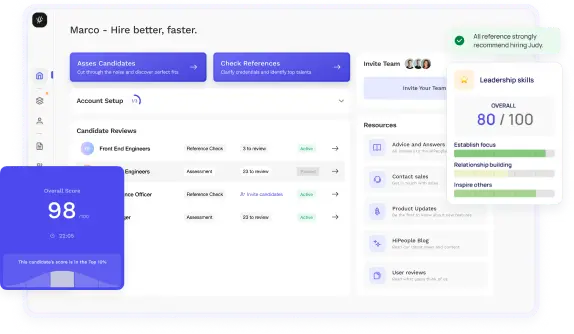
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Whether you are a seasoned Flutter developer looking to ace your next interview or a newcomer to the Flutter ecosystem preparing for your first job interview, this guide is here to help you succeed.
Flutter is an open-source UI software development kit (SDK) created by Google that allows you to build natively compiled applications for mobile, web, and desktop platforms from a single codebase. Flutter uses the Dart programming language, which is known for its performance, productivity, and simplicity.
Flutter follows a reactive and component-based architecture, which revolves around widgets. Widgets are the fundamental building blocks of Flutter UI, and they can be classified into two types: Stateless and Stateful. Stateless widgets represent elements that do not change during the application's lifetime, while Stateful widgets manage state and can be updated dynamically.
Dart is the programming language used in Flutter development. If you're new to Dart, here are some key concepts:
Widgets are the heart of Flutter applications. Here's an overview of widget hierarchy:
Stateless widgets are immutable and cannot be changed during runtime, while stateful widgets can change and are responsible for managing their own state.
Flutter provides a wide range of layout widgets, including:
To get started with Flutter development, follow these steps:
Flutter development is supported on various integrated development environments (IDEs). Two popular choices are Android Studio and Visual Studio Code (VS Code).
Android Studio:
Visual Studio Code (VS Code):
Once you have set up your IDE and installed the Flutter SDK, you'll need to configure emulators or physical devices for testing your Flutter apps.
Emulators:
Physical Devices:
To test on physical devices, you'll need to enable Developer Options on your Android device or join the Apple Developer Program for iOS devices.
In Flutter, creating a beautiful and responsive UI is a breeze, thanks to its rich set of widgets and powerful layout system. Let's dive into the process of building UI in Flutter:
Flutter offers a variety of basic widgets to build UI elements:
// Example: Using basic Flutter widgets
Text(
'Hello, Flutter!',
style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold),
),
Image.asset('assets/image.png'),
Icon(Icons.star),
Flutter supports both Material Design (Android-style) and Cupertino (iOS-style) widgets. This enables you to create platform-specific UIs and maintain a consistent look and feel across different platforms.
// Example: Material Design and Cupertino widgets
MaterialButton(
onPressed: () {
// Add your onPressed logic here
},
child: Text('Click Me'),
),
CupertinoButton(
onPressed: () {
// Add your onPressed logic here
},
child: Text('Click Me'),
),
Flutter allows you to create custom widgets to encapsulate complex UI elements and make them reusable across your app.
// Example: Creating a custom reusable button widget
class MyCustomButton extends StatelessWidget {
final String text;
final VoidCallback onPressed;
MyCustomButton({required this.text, required this.onPressed});
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: onPressed,
child: Text(text),
);
}
}
Theming is an essential aspect of UI design, and Flutter makes it easy to apply consistent styles to your app. Use the ThemeData class to define your app's theme.
// Example: Defining and applying a theme
ThemeData myAppTheme = ThemeData(
primaryColor: Colors.blue,
accentColor: Colors.orange,
fontFamily: 'Roboto',
);
void main() {
runApp(
MaterialApp(
theme: myAppTheme,
home: MyApp(),
),
);
}
State management is crucial in Flutter, especially for building dynamic and responsive applications. There are various state management approaches, each with its strengths and use cases:
Navigating between screens and managing routes is an essential part of building a multi-page Flutter application. Let's explore the key aspects of navigation in Flutter:
In Flutter, navigation is accomplished using a Navigator widget. The Navigator maintains a stack of routes, allowing users to move between different screens.
// Example: Basic navigation in Flutter
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SecondScreen()),
);
Flutter supports two types of routes: Named routes and Anonymous routes.
// Example: Using named routes
MaterialApp(
routes: {
'/': (context) => HomeScreen(),
'/second': (context) => SecondScreen(),
'/third': (context) => ThirdScreen(),
},
);
// Example: Using anonymous routes
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => ModalScreen(),
fullscreenDialog: true,
),
);
Passing data between screens is a common requirement in Flutter applications. You can achieve this using constructors or by using ModalRoute.
// Example: Passing data between screens using constructors
class SecondScreen extends StatelessWidget {
final String data;
SecondScreen({required this.data});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Second Screen'),
),
body: Center(
child: Text(data),
),
);
}
}
// Passing data when navigating to the second screen
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => SecondScreen(data: 'Hello from the first screen!'),
),
);
Flutter allows you to nest navigators within one another to manage complex navigation flows, such as bottom navigation bars or tab bars.
// Example: Nested navigators
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Main Screen'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => NestedScreen(),
),
);
},
child: Text('Go to Nested Screen'),
),
),
);
}
}
Flutter offers flexibility when it comes to choosing an architecture pattern for your app. Selecting the right architecture pattern can greatly impact the maintainability and scalability of your application.
An architecture pattern helps structure your app's codebase, making it easier to manage, test, and maintain.
BLoC (Business Logic Component) is a popular architecture pattern that separates business logic from the UI. It stands for:
The BLoC pattern involves the following components:
Clean Architecture is an architecture pattern that aims to separate concerns by dividing the app into different layers:
Flutter makes it easy to implement Clean Architecture with the use of BLoC or other state management libraries.
Testing is crucial for ensuring the quality and reliability of your Flutter applications. Flutter supports various testing approaches, allowing you to write unit tests, widget tests, and integration tests.
Widget tests focus on testing individual widgets in isolation. You can verify their behavior and rendering.
// Example: Widget testing in Flutter
testWidgets('Testing Widget', (WidgetTester tester) async {
await tester.pumpWidget(MyWidget());
// Add test expectations here
});
WidgetTester is a testing utility provided by Flutter that allows you to interact with widgets and simulate user interactions.
// Example: Widget testing with WidgetTester
testWidgets('Testing Widget Interaction', (WidgetTester tester) async {
await tester.pumpWidget(MyWidget());
// Simulate button tap
await tester.tap(find.byType(ElevatedButton));
// Trigger a frame
await tester.pump();
// Add test expectations here
});
Integration tests evaluate the interaction between multiple widgets, ensuring they work together as expected.
// Example: Integration testing in Flutter
testWidgets('Testing Integration', (WidgetTester tester) async {
await tester.pumpWidget(MyApp());
// Add test expectations here
});
In testing, it's essential to isolate external dependencies, such as APIs or services. Mocking allows you to create fake implementations of these dependencies.
// Example: Mocking in Flutter tests
class MockService extends Mock implements MyService {
// Define mocked methods here
}
In modern app development, working with asynchronous operations, such as fetching data from APIs or reading from databases, is common. Flutter provides various tools and patterns for handling asynchronous tasks effectively.
// Example: Working with Future
Future<int> fetchUserData() {
return Future.delayed(Duration(seconds: 2), () => 42);
}
fetchUserData().then((value) => print(value)); // Output: 42
// Example: Working with Stream
Stream<int> getCounterStream() async* {
for (int i = 1; i <= 5; i++) {
await Future.delayed(Duration(seconds: 1));
yield i;
}
}
getCounterStream().listen((data) => print(data)); // Output: 1, 2, 3, 4, 5
// Example: Using async/await
Future<void> fetchData() async {
final result = await fetchUserData();
print(result); // Output: 42
}
Fetching data from APIs is a common requirement in Flutter apps. To interact with APIs, you can use packages like http, dio, or retrofit.
// Example: Using the 'http' package to fetch data from an API
import 'package:http/http.dart' as http;
Future<String> fetchPost() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts/1'));
if (response.statusCode == 200) {
return response.body;
} else {
throw Exception('Failed to load post');
}
}
Handling errors and exceptions properly is crucial for ensuring the stability and reliability of your Flutter application.
// Example: Error handling in Flutter
try {
final data = await fetchData();
// Process data
} catch (e) {
// Handle the error
print('Error: $e');
}
Flutter is known for its performance, but it's still essential to optimize your app to provide the best user experience. Here are some tips for performance optimization:
Flutter's ecosystem is enriched with numerous plugins and packages that extend the capabilities of your app. Here are some popular ones:
Flutter plugins provide access to native device features, enabling you to integrate native functionalities seamlessly.
// Example: Using 'camera' plugin to capture an image
import 'package:camera/camera.dart';
Future<void> takePicture() async {
final cameras = await availableCameras();
final camera = cameras.first;
final controller = CameraController(camera, ResolutionPreset.medium);
await controller.initialize();
final image = await controller.takePicture();
}
Congratulations! You've built an amazing Flutter app, and now it's time to deploy it to the world. Let's explore how to publish your app on various platforms:
To create an APK (Android Package) file for Android devices or an IPA (iOS App Store Package) file for iOS devices, follow these steps:
# Example: Generating an APK file
flutter build apk
Publishing your app on Google Play Store and Apple App Store involves the following steps:
Setting up Continuous Integration and Delivery (CI/CD) for your Flutter app allows for automated testing, building, and deploying, ensuring a streamlined development process. Popular CI/CD services like Jenkins, CircleCI, Travis CI, and GitHub Actions support Flutter projects.
Flutter is not limited to mobile app development; it extends to web and desktop platforms as well. Let's explore how to leverage Flutter for web and desktop development:
Developing and testing for web and desktop platforms is similar to mobile development:
# Example: Running Flutter web app
flutter run -d chrome
# Example: Running Flutter desktop app on macOS
flutter run -d macos
Firebase is Google's mobile and web application development platform. It provides a suite of tools and services to build scalable and feature-rich apps. Integrating Firebase with Flutter is straightforward:
# Example: Adding Firebase plugins to pubspec.yaml
dependencies:
flutter:
sdk: flutter
firebase_core: ^1.10.0
firebase_auth: ^3.3.4
cloud_firestore: ^2.5.4
Firebase offers various services, but two of the most commonly used ones are authentication and the real-time database.
Firebase provides easy-to-use authentication options, including email/password, Google, Facebook, and more.
// Example: Email/password authentication in Flutter using Firebase
void signInWithEmail(String email, String password) async {
try {
UserCredential userCredential = await FirebaseAuth.instance.signInWithEmailAndPassword(
email: email,
password: password,
);
User? user = userCredential.user;
// Handle successful authentication
} catch (e) {
// Handle authentication errors
}
}
Firebase's real-time database enables real-time synchronization between connected devices. You can store and sync data in JSON format.
// Example: Using Firebase Firestore for real-time data storage
void addUserToFirestore(String name, int age) {
FirebaseFirestore.instance.collection('users').add({
'name': name,
'age': age,
});
}
Flutter provides built-in support for state restoration, allowing your app to preserve its state during interruptions or app lifecycle changes.
State restoration allows Flutter to save and restore the state of your app when it's terminated or resumed. This feature is particularly useful when dealing with long-running tasks or preserving user interactions.
Flutter uses the RestorationMixin and RestorationManager to handle state restoration. Widgets can opt-in for state restoration by implementing the RestorableState mixin.
// Example: State restoration in Flutter
class MyRestorableWidget extends StatefulWidget {
@override
_MyRestorableWidgetState createState() => _MyRestorableWidgetState();
}
class _MyRestorableWidgetState extends State<MyRestorableWidget> with RestorationMixin {
// Add state restoration properties here
late final RestorableInt _counter = RestorableInt(0);
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
// Restore state from oldBucket or initialize it
registerForRestoration(_counter, 'counter');
}
@override
void dispose() {
_counter.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: ElevatedButton(
onPressed: () {
setState(() {
_counter.value++;
});
},
child: Text('Increment Counter'),
),
),
);
}
}
Flutter makes it easy to internationalize your app, allowing it to support multiple languages and locales.
dependencies:
flutter:
sdk: flutter
flutter_localizations: # Add this line
sdk: flutter
intl: ^0.17.0 # Add this line
# Generate localizations code
flutter pub run intl_translation:generate_from_arb --output-dir=lib/l10n lib/l10n/app_localizations.dart lib/l10n/intl_*.arb
// Example: Loading app localizations
void main() async {
await initializeDateFormatting();
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
localizationsDelegates: [
AppLocalizations.delegate, // Add this line
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
],
supportedLocales: AppLocalizations.supportedLocales, // Add this line
home: MyHomePage(),
);
}
}
// Example: Displaying translated text
Text(AppLocalizations.of(context).helloWorld),
To ensure the maintainability and scalability of your Flutter projects, consider the following best practices:
While you've mastered the fundamentals of Flutter, there are advanced concepts and techniques that can take your skills to the next level. Let's explore some of these concepts and suggest further steps to enhance your Flutter expertise:
Flutter provides powerful animation APIs to create smooth and engaging user experiences. Learning how to implement animations can add a touch of elegance to your apps.
// Example: Implicit animation using AnimatedContainer
AnimatedContainer(
duration: Duration(seconds: 1),
height: _isExpanded ? 200 : 100,
color: Colors.blue,
child: Text('Animated Container'),
),
// Example: Explicit animation using AnimationController and Tween
AnimationController controller;
Animation<double> animation;
@override
void initState() {
super.initState();
controller = AnimationController(
duration: Duration(seconds: 2),
vsync: this,
);
animation = Tween(begin: 0.0, end: 1.0).animate(controller);
controller.forward();
}
@override
Widget build(BuildContext context) {
return Opacity(
opacity: animation.value,
child: Text('Fading Text'),
);
}
With the growing interest in Augmented Reality (AR) and Virtual Reality (VR), integrating Flutter with AR/VR technologies can open up new possibilities for app development.
// Example: AR integration using 'ar_flutter_plugin'
ARKitSceneView(
onARKitViewCreated: (controller) {
// Add AR scene here
},
),
Combining Flutter with Machine Learning (ML) libraries can lead to exciting applications in image recognition, natural language processing, and more.
// Example: ML integration using 'tflite_flutter'
final interpreter = await tflite.Interpreter.fromAsset('model.tflite');
final input = Float32List.fromList([0.5, 0.2, 0.1, 0.7]);
final output = Float32List(1 * 1 * 1);
interpreter.run(input, output);
If you're interested in the Internet of Things (IoT), you can connect Flutter with IoT devices using Bluetooth or Wi-Fi.
// Example: IoT communication using 'flutter_blue'
final blue = FlutterBlue.instance;
StreamSubscription<BluetoothDeviceState> subscription;
void listenToDevice(BluetoothDevice device) {
subscription = device.state.listen((state) {
if (state == BluetoothDeviceState.connected) {
// Connected to IoT device
}
});
}
// Start listening to a specific IoT device
final device = await blue.scanForDevices(timeout: Duration(seconds: 4)).first;
listenToDevice(device);
Firebase Extensions are pre-packaged solutions for common Firebase-related tasks. Leveraging Firebase Extensions can simplify and speed up your app development process.
As you advance in your Flutter journey, consider giving back to the Flutter community by:
The Flutter ecosystem is continually evolving, with regular updates and improvements. Stay up-to-date by:
Flutter provides powerful animation APIs that allow you to create smooth and engaging user experiences. Understanding the basics of animations in Flutter is essential for building visually appealing apps.
// Example: AnimationController for a fade animation
AnimationController _controller;
@override
void initState() {
super.initState();
_controller = AnimationController(
vsync: this,
duration: Duration(seconds: 1),
);
}
// Example: CurvedAnimation for smooth transitions
Animation<double> _animation = CurvedAnimation(
parent: _controller,
curve: Curves.easeInOut,
);
While Flutter provides many built-in animations, you can create custom animations to achieve unique effects and interactions.
// Example: Tween animation for scaling
Animation<double> _animation = Tween<double>(begin: 0.0, end: 1.0).animate(_controller);
// Example: Custom transition using AnimatedBuilder
AnimatedBuilder(
animation: _controller,
builder: (context, child) {
return Transform.scale(
scale: _animation.value,
child: child,
);
},
child: MyWidget(),
)
Flutter allows you to capture and respond to user gestures, making your app interactive and responsive.
// Example: GestureDetector for handling taps
GestureDetector(
onTap: () {
// Handle tap gesture
},
child: MyWidget(),
)
// Example: Draggable widget
Draggable(
child: MyWidget(),
feedback: MyWidget(),
childWhenDragging: Opacity(
opacity: 0.5,
child: MyWidget(),
),
)
Flutter allows seamless integration with native code on Android and iOS platforms through platform channels.
// Example: Invoking a method on the native side
static const platform = MethodChannel('com.example.flutter_app/channel_name');
Future<void> _sendMessageToNative(String message) async {
try {
await platform.invokeMethod('method_name', {'message': message});
} catch (e) {
// Handle platform-specific errors
}
}
Flutter provides a way to use native modules and components in your app for platform-specific functionalities.
// Example: Using a camera plugin to access the device's camera
import 'package:camera/camera.dart';
List<CameraDescription> cameras;
Future<void> initCamera() async {
cameras = await availableCameras();
}
void takePicture() async {
final CameraController controller = CameraController(
cameras[0],
ResolutionPreset.medium,
);
await controller.initialize();
await controller.takePicture();
}
Flutter is well-suited for enterprise app development due to its performance and cross-platform capabilities. Scaling Flutter apps for enterprise requires careful planning and implementation.
Security is a top priority for enterprise apps. As you develop Flutter apps for enterprise use, consider the following security measures:
Explain that Flutter is an open-source UI software development toolkit created by Google. It allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase. Emphasize that Flutter differs from other frameworks due to its reactive and declarative approach, which enables hot reload for faster development and a rich set of customizable widgets.
"Flutter is a free and open-source UI toolkit developed by Google, enabling developers to create high-performance, visually appealing applications for multiple platforms from a single codebase. It stands out from other frameworks with its reactive and declarative nature, facilitating hot reload for rapid iteration during development. Additionally, Flutter's extensive widget library allows for fully customizable UI components."
Candidates should showcase a clear understanding of Flutter's unique features and how it stands out from other frameworks. A strong answer should highlight Flutter's capabilities and emphasize its advantages in terms of rapid development and cross-platform capabilities.
Emphasize that in Flutter, everything is a widget, including UI elements, layout, and even the application itself. Discuss how widgets represent the building blocks of a Flutter app's UI and how they are organized in a hierarchical manner to create the app's visual layout. Mention that widgets are lightweight and stateful, making them efficient for rendering and updating.
"In Flutter, widgets are fundamental components that serve as the building blocks of the user interface. They encompass everything, from simple elements like buttons and text to complex layouts and entire app screens. Widgets are organized in a hierarchical manner, forming the widget tree, which defines the app's visual layout. Since widgets are lightweight and stateful, Flutter efficiently renders and updates the UI, contributing to its smooth performance."
Look for candidates who can articulate the significance of widgets and how they define the app's user interface. They should demonstrate an understanding of the widget tree's hierarchical structure and explain how Flutter leverages widgets to provide efficient rendering and state management.
Discuss the various state management options available in Flutter, such as setState, Provider, BLoC (Business Logic Component), Redux, and MobX. Explain that the choice of state management depends on the app's complexity, team preferences, and the need for predictability and scalability.
"In Flutter, we have several state management options, each catering to different app requirements. For simpler applications, we can use the built-in setState method to manage state locally within the widget. For more complex apps, Provider offers a straightforward and efficient solution. BLoC and Redux are great choices for managing state in larger applications with complex data flows. MobX is suitable when we need reactive programming to handle state changes automatically."
Candidates should demonstrate a thorough understanding of different state management approaches in Flutter and when each option is most appropriate. Look for candidates who can justify their choices based on the app's size, complexity, and desired level of predictability.
Describe that BLoC is a state management pattern that separates business logic from UI components. The BLoC pattern involves creating streams of data (using Stream or BehaviorSubject) that emit events and states. These streams are then subscribed to by widgets to receive updates and rebuild accordingly.
"BLoC is a state management pattern that aids in the separation of business logic from the user interface. It involves creating streams of data using Stream or BehaviorSubject, which emit events and states. Widgets can then subscribe to these streams, allowing them to receive updates and rebuild their UI components accordingly. This separation ensures that the UI remains clean and focused on presentation, while the BLoC handles the application's logic and data flow."
A strong response should demonstrate a clear understanding of the BLoC pattern's purpose and its mechanics, such as streams and subscriptions. Candidates should explain how BLoC fosters separation of concerns in Flutter apps.
Explain that Flutter offers two primary methods for navigation: using Navigator.push for pushing a new screen onto the stack and Navigator.pop for removing the current screen from the stack. Describe that pushNamed and pop are commonly used when using named routes and that parameters can be passed between screens.
"In Flutter, we navigate between screens using the Navigator class. To push a new screen onto the stack, we use Navigator.push, and to remove the current screen and go back, we use Navigator.pop. For named routes, we can use pushNamed to navigate based on route names defined in the app's MaterialApp. Additionally, we can pass parameters between screens when navigating to share data."
Candidates should demonstrate a clear understanding of Flutter's navigation system and how to use Navigator.push and Navigator.pop for screen transitions. The ability to explain navigation using both routes and named routes is a plus.
Explain that Flutter allows developers to handle back button presses using the WillPopScope widget. This widget wraps the current screen, and the onWillPop callback is triggered when the back button is pressed. The developer can then decide how to handle the back button press, such as navigating back or showing a confirmation dialog.
"In Flutter, we can handle back button presses using the WillPopScope widget. This widget wraps the current screen and listens to back button presses. When the back button is pressed, the onWillPop callback is invoked, allowing us to define our desired behavior. For instance, we can navigate back or show a confirmation dialog to ask the user if they want to exit the app."
Candidates should demonstrate familiarity with the WillPopScope widget and how to use it to handle back button presses in Flutter. Look for candidates who can effectively explain how to customize the behavior when the back button is pressed.
Explain that both Provider and BLoC are state management patterns, but they differ in their approaches. Provider offers a straightforward and flexible solution for smaller projects with built-in support for ChangeNotifier. BLoC, on the other hand, involves creating streams of data and is suitable for larger applications with complex data flows.
"Provider and BLoC are two popular state management patterns in Flutter. Provider is known for its simplicity and ease of use, making it a great choice for smaller projects. It has built-in support for ChangeNotifier, allowing us to easily manage state and update UI components. BLoC, on the other hand, uses streams to manage data flow, making it more suitable for larger applications with complex state management needs. It encourages a clear separation of concerns, with the business logic handled by the BLoC and UI components subscribing to its streams."
Candidates should demonstrate a clear understanding of the differences between Provider and BLoC, their strengths, and the scenarios where each pattern is most appropriate. Look for candidates who can explain the advantages of using Provider for simplicity and BLoC for more extensive projects with complex data flows.
Explain that Flutter leverages reactive programming through streams and the StreamBuilder widget. Streams are used to emit data changes, and the StreamBuilder widget automatically updates UI components whenever the stream emits new data, facilitating real-time updates.
"Flutter's Reactive Programming is based on the concept of streams, which are used to handle asynchronous data flow. When state changes occur, streams emit new data, and UI components listening to these streams can update their state in real-time. The StreamBuilder widget is a fundamental tool for this approach, as it automatically rebuilds the UI whenever the stream emits new data, ensuring a responsive and reactive user interface."
Candidates should demonstrate a clear understanding of reactive programming in Flutter, highlighting the use of streams and the StreamBuilder widget for handling asynchronous data flow and real-time updates in the UI.
Explain that Flutter supports three types of testing: Unit Testing, Widget Testing, and Integration Testing. Unit Testing involves testing individual functions or methods, Widget Testing verifies the correctness of widgets in isolation, and Integration Testing checks the app's behavior and interactions across multiple widgets and screens.
"Flutter provides three types of testing: Unit Testing, Widget Testing, and Integration Testing. Unit Testing allows us to test individual functions or methods in isolation to ensure they produce the expected output. Widget Testing focuses on verifying the correctness of widgets in isolation, simulating user interactions and widget behavior. Integration Testing, on the other hand, evaluates the interactions and behavior of the entire app, checking the integration of various widgets and screens."
Candidates should be able to articulate the different testing types supported by Flutter and their respective purposes. Look for candidates who can distinguish between Unit Testing, Widget Testing, and Integration Testing and understand when to use each type.
Explain that Widget Tests in Flutter are written using the flutter_test package. These tests allow developers to verify the correctness of UI components in isolation, simulating user interactions and widget behavior. Emphasize that Widget Tests are essential as they ensure that UI components work as intended and help catch UI-related bugs early in the development process.
"In Flutter, Widget Tests are written using the flutter_test package. These tests are designed to check the correctness of UI components in isolation, simulating user interactions and widget behavior. Widget Tests play a crucial role in ensuring that the UI components work as intended and that the app's user interface behaves as expected. They are valuable for detecting and addressing UI-related bugs early in the development process, contributing to a more robust and reliable app."
Candidates should demonstrate familiarity with writing Widget Tests using the flutter_test package and explain the significance of these tests in verifying the correctness of UI components. Look for candidates who can articulate the importance of early bug detection through Widget Testing.
Explain that preparing a Flutter app for release involves several steps, including setting up signing keys, configuring app metadata, and optimizing app performance. Emphasize the importance of testing the app thoroughly before release and following platform-specific guidelines for submission to the Google Play Store and Apple App Store.
"To prepare a Flutter app for release on the Google Play Store and Apple App Store, we need to follow several essential steps. First, we must generate and configure signing keys for each platform to ensure app integrity and security. Next, we need to set up the app's metadata, including app name, description, icons, and screenshots. It is crucial to thoroughly test the app in release mode to identify and fix any issues before submission. Finally, we should adhere to the respective platform's guidelines for app submission to ensure a smooth review process."
Candidates should be well-versed in the steps required to prepare a Flutter app for release on the Google Play Store and Apple App Store. Look for candidates who understand the significance of signing keys, metadata setup, and following platform-specific guidelines.
Explain that CI/CD for Flutter apps involves automating the build, testing, and deployment processes to achieve continuous delivery. Mention that Flutter projects can use popular CI/CD services like Jenkins, CircleCI, Travis CI, and GitHub Actions to automate these workflows.
"Continuous Integration and Delivery (CI/CD) for Flutter apps involves automating the build, testing, and deployment processes to achieve continuous delivery of new features and bug fixes. Popular CI/CD services like Jenkins, CircleCI, Travis CI, and GitHub Actions are compatible with Flutter projects and can be configured to build, test, and deploy the app automatically whenever changes are pushed to the repository. This approach ensures that the app is consistently tested and delivered to users in a timely manner."
Candidates should understand the concept of CI/CD for Flutter apps and how it streamlines the development and deployment process. Look for candidates who can identify well-known CI/CD services that are compatible with Flutter projects.
Explain that Flutter extends beyond mobile platforms and also supports web and desktop development. For web development, Flutter allows developers to compile the same codebase for modern web browsers using Flutter for Web. For desktop development, Flutter supports Windows, macOS, and Linux using the Flutter Desktop Embedding project.
"Flutter is not limited to mobile app development; it extends to web and desktop platforms as well. With Flutter for Web, we can compile the same codebase for modern web browsers, providing a seamless user experience across devices. For desktop development, Flutter supports Windows, macOS, and Linux platforms through the Flutter Desktop Embedding project, enabling us to build native desktop applications using Flutter."
Candidates should showcase an understanding of Flutter's versatility in supporting web and desktop platforms in addition to mobile. Look for candidates who can explain how Flutter enables code reuse across different platforms.
Explain that when developing Flutter apps for web and desktop, it's essential to ensure that the UI is responsive and adaptable to various screen sizes and form factors. Highlight the importance of considering platform-specific guidelines and ensuring that the app's features and interactions align with the user's expectations on each platform.
"When developing Flutter apps for web and desktop, we need to focus on creating a responsive and adaptable UI that can cater to various screen sizes and form factors. It is crucial to consider platform-specific guidelines to provide a consistent user experience across different devices. Additionally, we should pay attention to how certain interactions and features may vary between mobile and desktop users, ensuring that the app's behavior aligns with users' expectations on each platform."
Candidates should demonstrate awareness of the key considerations when developing Flutter apps for web and desktop platforms. Look for candidates who emphasize the importance of a responsive UI and adherence to platform-specific guidelines.
Explain that Flutter can integrate with native code using platform channels. Platform channels allow Flutter to communicate with native code in Android and iOS, enabling access to device-specific functionalities and features. Additionally, Flutter offers packages that provide seamless integration with specific platform features, such as camera access, location services, and more.
"Flutter can seamlessly integrate with native code and platform-specific features using platform channels. Platform channels enable Flutter to communicate with native code in Android and iOS, granting access to device-specific functionalities and APIs. Flutter also provides packages that facilitate easy integration with various platform features, such as camera access, location services, and hardware sensors, ensuring a smooth and native-like user experience."
Candidates should be familiar with Flutter's capability to integrate with native code and platform-specific features using platform channels. Look for candidates who can explain the benefits of accessing native functionalities and how it enhances the app's capabilities.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Preparing for Flutter interviews can significantly increase your chances of success. Here are some tips to help you excel:
During interviews, avoid these common mistakes that can hinder your chances of success:
Behavioral questions assess your soft skills and how you handle various situations. Use the STAR method (Situation, Task, Action, Result) to structure your responses:
Remember to be concise, stay positive, and highlight your ability to collaborate and communicate effectively.
This guide on Flutter Interview Questions has provided a deep dive into the essential topics that candidates may encounter during Flutter interviews. We covered a diverse range of areas, including Flutter fundamentals, state management, navigation, architecture patterns, testing, deployment, and integration with native code. Each question was accompanied by valuable guidance on how to effectively answer it, along with sample answers to serve as reference points for candidates.
For hiring managers and talent acquisition professionals, this guide offers a valuable resource to assess candidates' knowledge and expertise in Flutter development. By using these interview questions, you can gauge a candidate's understanding of key Flutter concepts, problem-solving skills, and familiarity with best practices.
For candidates seeking Flutter opportunities, this guide equips you with the necessary knowledge and strategies to excel in interviews. Understanding these questions, crafting well-structured responses, and showcasing your proficiency in Flutter will undoubtedly enhance your chances of securing a rewarding position in the Flutter development landscape.
Whether you are an interviewer or an interviewee, this guide serves as a valuable tool to navigate the world of Flutter interviews confidently. As Flutter continues to grow in popularity, staying well-prepared and informed is essential to succeed in this dynamic and innovative field. Good luck on your Flutter interview journey, and may you flourish as a skilled Flutter developer!