>;
}
function ParentComponent() {
const handleButtonClick = (event) => {
if (event.target.tagName === "BUTTON") {
console.log("Button clicked!");
}
};
return (
<div onClick={handleButtonClick}>
<button>Button 1</button>
<button>Button 2</button>
<button>Button 3</button>
</div>
);
}
Understanding event handling in React is vital for building interactive user interfaces. Now, let's explore different techniques for component communication in React.
In React applications, components often need to communicate with one another. Understanding the various methods of component communication is crucial for building scalable and maintainable applications.
Before exploring communication methods, it's essential to distinguish between props and state:
Parent components can pass data down to child components using props.
// Parent Component
function ParentComponent() {
const data = "Hello from Parent!";
return <ChildComponent message={data} />;
}
// Child Component
function ChildComponent(props) {
return <div>{props.message}</div>;
}
Parent components can also pass functions as props to child components, allowing child components to communicate back to their parents.
// Parent Component
function ParentComponent() {
const handleChildClick = () => {
console.log("Child component clicked!");
};
return <ChildComponent onClick={handleChildClick} />;
}
// Child Component
function ChildComponent(props) {
return <button onClick={props.onClick}>Click Me</button>;
}
The Context API allows data to be shared across the component tree without the need to pass props explicitly at each level.
const MyContext = React.createContext();
// Parent Component
function ParentComponent() {
const data = "Hello from Parent!";
return (
<MyContext.Provider value={data}>
<ChildComponent />
</MyContext.Provider>
);
}
// Child Component
function ChildComponent() {
const data = React.useContext(MyContext);
return <div>{data}</div>;
}
Child components can communicate with their parent components by passing callback functions as props.
// Parent Component
function ParentComponent() {
const handleChildClick = () => {
console.log("Child component clicked!");
};
return <ChildComponent onClick={handleChildClick} />;
}
// Child Component
function ChildComponent(props) {
const handleButtonClick = () => {
props.onClick(); // Call the parent's callback function
};
return <button onClick={handleButtonClick}>Click Me</button>;
}
The Context API can also facilitate child-to-parent communication, allowing components to consume data from a context and modify it through provided functions.
Lifting state up refers to moving the state to a common ancestor of two sibling components to share data between them.
function ParentComponent() {
const [count, setCount] = useState(0);
const handleIncrement = () => {
setCount(count + 1);
};
return (
<>
<ChildComponent count={count} />
<SiblingComponent onIncrement={handleIncrement} />
</>
);
}
function ChildComponent(props) {
return <div>Count: {props.count}</div>;
}
function SiblingComponent(props) {
return <button onClick={props.onIncrement}>Increment</button>;
}
Another approach is to create a shared parent component that holds the state and passes it down to the sibling components as props.
function SharedParentComponent() {
const [count, setCount] = useState(0);
const handleIncrement = () => {
setCount(count + 1);
};
return (
<>
<ChildComponent count={count} />
<SiblingComponent count={count} onIncrement={handleIncrement} />
</>
);
}
function ChildComponent(props) {
return <div>Count: {props.count}</div>;
}
function SiblingComponent(props) {
return <button onClick={props.onIncrement}>Increment</button>;
}
For more complex applications, state management libraries like Redux or MobX can be used to centralize the application's state and facilitate communication between components.
In a single-page React application, React Router allows for smooth navigation and routing. It provides a declarative way to define the navigation structure of your application.
React Router is a collection of navigational components that synchronize the UI with the URL. It enables developers to implement client-side routing, ensuring that the user can navigate through different parts of the application without full-page reloads.
To get started with React Router, install the package and define your routes.
npm install react-router-dom
import { BrowserRouter as Router, Route, Link } from "react-router-dom";
function App() {
return (
<Router>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/contact">Contact</Link>
</li>
</ul>
</nav>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Router>
);
}
React Router allows passing parameters to routes, enabling dynamic and personalized content.
// Route configuration
<Route path="/users/:id" component={UserDetail} />
// Accessing route parameters
function UserDetail(props) {
const { id } = props.match.params;
// Fetch user data based on the ID and render the component
}
// Using useLocation Hook
import { useLocation } from "react-router-dom";
function ComponentWithQueryParams() {
const location = useLocation();
const queryParams = new URLSearchParams(location.search);
const searchValue = queryParams.get("search");
// Render content based on searchValue
}
// Using withRouter HOC
import { withRouter } from "react-router-dom";
function ComponentWithQueryParams(props) {
const queryParams = new URLSearchParams(props.location.search);
const searchValue = queryParams.get("search");
// Render content based on searchValue
}
export default withRouter(ComponentWithQueryParams);
Nested routes allow you to create complex page layouts and nested UI structures.
function App() {
return (
<Router>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route path="/users" component={Users} />
</Router>
);
}
function Users() {
return (
<div>
<h2>Users Page</h2>
<ul>
<li>
<Link to="/users/1">User 1</Link>
</li>
<li>
<Link to="/users/2">User 2</Link>
</li>
</ul>
<Route path="/users/:id" component={UserDetail} />
</div>
);
}
React Router is an essential tool for building single-page applications with smooth navigation. Next, we'll explore state management with Redux, a popular choice for managing application state in React.
Redux is a predictable state container for JavaScript applications, providing a centralized store to manage the state of your entire application.
Redux operates on a unidirectional data flow, where data flows in a single direction: from the application state to the UI. The Redux store holds the application state, and actions trigger changes to the state.
const incrementAction = {
type: "INCREMENT",
};
const counterReducer = (state = 0, action) => {
switch (action.type) {
case "INCREMENT":
return state + 1;
default:
return state;
}
};
import { createStore } from "redux";
const store = createStore(counterReducer);
To use Redux in a React application, follow these steps:
npm install redux react-redux
// src/store.js
import { createStore } from "redux";
import rootReducer from "./reducers";
const store = createStore(rootReducer);
export default store;
// src/reducers/index.js
import { combineReducers } from "redux";
import counterReducer from "./counterReducer";
// Other reducers if you have multiple
const rootReducer = combineReducers({
counter: counterReducer,
// Other reducers if you have multiple
});
export default rootReducer;
// src/index.js
import React from "react";
import ReactDOM from "react-dom";
import { Provider } from "react-redux";
import store from "./store";
import App from "./App";
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById("root")
);
import { useSelector, useDispatch } from "react-redux";
function CounterComponent() {
const counter = useSelector((state) => state.counter);
const dispatch = useDispatch();
const handleIncrement = () => {
dispatch({ type: "INCREMENT" });
};
return (
<div>
<p>Count: {counter}</p>
<button onClick={handleIncrement}>Increment</button>
</div>
);
}
Forms are a critical part of web applications, allowing users to input data and interact with the application. In React, handling form input and validation can be done in various ways.
React provides two main approaches for handling form elements: controlled components and uncontrolled components.
Controlled components store form data in the component's state, updating the state whenever the form elements change. This allows React to maintain complete control over the form's data.
function ControlledForm() {
const [username, setUsername] = useState("");
const [email, setEmail] = useState("");
const handleUsernameChange = (event) => {
setUsername(event.target.value);
};
const handleEmailChange = (event) => {
setEmail(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
// Perform form submission using the state data (username and email)
};
return (
<form onSubmit={handleSubmit}>
<label>
Username:
<input type="text" value={username} onChange={handleUsernameChange} />
</label>
<label>
Email:
<input type="email" value={email} onChange={handleEmailChange} />
</label>
<button type="submit">Submit</button>
</form>
);
}
Uncontrolled components rely on the DOM to store form data. React does not manage the form's state directly, and you access the form's data using references.
function UncontrolledForm() {
const usernameRef = useRef();
const emailRef = useRef();
const handleSubmit = (event) => {
event.preventDefault();
// Access form data using refs
const usernameValue = usernameRef.current.value;
const emailValue = emailRef.current.value;
// Perform form submission using the form data
};
return (
<form onSubmit={handleSubmit}>
<label>
Username:
<input type="text" ref={usernameRef} />
</label>
<label>
Email:
<input type="email" ref={emailRef} />
</label>
<button type="submit">Submit</button>
</form>
);
}
Both approaches have their advantages and use cases. Controlled components provide better control and validation of form data, while uncontrolled components can be useful for simple forms.
For more complex forms and advanced validation, consider using form libraries like Formik or react-hook-form. These libraries simplify form handling, provide validation capabilities, and offer a better user experience.
import { Formik, Form, Field, ErrorMessage } from "formik";
function FormikForm() {
const handleSubmit = (values) => {
// Perform form submission using the Formik form data (values)
};
const validate = (values) => {
// Validation logic here
};
return (
<Formik initialValues={{ username: "", email: "" }} onSubmit={handleSubmit} validate={validate}>
<Form>
<label>
Username:
<Field type="text" name="username" />
<ErrorMessage name="username" component="div" />
</label>
<label>
Email:
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
</label>
<button type="submit">Submit</button>
</Form>
</Formik>
);
}
import { useForm, Controller } from "react-hook-form";
function HookForm() {
const { control, handleSubmit } = useForm();
const onSubmit = (data) => {
// Perform form submission using the form data (data)
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<label>
Username:
<Controller
control={control}
name="username"
render={({ field }) => <input type="text" {...field} />}
/>
</label>
<label>
Email:
<Controller
control={control}
name="email"
render={({ field }) => <input type="email" {...field} />}
/>
</label>
<button type="submit">Submit</button>
</form>
);
}
Form libraries offer powerful tools for form handling, including form validation, error handling, and easy integration with form-related components.
Testing is a crucial aspect of software development, ensuring the application functions as intended and remains stable throughout its lifecycle. In React development, testing plays a significant role in maintaining code quality.
React applications can be tested at various levels:
Several testing libraries and tools are widely used in the React community. Here are some of the most popular ones:
// Example Jest test for a React component
import React from "react";
import { render, screen } from "@testing-library/react";
import ButtonComponent from "./ButtonComponent";
test("renders the button correctly", () => {
render(<ButtonComponent />);
const buttonElement = screen.getByRole("button");
expect(buttonElement).toBeInTheDocument();
});
// Example React Testing Library test for a React component
import React from "react";
import { render, screen, fireEvent } from "@testing-library/react";
import FormComponent from "./FormComponent";
test("submits the form with the correct data", () => {
render(<FormComponent />);
const usernameInput = screen.getByLabelText("Username:");
const emailInput = screen.getByLabelText("Email:");
const submitButton = screen.getByRole("button", { name: /submit/i });
fireEvent.change(usernameInput, { target: { value: "testuser" } });
fireEvent.change(emailInput, { target: { value: "test@example.com" } });
fireEvent.click(submitButton);
// Add assertions to test form submission
});
// Example Cypress E2E test
describe("Form Submission", () => {
it("submits the form with the correct data", () => {
cy.visit("/");
cy.get("input[name='username']").type("testuser");
cy.get("input[name='email']").type("test@example.com");
cy.contains("Submit").click();
// Add assertions to test form submission
});
});
Optimizing the performance of a React application is essential for delivering a smooth user experience and improving the application's overall efficiency.
Common performance bottlenecks in React applications include:
React.memo() is a Higher-Order Component (HOC) that optimizes functional components by preventing unnecessary re-renders. It memoizes the component's props and re-renders only when the props change.
import React from "react";
const MyComponent = React.memo((props) => {
// Component logic here
});
The useCallback() and useMemo() Hooks optimize the creation of functions and values in functional components. They memoize the results and prevent re-creation on each render.
import React, { useCallback, useMemo } from "react";
function MyComponent({ data }) {
const expensiveFunction = useCallback(() => {
// Expensive calculations based on data
}, [data]);
const memoizedValue = useMemo(() => {
// Memoized value based on data
}, [data]);
// Component logic using expensiveFunction and memoizedValue
}
Lazy loading is a technique that defers the loading of components until they are needed. It improves the initial loading time of your application.
import React, { lazy, Suspense } from "react";
const LazyComponent = lazy(() => import("./LazyComponent"));
function MyComponent() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
Code splitting is a technique that breaks the application code into smaller chunks, improving performance by loading only the necessary code.
import React from "react";
function MyComponent() {
const handleButtonClick = async () => {
// Dynamically import a module when the button is clicked
const module = await import("./DynamicModule");
// Use the imported module
};
return <button onClick={handleButtonClick}>Load Module</button>;
}
For class components, React.PureComponent and shouldComponentUpdate() can optimize rendering by preventing unnecessary updates.
import React, { PureComponent } from "react";
class MyComponent extends PureComponent {
// Component logic here
}
// or
class MyComponent extends React.Component {
shouldComponentUpdate(nextProps, nextState) {
// Return true or false based on custom logic to decide whether to update
}
// Component logic here
}
To optimize data fetching and reduce network-related performance issues, consider the following techniques:
Error boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI. This prevents the entire application from crashing due to errors in isolated parts of the code.
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// Log the error
}
render() {
if (this.state.hasError) {
return <FallbackUI />;
}
return this.props.children;
}
}
The Context API allows data to be shared across the component tree without the need to pass props explicitly. The useContext() Hook simplifies accessing context values in functional components.
const MyContext = React.createContext();
function ParentComponent() {
return (
<MyContext.Provider value={{ name: "John" }}>
<ChildComponent />
</MyContext.Provider>
);
}
function ChildComponent() {
const contextValue = useContext(MyContext);
return <div>Hello, {contextValue.name}!</div>;
}
Render Props is a design pattern in React where a component passes a function as a prop to its child components. This function allows the child components to render content or provide functionality based on the data received from the parent.
class MouseTracker extends React.Component {
render() {
return (
<div onMouseMove={(event) => this.props.render(event)}>
{/* Render props allow children to access the mouse position */}
{this.props.children}
</div>
);
}
}
function App() {
return (
<MouseTracker render={(event) => <p>Mouse position: {event.clientX}, {event.clientY}</p>}>
{/* Additional components */}
</MouseTracker>
);
}
Higher-Order Components are functions that take a component and return a new component with additional props or behavior. They are a way to share logic between multiple components.
function withLogger(WrappedComponent) {
class WithLogger extends React.Component {
componentDidMount() {
console.log(`Component ${WrappedComponent.name} mounted.`);
}
componentWillUnmount() {
console.log(`Component ${WrappedComponent.name} unmounted.`);
}
render() {
return <WrappedComponent {...this.props} />;
}
}
return WithLogger;
}
const MyComponent = ({ name }) => <div>Hello, {name}!</div>;
const MyComponentWithLogger = withLogger(MyComponent);
React provides various tools to analyze and optimize the performance of your applications. Some commonly used tools include:
How to Answer:Explain that React is a popular JavaScript library used for building user interfaces. Emphasize its component-based architecture, which allows developers to create reusable and modular UI components. Compare React to other frameworks like Angular and Vue, highlighting React's virtual DOM and one-way data flow.
Sample Answer:"React is a JavaScript library for building user interfaces. It follows a component-based approach, where UI elements are broken down into reusable components. React's virtual DOM efficiently updates the actual DOM, leading to better performance. Unlike Angular, React focuses solely on the view layer, making it more lightweight and easier to integrate with other libraries."
What to Look For:Look for candidates who demonstrate a clear understanding of React's core concepts and can articulate its key advantages over other frameworks. A strong answer should highlight React's component-based nature and its role in managing UI rendering efficiently.
How to Answer:Explain that React Hooks are functions that allow functional components to have state and lifecycle features previously available only in class components. Discuss popular Hooks like useState and useEffect, and how they simplify managing state and side effects in functional components.
Sample Answer:"React Hooks are functions that allow functional components to use state and lifecycle features. The useState Hook enables us to add state to functional components without using classes. Meanwhile, the useEffect Hook handles side effects, such as data fetching or subscriptions, in a clean and declarative manner."
What to Look For:Seek candidates who demonstrate a good grasp of React Hooks and can discuss specific Hooks, their purpose, and benefits. Look for examples of how Hooks can simplify code and improve the readability and maintainability of functional components.
How to Answer:Explain that the virtual DOM is a lightweight representation of the actual DOM, and React uses it to improve rendering performance. When the state of a component changes, React updates the virtual DOM, calculates the difference (diffing), and efficiently updates only the necessary parts of the actual DOM.
Sample Answer:"The virtual DOM is a virtual representation of the actual DOM. When the state changes, React calculates the difference between the current virtual DOM and the new one using a process called diffing. It then applies only the necessary changes to the real DOM, reducing unnecessary re-renders and improving performance."
What to Look For:Seek candidates who can clearly explain the purpose and benefits of the virtual DOM. Look for an understanding of how React's virtual DOM helps optimize rendering and minimizes the performance impact of frequent updates.
How to Answer:Clarify that props are used to pass data from a parent component to a child component, while state is used to manage data within a component. Emphasize that props are immutable and passed down from parent to child, while state can be changed within a component using setState.
Sample Answer:"Props are used to pass data from a parent component to a child component. They are read-only and cannot be modified within the child component. On the other hand, state is used to manage data within a component and can be changed using the setState method. State is local to the component and can be updated based on events or user interactions."
What to Look For:Ensure candidates can differentiate between props and state and understand their respective roles in React component communication. Look for examples of how props and state are used in practical scenarios.
How to Answer:Explain that in React, data can be passed from a child component to its parent component using callback functions. The parent component defines a function and passes it to the child component as a prop. The child component can then invoke the function and pass data back to the parent.
Sample Answer:"To pass data from a child to its parent, the parent component defines a callback function and passes it as a prop to the child component. When the child component needs to send data back to the parent, it calls the callback function with the data as an argument."
What to Look For:Look for candidates who can demonstrate a solid understanding of React's unidirectional data flow and can describe how data flows from child to parent. Seek examples of how callback functions facilitate this communication.
How to Answer:Explain that controlled components are form elements whose values are controlled by React state. The value of a controlled component is tied to the state, and any changes to the input trigger updates to the state. Controlled components are essential for React's one-way data flow and form validation.
Sample Answer:"In React, controlled components are form elements whose values are tied to React state. When the user interacts with a controlled component, the state is updated, which, in turn, triggers a re-render of the component with the updated value. Controlled components ensure that the form data is always in sync with the React state and enable straightforward form validation."
What to Look For:Seek candidates who can clearly explain the concept of controlled components and their role in managing form data and validation. Look for examples of how controlled components are implemented in real-world scenarios.
How to Answer:Explain that React Router is a popular routing library for React applications. It enables client-side routing, allowing users to navigate through different parts of the application without full-page reloads. React Router helps create a smooth user experience in single-page applications.
Sample Answer:"React Router is a library used for client-side routing in React applications. It allows us to define routes for different parts of the application, and when users navigate to specific URLs, React Router renders the corresponding components without a full-page refresh. This enables smooth and seamless navigation within single-page applications."
What to Look For:Ensure candidates understand the role of React Router in managing client-side routing and navigation. Look for examples of how React Router is used to create route configurations and handle navigation in React applications.
How to Answer:Explain that route parameters can be defined in the route path using a colon notation, such as /users/:id. React Router then makes these parameters available as props to the rendered component through the match object.
Sample Answer:"To pass route parameters to a React component, we define them in the route path using a colon notation, like /users/:id. When the user navigates to a route with the corresponding path, React Router extracts the parameter value from the URL and makes it available as a prop to the rendered component through the match object."
What to Look For:Look for candidates who can describe how route parameters are defined and accessed in React Router. Seek examples of how route parameters can be used in practical scenarios, such as fetching data based on the parameter value.
How to Answer:Explain that React Router provides a special <Switch> component that allows defining a fallback route for unmatched URLs. Placing a <Route> with no path prop at the end of the <Switch> ensures that it serves as the 404 page, rendering when no other route matches the URL.
Sample Answer:"To handle 404 errors in a React application using React Router, we can use the <Switch> component. Inside the <Switch>, we define our regular routes as usual. At the end of the <Switch>, we add a <Route> with no path prop. This <Route> serves as the fallback route and renders a 404 page when no other route matches the URL."
What to Look For:Ensure candidates understand how to set up a 404 page using React Router and the <Switch> component. Look for examples of how to define routes and place the fallback route correctly within the <Switch>.
How to Answer:Explain that React Context is a feature that allows data to be passed through the component tree without explicit props drilling. Context provides a way to share state across components without passing it through intermediate components manually.
Sample Answer:"React Context is a mechanism for sharing data without having to pass props down through the component tree manually. It consists of two main components: the Context Provider, which wraps the higher-level component tree and holds the shared state, and the Context Consumer, which allows components to access the shared state without explicitly receiving it as props."
What to Look For:Seek candidates who can articulate the purpose and benefits of using React Context for state management. Look for examples of how to create and use Context Providers and Consumers in real-world scenarios.
How to Answer:Explain that React's built-in state management is suitable for small to medium-sized applications with limited shared state. Redux, on the other hand, is a predictable state container that scales well for complex applications with extensive state management requirements.
Sample Answer:"React's built-in state management, using useState and useReducer, is great for managing local component state and small-scale state management needs. It works well when sharing state between parent and child components. However, as an application grows in complexity and requires a central state management solution, Redux becomes a more suitable choice. Redux provides a predictable state container and enables more efficient state management for larger applications."
What to Look For:Seek candidates who can differentiate between React's built-in state management and external state management libraries like Redux. Look for an understanding of the scenarios where one approach might be preferred over the other.
How to Answer:Explain that Redux uses middleware like Redux Thunk or Redux Saga to handle asynchronous actions. With Redux Thunk, actions can return functions instead of plain objects, allowing asynchronous logic such as API calls. Redux Saga uses generator functions to manage complex asynchronous flows.
Sample Answer:"To handle asynchronous actions in Redux, we use middleware like Redux Thunk or Redux Saga. Redux Thunk allows us to dispatch functions as actions, and those functions can perform asynchronous operations, such as making API calls. Redux Saga, on the other hand, uses generator functions to manage complex asynchronous flows, making it suitable for applications with intricate data flow requirements."
What to Look For:Ensure candidates can describe the role of Redux middleware in handling asynchronous actions. Look for examples of how Redux Thunk or Redux Saga can be integrated into Redux stores to manage asynchronous operations effectively.
How to Answer:Explain some common performance optimization techniques, such as using React.memo(), useCallback(), and useMemo() to prevent unnecessary re-renders. Mention code splitting and lazy loading to improve initial loading times, and discuss the importance of optimizing images and reducing network requests.
Sample Answer:"To optimize the performance of React applications, we can use techniques like React.memo() to prevent re-renders of components, useCallback() and useMemo() to memoize functions and values, and lazy loading and code splitting to reduce initial loading times. Additionally, optimizing images, caching API responses, and minimizing network requests can significantly improve performance."
What to Look For:Look for candidates who can demonstrate a solid understanding of performance optimization techniques specific to React applications. Seek examples of how these techniques are applied in real-world scenarios.
How to Answer:Explain that performance bottlenecks in React applications can be identified using browser developer tools, such as Chrome DevTools. Look for unnecessary re-renders, large component trees, and excessive data fetching. Address bottlenecks by optimizing components, using React.memo(), and implementing lazy loading and code splitting.
Sample Answer:"To identify performance bottlenecks in a React application, I would use browser developer tools like Chrome DevTools to analyze rendering times and detect any unnecessary re-renders or inefficient components. I would also check for large component trees that might cause slow rendering. To address the bottlenecks, I would optimize components, use React.memo() to prevent unnecessary re-renders, and implement lazy loading and code splitting to improve the initial loading time."
What to Look For:Seek candidates who can demonstrate their ability to identify and diagnose performance issues in React applications using developer tools. Look for examples of how they have optimized components and improved rendering performance.
How to Answer:Explain that the useContext() Hook is used to consume context values in functional components. It allows components to access shared data without the need for prop drilling. Discuss common use cases, such as accessing user authentication data, theme settings, or language preferences.
Sample Answer:"The useContext() Hook enables functional components to access shared data provided by a Context Provider. It eliminates the need for prop drilling and allows us to consume context values directly within the component. Use cases for useContext() include accessing user authentication data, theme settings, and language preferences throughout the application."
What to Look For:Seek candidates who can demonstrate a clear understanding of how the useContext() Hook works and its benefits in managing shared data. Look for examples of how they have utilized useContext() in real-world scenarios to improve component efficiency and code readability.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Remember, preparation and practice are key to acing your React interviews. Stay confident, keep learning, and showcase your passion for React development. Good luck on your journey to becoming a React expert!
To become a true React expert, consider diving deeper into the following topics:
This has covered the top React interview questions to help candidates prepare effectively and confidently for their React-related interviews. Each question was accompanied by insightful guidance on how to answer, well-crafted sample answers, and valuable insights for hiring managers on what to look for in candidates' responses.
Throughout this guide, we explored essential React fundamentals, such as React's core concepts, the significance of React Hooks, and the role of the virtual DOM in optimizing rendering performance. We delved into React component communication, including the differences between props and state, passing data from child to parent components, and the importance of controlled components in form handling.
Additionally, we covered React Router and navigation, discussing the purpose of React Router in client-side routing and how to handle route parameters and 404 errors effectively. The guide also touched on state management in React, comparing React's built-in state management with external libraries like Redux and explaining how to handle asynchronous actions using middleware.
Furthermore, we explored React performance optimization techniques, including memoization, lazy loading, and code splitting, to ensure applications deliver a seamless user experience. We also provided insights on identifying and addressing performance bottlenecks within React applications.
Finally, the guide offered additional tips for interview success, emphasizing the significance of staying up-to-date with the latest advancements in React through active learning, community engagement, and hands-on projects.
By following this guide, candidates can be well-prepared to demonstrate their expertise in React, while hiring managers can confidently evaluate the skill and potential of React developers seeking to join their teams. We hope this guide serves as a valuable resource for both candidates and hiring managers in navigating React-related interviews and fostering a successful career in web development. Happy interviewing!