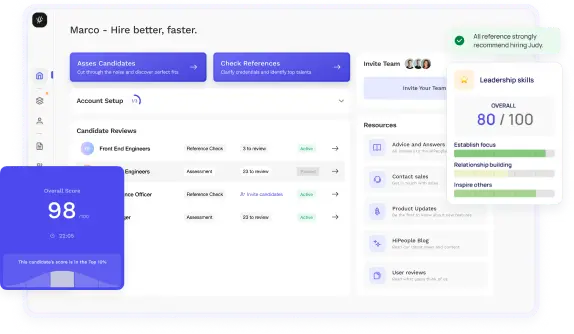
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you preparing for a C++ interview and want to ensure you're ready to tackle any question that comes your way? Look no further! In this guide, we'll equip you with the knowledge and strategies to ace your C++ interview. C++ is a powerful and widely-used programming language, and employers often assess candidates' proficiency in this language during technical interviews. Whether you're a seasoned programmer or just starting your C++ journey, this guide will help you gain a solid understanding of the key concepts and prepare you for the most common interview questions.
Before we dive into the specifics, let's start with some valuable tips to help you optimize your preparation for a C++ interview.
Now that you have some helpful tips to guide your preparation, let's delve into the different sections of C++ that you should focus on.
To succeed in a C++ interview, you need a strong foundation in the language's core concepts. This section will cover the fundamental building blocks of C++, including variables, data types, control structures, functions, arrays, and pointers. Let's explore each of these topics in detail:
In C++, variables serve as containers for storing data. Understanding the different data types and how to declare variables is essential. Here are the key points to grasp:
Operators allow you to perform operations on variables and values. They play a vital role in manipulating data in C++. Here are the key operators to familiarize yourself with:
Control structures allow you to control the flow of your program based on certain conditions or iterations. Mastering these constructs is essential for writing efficient and logic-driven code. Let's explore two essential control structures:
if (condition) {
// code to execute if the condition is true
} else {
// code to execute if the condition is false
}
for (initialization; condition; increment/decrement) {
// code to execute repeatedly
}
while (condition) {
// code to execute while the condition is true
}
do {
// code to execute at least once, then repeatedly while the condition is true
} while (condition);
Functions are reusable blocks of code that perform specific tasks. Understanding function syntax, return types, parameters, and scoping rules is crucial. Here are the key points to grasp:
Arrays and pointers are fundamental concepts in C++. Understanding their usage and interactions is crucial for working with data structures and memory management. Here are the key points to grasp:
Memory management is a critical aspect of programming. Understanding how memory is allocated and deallocated in C++ helps prevent memory leaks and improves performance. Here are the key points to grasp:
Now that you have a solid grasp of the basics, let's move on to understanding the object-oriented programming (OOP) concepts in C++.
Object-oriented programming (OOP) is a programming paradigm that emphasizes the concept of objects and their interactions. In this section, we'll explore the key OOP concepts in C++ and understand how they are implemented. Mastering these concepts is crucial, as they are often tested during C++ interviews.
Object-oriented programming focuses on organizing code into reusable and self-contained objects. These objects encapsulate data and behaviors. Here are the key OOP concepts you need to understand:
Classes and objects are fundamental to OOP in C++. Let's dive into the key aspects of defining classes, creating objects, and interacting with them:
class MyClass {
// class members and methods
};
MyClass myObject; // Object creation without parameters
Inheritance and polymorphism are powerful concepts that enable code reuse and flexibility in C++. Let's explore these concepts in more detail:
class DerivedClass : accessSpecifier BaseClass {
// derived class members and methods
};
class BaseClass {
public:
virtual void virtualFunction() {
// base class implementation
}
};
class DerivedClass : public BaseClass {
public:
void virtualFunction() override {
// derived class implementation
}
};
Encapsulation and abstraction are crucial aspects of object-oriented programming. They help organize code, enhance security, and promote code reusability. Let's explore these concepts further:
Constructors and destructors are special member functions in C++ that play a crucial role in object creation and destruction. Let's explore their purpose and usage:
Operator overloading allows you to redefine the behavior of operators when used with objects of a class. It enables natural and intuitive operations on custom objects. Here's what you need to know about operator overloading:
ReturnType operator+(const ClassName& otherObject) {
// implementation
}
Virtual functions and abstract classes are essential in C++ when dealing with polymorphism and providing a base for derived classes. Let's dive deeper into these concepts:
class AbstractClass {
public:
virtual void pureVirtualFunction() = 0;
};
Now that you have a solid understanding of OOP in C++, let's explore advanced concepts that are commonly tested in C++ interviews.
To truly excel in a C++ interview, you should be familiar with advanced concepts and features of the language. In this section, we'll cover some of these concepts, including templates and generics, exception handling, the Standard Template Library (STL), namespaces, multithreading and concurrency, lambda expressions, smart pointers, move semantics, regular expressions, and type traits and metaprogramming. Let's dive in!
Templates provide a powerful mechanism for generic programming in C++. They allow the creation of functions and classes that can work with different types. Key points to understand:
template <typename T>
void functionName(T parameter) {
// implementation
}
template <typename T>
class ClassName {
// class members and methods
};
Exception handling is an essential aspect of writing robust and reliable C++ code. It allows you to handle and recover from runtime errors gracefully. Key points to understand:
try {
// code that may throw an exception
} catch (ExceptionType& exception) {
// exception handling code
}
The Standard Template Library (STL) is a powerful collection of container classes, algorithms, and utility functions provided by C++. It simplifies complex data structures and common operations. Key components of the STL:
Namespaces provide a way to group related code and avoid naming conflicts. They help organize code and improve code readability. Key points to understand:
namespace MyNamespace {
// code belonging to the namespace
}
Multithreading and concurrency allow programs to perform multiple tasks concurrently, leading to improved performance and responsiveness. C++ provides built-in support for multithreading. Key points to understand:
Lambda expressions provide a concise way to define anonymous functions in C++. They are especially useful in situations where a function is needed as an argument to another function or algorithm. Key points to understand:
[] (parameters) {
// function body
}
Smart pointers are objects that manage the lifetime of dynamically allocated memory. They provide automatic memory management and help prevent common issues like memory leaks and dangling pointers. Key smart pointer types:
Move semantics optimize object copying and enable the efficient transfer of resources between objects. It allows for the transfer of ownership rather than making expensive deep copies. Key points to understand:
Regular expressions are a powerful tool for pattern matching and manipulation of text data. C++ provides support for regular expressions through the <regex> library. Key points to understand:
Type traits and metaprogramming techniques allow compile-time introspection and manipulation of types. They enable the creation of generic code that adapts to different types. Key points to understand:
With a solid understanding of these advanced concepts, you'll be well-prepared to tackle any challenging C++ interview question.
How to Answer: Explain that a pointer is a variable that holds the memory address of another variable, while a reference is an alias or alternate name for an existing variable. Highlight that pointers can be reassigned and can point to null or garbage values, while references must be initialized when declared and cannot be reassigned.
Sample Answer: "In C++, a pointer is a variable that stores the memory address of another variable. It allows indirect access to the value stored at that memory location. On the other hand, a reference is an alias or alternate name for an existing variable. Once a reference is initialized, it cannot be changed to refer to another variable. References are often used for function parameters and provide a convenient way to work with the original variable."
What to Look For: Look for candidates who can clearly explain the difference between pointers and references, highlighting their uses and limitations. Candidates should demonstrate an understanding of how pointers and references are declared and used in C++ code.
How to Answer: Explain that stack memory is used for storing local variables and function call information. Memory allocation and deallocation are handled automatically. In contrast, heap memory is used for dynamic memory allocation. Memory allocation and deallocation must be managed manually using new and delete or smart pointers.
Sample Answer: "In C++, stack memory is used for storing local variables and function call information. Memory allocation and deallocation are handled automatically by the compiler. The lifetime of stack variables is tied to their scope. On the other hand, heap memory is used for dynamic memory allocation. Memory allocation and deallocation must be managed manually using new and delete operators or smart pointers. Heap memory allows for more flexibility in managing memory but requires careful memory management to avoid memory leaks."
What to Look For: Look for candidates who can clearly explain the differences between stack and heap memory allocation, including their uses and implications. Candidates should demonstrate an understanding of the benefits and challenges of each memory allocation method.
How to Answer: Describe encapsulation as the bundling of data and methods within a class, hiding the internal implementation details and exposing only necessary interfaces. Explain that encapsulation helps maintain data integrity, promotes code reusability, and enhances security.
Sample Answer: "In C++, encapsulation is a fundamental OOP concept that involves bundling data and methods within a class. It allows us to hide the internal implementation details of a class and expose only the necessary interfaces to interact with the object. Encapsulation provides several benefits: it helps maintain data integrity by preventing unauthorized access, promotes code reusability through encapsulated objects, and enhances security by hiding implementation details from external entities."
What to Look For: Look for candidates who can provide a clear and concise definition of encapsulation, highlighting its importance in C++. Candidates should demonstrate an understanding of the benefits and rationale behind encapsulation.
How to Answer: Describe inheritance as a mechanism that allows the creation of new classes (derived classes) based on existing classes (base classes). Explain that derived classes inherit the properties and behaviors of the base class, enabling code reuse and hierarchy.
Sample Answer: "In C++, inheritance is a powerful mechanism that allows us to create new classes based on existing classes. A derived class inherits the properties and behaviors of the base class, allowing for code reuse and the creation of class hierarchies. Inheritance promotes the concept of specialization and generalization, where the derived classes can add new functionalities or override existing ones while maintaining the core characteristics inherited from the base class."
What to Look For: Look for candidates who can explain the concept of inheritance clearly, emphasizing the benefits of code reuse and the hierarchical relationship between base and derived classes.
How to Answer: Define polymorphism as the ability of objects of different classes to be treated as objects of a common base class. Explain that polymorphism allows for dynamic binding and the selection of the appropriate method implementation at runtime based on the actual object type. Highlight that polymorphism is achieved through virtual functions and function overriding.
Sample Answer: "In C++, polymorphism refers to the ability of objects of different classes to be treated as objects of a common base class. It allows for dynamic binding, where the appropriate method implementation is selected at runtime based on the actual object type. Polymorphism is achieved through the use of virtual functions and function overriding. By declaring a function as virtual in the base class and providing different implementations in the derived classes, we can achieve polymorphic behavior."
What to Look For: Look for candidates who can provide a clear definition of polymorphism and explain how it is achieved in C++ through virtual functions and function overriding. Candidates should demonstrate an understanding of the benefits and use cases of polymorphism.
How to Answer: Explain that composition and inheritance are two ways to establish relationships between classes in C++. Describe composition as a "has-a" relationship, where one class contains another class as a member. Discuss inheritance as an "is-a" relationship, where a derived class inherits properties and behaviors from a base class.
Sample Answer: "In C++, composition and inheritance are two mechanisms to establish relationships between classes. Composition represents a 'has-a' relationship, where one class contains another class as a member. It allows us to build complex objects by combining simpler objects. Inheritance, on the other hand, represents an 'is-a' relationship, where a derived class inherits properties and behaviors from a base class. It enables code reuse and supports the concept of a hierarchy."
What to Look For: Look for candidates who can clearly explain the difference between composition and inheritance, emphasizing their respective relationships and use cases. Candidates should demonstrate an understanding of when to use composition and when to use inheritance.
How to Answer: Explain that the virtual keyword is used to define a virtual function in C++. Describe virtual functions as functions that can be overridden in derived classes to provide specific implementations. Emphasize that the virtual keyword is used in the base class declaration, and derived classes can choose to override virtual functions.
Sample Answer: "In C++, the virtual keyword is used to declare a virtual function. A virtual function is a function that can be overridden in derived classes to provide specific implementations. When a virtual function is called through a base class pointer or reference, the appropriate derived class implementation is invoked based on the actual object type. The virtual keyword is used in the base class declaration, and derived classes can choose to override the virtual function using the override keyword. Virtual functions are essential when working with polymorphism and allowing derived classes to provide their own implementations."
What to Look For: Look for candidates who can explain the purpose and usage of the virtual keyword in C++. Candidates should demonstrate an understanding of how virtual functions enable polymorphism and the importance of proper function overriding.
How to Answer: Explain that templates allow for generic programming in C++, enabling the creation of functions and classes that can work with different types. Describe function templates and class templates, emphasizing the use of placeholder types and template parameters.
Sample Answer: "In C++, templates are a powerful feature that allows for generic programming. Templates enable the creation of functions and classes that can work with different types. Function templates allow us to define a generic function that can operate on various data types, using placeholder types as function parameters. Class templates enable the creation of generic classes that can define member variables and member functions that work with different types. Templates make it possible to write reusable code that adapts to different data types, improving code maintainability and reducing code duplication."
What to Look For: Look for candidates who can provide a clear and concise definition of templates, highlighting their use in generic programming. Candidates should demonstrate an understanding of both function templates and class templates and how they enable code reuse.
How to Answer: Explain that exception handling is a mechanism used to handle and recover from runtime errors gracefully. Describe try-catch blocks and the roles of the try, catch, and throw keywords in exception handling.
Sample Answer: "In C++, exception handling is a mechanism used to handle and recover from runtime errors in a structured and graceful manner. It allows us to catch and respond to exceptional situations that might occur during program execution. Exception handling is done using try-catch blocks. The code that may potentially throw an exception is enclosed within the try block, and the catch block(s) following it handle the thrown exception. The throw keyword is used to explicitly throw an exception, while the try keyword marks the beginning of the protected code block."
What to Look For: Look for candidates who can explain the purpose and mechanics of exception handling in C++, including the use of try-catch blocks and the throw keyword. Candidates should demonstrate an understanding of how exception handling can improve code robustness and maintainability.
How to Answer: Describe the STL as a collection of container classes, algorithms, and utility functions provided by C++. Explain that the STL simplifies complex data structures and common operations by providing reusable components. Highlight key components such as containers, algorithms, and iterators.
Sample Answer: "The Standard Template Library (STL) in C++ is a powerful collection of container classes, algorithms, and utility functions. It provides a set of reusable components that simplify the implementation of complex data structures and common operations. The STL consists of various container classes, such as vectors, lists, sets, maps, and queues, each offering different features and trade-offs. It also includes a rich set of algorithms, such as sorting, searching, and manipulating elements in containers. The algorithms operate on iterators, which provide a uniform way to traverse elements in containers. By leveraging the STL, developers can save time, improve code quality, and utilize well-established patterns."
What to Look For: Look for candidates who can provide a clear and concise explanation of the STL, emphasizing its role in simplifying data structures and operations. Candidates should demonstrate an understanding of the different components of the STL, including containers, algorithms, and iterators.
How to Answer: Explain that std::vector and std::array are container classes in the STL with different characteristics. Describe std::vector as a dynamically resizable array that provides convenience methods for insertion and deletion. Discuss std::array as a fixed-size array with a static number of elements.
Sample Answer: "In the STL, std::vector and std::array are container classes, but they have different characteristics. std::vector is a dynamically resizable array that allows elements to be efficiently inserted or deleted at the end or anywhere within the container. It automatically manages memory and provides convenience methods like push_back() and pop_back(). On the other hand, std::array represents a fixed-size array with a static number of elements. The size of std::array is determined at compile-time and cannot be changed. It provides a safer alternative to raw arrays by offering bounds-checking and iterators."
What to Look For: Look for candidates who can explain the differences between std::vector and std::array in terms of dynamic resizing and fixed-size characteristics. Candidates should demonstrate an understanding of when to use each container based on the specific requirements of the problem.
How to Answer: Explain that the const keyword is used to declare constant variables or functions that cannot be modified. Describe its role in ensuring immutability, enforcing compile-time constraints, and preventing unintentional modifications.
Sample Answer: "In C++, the const keyword is used to declare constants, which are variables that cannot be modified once initialized. It ensures immutability and helps prevent unintentional modifications to data. The const keyword can also be used with member functions to indicate that the function does not modify the state of the object. It allows for compiler optimizations and enforces compile-time constraints to prevent accidental changes. Additionally, const objects or references can only call const member functions to maintain consistency and avoid potential side effects."
What to Look For: Look for candidates who can explain the role and usage of the const keyword in C++. Candidates should demonstrate an understanding of const-correctness and how it contributes to code readability, safety, and optimization.
How to Answer: Explain that lambdas are anonymous functions in C++ that can be defined inline. Describe their role in providing a concise way to define small, local functions. Emphasize that lambdas capture variables from their enclosing scope and can be used as arguments in function calls or assigned to variables.
Sample Answer: "In C++, lambdas are anonymous functions that can be defined inline within a code block. They provide a concise way to define small, local functions without the need for explicit function declarations. Lambdas can capture variables from their enclosing scope by value or by reference. They are often used as arguments to functions or assigned to variables, allowing for the creation of ad-hoc functions tailored to specific contexts. Lambdas simplify code by eliminating the need for separate named functions and improving code readability, especially for short, one-time-use functions."
What to Look For: Look for candidates who can explain the concept of lambdas in C++ and their benefits, such as code conciseness and improved readability. Candidates should demonstrate an understanding of lambda syntax, variable capture, and appropriate use cases.
How to Answer: Explain that smart pointers are objects that manage the lifetime of dynamically allocated memory. Describe key smart pointer types, such as std::unique_ptr and std::shared_ptr, and how they automate memory deallocation.
Sample Answer: "In C++, smart pointers are objects that provide automatic memory management for dynamically allocated memory. They help prevent memory leaks and improve code safety. Two commonly used smart pointers are std::unique_ptr and std::shared_ptr. std::unique_ptr ensures exclusive ownership of the dynamically allocated object and automatically deletes the object when it goes out of scope. On the other hand, std::shared_ptr allows multiple smart pointers to manage the same object by keeping track of the reference count. It deletes the object when the last shared pointer goes out of scope. Smart pointers simplify memory management by automating deallocation and reducing the risk of memory leaks."
What to Look For: Look for candidates who can provide a clear and concise definition of smart pointers, emphasizing their role in memory management. Candidates should demonstrate an understanding of different smart pointer types and when to use them.
How to Answer: Explain that optimizing C++ code for performance involves several techniques. Highlight the importance of profiling and identifying bottlenecks, avoiding unnecessary operations, utilizing efficient data structures, optimizing loops, and minimizing memory allocations.
Sample Answer: "Optimizing C++ code for performance requires several techniques. First, it's important to profile the code and identify the performance bottlenecks. Then, focus on avoiding unnecessary operations, such as redundant calculations or excessive function calls. Utilizing efficient data structures, such as vectors for random access or lists for frequent insertions/deletions, can improve performance. Optimizing loops by minimizing iterations, reducing function calls within the loop body, and avoiding unnecessary variable modifications can also lead to performance gains. Finally, minimizing memory allocations by using stack memory instead of heap memory and reusing objects can further enhance performance."
What to Look For: Look for candidates who can provide a comprehensive approach to optimizing C++ code for performance. Candidates should demonstrate an understanding of profiling, identifying bottlenecks, and employing various optimization techniques.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
To excel in a C++ interview and showcase your skills as a competent developer, it's important to follow best practices and employ optimization techniques. In this section, we'll cover essential practices that can enhance your coding style, improve performance, and ensure robustness.
Maintaining a consistent and readable coding style is essential for collaboration and code maintainability. Consider the following practices:
Optimizing code for performance is crucial, especially in performance-critical applications. Consider the following techniques to improve performance:
Proper memory management is crucial to prevent memory leaks, improve performance, and avoid undefined behavior. Consider the following best practices:
Effective exception handling enhances code robustness and maintainability. Consider the following best practices:
Debugging and troubleshooting skills are valuable for resolving issues and improving code quality. Consider the following tips:
By following these best practices and optimization techniques, you can write clean, performant, and robust C++ code, impressing interviewers and demonstrating your proficiency as a C++ developer.
To further enhance your preparation for C++ interviews, consider the following tips and resources:
Congratulations! You've now embarked on a journey to become a C++ interview expert. You've learned about essential OOP concepts, advanced C++ features, and best practices for effective coding, performance optimization, and memory management.
By following the outlined guide, practicing interview questions, and exploring additional resources, you'll be well-prepared to tackle any C++ interview challenge that comes your way. Remember to stay confident, demonstrate your thought process, and showcase your passion for C++ programming. Good luck with your interviews, and happy coding!