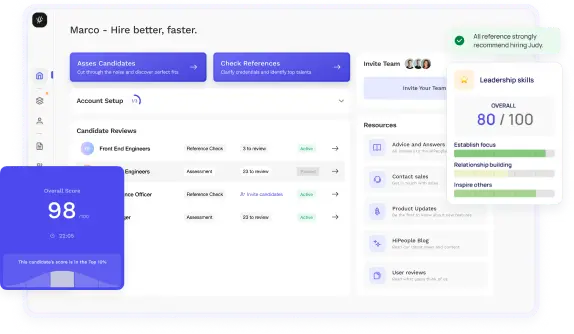
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Angular is a popular open-source JavaScript framework developed by Google. It is widely used for building dynamic web applications with a focus on scalability and modularity. Angular combines declarative templates, dependency injection, end-to-end tooling, and integrated best practices to streamline the development process.
Interviews can feel like a shot in the dark... unless you have the right data to guide you. Why waste time with generic questions and gut-feel decisions when you can unlock precision hiring with HiPeople?
Here’s how HiPeople changes the game:
Ready to transform your hiring process? 🚀 Book a demo today and see how HiPeople makes your interviews smarter, faster, and way more effective!
Angular follows the component-based architecture, where the application is divided into reusable components. These components encapsulate the HTML templates, styles, and business logic, making the code more modular and maintainable. Angular leverages TypeScript, a statically typed superset of JavaScript, to enhance developer productivity and code reliability.
Angular offers a robust framework for building single-page applications (SPAs) and complex enterprise-level web applications. Its comprehensive feature set, including two-way data binding, dependency injection, and powerful templating, enables developers to create rich and interactive user interfaces. Angular's ecosystem provides a wide range of tools, libraries, and community support, making it a popular choice among developers.
Angular has gone through several major releases, each introducing significant enhancements and features. Some of the key versions and their notable features are:
Now that we have a basic understanding of Angular, let's move on to setting up the development environment.
Before you start working with Angular, you need to set up your development environment. Let's go through the steps required to install the necessary tools and create your first Angular project.
Node.js is a JavaScript runtime environment that allows you to run JavaScript on the server-side. Angular relies on Node.js and npm (Node Package Manager) for managing dependencies and running scripts. Follow these steps to install Node.js and npm:
node --version
npm --version
Make sure the version numbers are displayed, indicating a successful installation.
Angular CLI (Command Line Interface) is a powerful tool that simplifies Angular development by providing a command-line interface for creating projects, generating components, running tests, and more. To install Angular CLI, follow these steps:
1. Open a terminal or command prompt.
2. Run the following command to install Angular CLI globally:
npm install -g @angular/cli
3. The -g flag ensures that Angular CLI is installed globally on your system.
4. After the installation is complete, verify that Angular CLI is installed correctly by running the following command:
ng --version
This command should display the version of Angular CLI installed on your system.
Now that you have Node.js, npm, and Angular CLI installed, you're ready to create your first Angular project. Follow these steps:
1. Open a terminal or command prompt and navigate to the directory where you want to create your project.
2. Run the following command to generate a new Angular project:
ng new my-angular-project
Replace my-angular-project with the desired name for your project.
4. Angular CLI will prompt you to choose various options for your project, such as the stylesheet format (CSS, SCSS, etc.) and whether to enable routing. Make your selections based on your preferences and project requirements.
5. Once the project is generated, navigate into the project directory using the following command:
cd my-angular-project
6. Finally, start the development server by running the following command:
ng serve
This command will compile your Angular application and launch the development server. By default, the application will be accessible at http://localhost:4200.
Congratulations! You've successfully set up your Angular development environment and created your first Angular project.
To build a solid foundation in Angular, it's essential to understand its core concepts and fundamental features. We'll cover the key aspects of Angular, including its architecture, TypeScript, modules, components, data binding, and directives.
Angular follows a component-based architecture, where the application is composed of reusable and self-contained building blocks called components. Components are responsible for rendering the UI, handling user interactions, and managing the application's state.
At the heart of Angular's architecture is the concept of a component. A component consists of three main parts:
The component-based architecture of Angular promotes code reusability, separation of concerns, and maintainability.
TypeScript is a statically typed superset of JavaScript that enhances the development experience in Angular. It brings static type checking, class-based object-oriented programming, modules, and more to JavaScript.
To fully utilize Angular's capabilities, it's essential to understand some TypeScript fundamentals:
Understanding these TypeScript fundamentals will enable you to leverage the full power of Angular and write clean and maintainable code.
Modules play a vital role in organizing and structuring an Angular application. They act as containers for related components, services, directives, and other building blocks. Angular modules help encapsulate the functionality of different parts of an application and provide a way to manage their dependencies.
Here are some key points to understand about Angular modules:
By using Angular modules effectively, you can create a modular and scalable application structure.
Components are the building blocks of an Angular application's UI. They encapsulate the UI, handle user interactions, and manage the application's state. Components consist of a TypeScript class, an HTML template, and CSS styles (optional).
To create a component in Angular, you follow these steps:
1. Generate a new component using Angular CLI:
ng generate component my-component
This command will create a new component with the name my-component and generate the necessary files.
2. In the generated component files, you'll find a TypeScript class that represents the component's logic, an HTML template that defines the component's UI, and a CSS file for styling.
3. Customize the component's template and class according to your application's requirements. The template uses Angular's template syntax to bind data and handle events.
Here are some key points to understand about Angular components and templates:
By understanding Angular components and templates, you'll be able to create dynamic and interactive user interfaces for your Angular applications.
Data binding is a powerful feature in Angular that allows you to establish a connection between the component's class and its template. It enables you to update the UI based on changes in the component's data and capture user input to update the component's data.
Angular provides several types of data binding:
Here's an example that demonstrates different types of data binding in Angular:
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<h1>{{ title }}</h1>
<input [value]="name" (input)="name = $event.target.value" />
<button (click)="greet()">Greet</button>
`,
})
export class AppComponent {
title = 'Data Binding Example';
name = '';
greet() {
alert(`Hello, ${this.name}!`);
}
}
In the above example, the title property is bound using interpolation ({{ }}), the input field's value is bound using property binding ([value]="name"), and the button's click event is bound using event binding ((click)="greet()"). When the button is clicked, the greet() method is invoked.
Understanding data binding is crucial for creating dynamic and interactive Angular applications. It allows you to create responsive UIs and handle user input effectively.
Directives are a powerful feature in Angular that allows you to extend the HTML syntax and provide additional functionality to templates. Angular has two types of directives: structural directives and attribute directives.
Structural directives, such as ngIf and ngFor, allow you to add or remove elements from the DOM based on conditions or iterate over a collection of items. Attribute directives, such as ngStyle and ngClass, allow you to dynamically change the style or class of an element.
Here are some commonly used directives in Angular:
Here's an example that demonstrates the usage of some directives in Angular:
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<h1>{{ title }}</h1>
<div *ngIf="showMessage">
<p>Hello, Angular!</p>
</div>
<ul>
<li *ngFor="let item of items">{{ item }}</li>
</ul>
<div [ngStyle]="{ color: 'red', fontWeight: 'bold' }">Styled Text</div>
<button [ngClass]="{ 'active': isActive, 'disabled': !isEnabled }">Submit</button>
<input [(ngModel)]="name" placeholder="Enter your name" />
<p>Welcome, {{ name }}!</p>
`,
})
export class AppComponent {
title = 'Directives Example';
showMessage = true;
items = ['Item 1', 'Item 2', 'Item 3'];
isActive = true;
isEnabled = false;
name = '';
}
In the above example, the ngIf directive is used to conditionally render the div element based on the showMessage property. The ngFor directive iterates over the items array and generates li elements dynamically. The ngStyle directive applies dynamic styles to the div element. The ngClass directive applies dynamic classes to the button element based on the isActive and isEnabled properties. Finally, the ngModel directive establishes two-way binding between the input field and the name property.
By leveraging Angular directives, you can enhance the functionality and interactivity of your application's templates.
Forms play a crucial role in many web applications, and Angular provides robust support for working with forms. Angular offers two approaches to form handling: template-driven forms and reactive forms. Let's explore both approaches and learn how to implement form validation in Angular.
Template-driven forms are based on directives and require less code compared to reactive forms. They rely on Angular's two-way data binding and automatically synchronize the form's data model with the UI controls. Template-driven forms are ideal for simple forms with basic validation requirements.
To create a template-driven form in Angular, follow these steps:
1. Import the FormsModule from @angular/forms in your module:
import { FormsModule } from '@angular/forms';
@NgModule({
imports: [
FormsModule,
// other imports...
],
// other module configurations...
})
export class AppModule { }
2. Use Angular's template-driven form directives in your component's template. For example, use the ngModel directive to bind form controls to component properties:
<form>
<label for="name">Name:</label>
<input type="text" id="name" name="name" [(ngModel)]="name" required>
<button type="submit">Submit</button>
</form>
3. Handle the form submission in your component's class:
import { Component } from '@angular/core';
@Component({
selector: 'app-form',
templateUrl: './form.component.html',
styleUrls: ['./form.component.css']
})
export class FormComponent {
name: string = '';
onSubmit() {
console.log('Form submitted!', this.name);
}
}
In the above example, the ngModel directive is used to bind the name input field to the name property in the component's class. The required attribute is added to enforce the field's validation.
Template-driven forms provide a quick and easy way to implement forms in Angular. However, they have limitations when it comes to complex form scenarios and custom validation requirements.
Reactive forms, also known as model-driven forms, offer a more powerful and flexible approach to working with forms in Angular. Reactive forms are based on reactive programming principles and allow you to manage form state and validation programmatically. They are suitable for complex forms with custom validation requirements.
To create a reactive form in Angular, follow these steps:
1. Import the ReactiveFormsModule from @angular/forms in your module:
import { ReactiveFormsModule } from '@angular/forms';
@NgModule({
imports: [
ReactiveFormsModule,
// other imports...
],
// other module configurations...
})
export class AppModule { }
2. Create a form group in your component's class and define form controls and validators:
import { Component } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
@Component({
selector: 'app-form',
templateUrl: './form.component.html',
styleUrls: ['./form.component.css']
})
export class FormComponent {
form: FormGroup;
constructor(private formBuilder: FormBuilder) {
this.form = this.formBuilder.group({
name: ['', Validators.required],
email: ['', [Validators.required, Validators.email]],
password: ['', [Validators.required, Validators.minLength(6)]],
});
}
onSubmit() {
if (this.form.valid) {
console.log('Form submitted!', this.form.value);
} else {
// Handle form validation errors
}
}
}
3. Bind form controls to UI elements in your component's template:
<form [formGroup]="form" (ngSubmit)="onSubmit()">
<label for="name">Name:</label>
<input type="text" id="name" formControlName="name">
<label for="email">Email:</label>
<input type="email" id="email" formControlName="email">
<label for="password">Password:</label>
<input type="password" id="password" formControlName="password">
<button type="submit">Submit</button>
</form>
In the above example, the ReactiveFormsModule is imported in the module, and the FormGroup and form controls are created using the FormBuilder service. Validators are used to enforce required fields, valid email format, and a minimum password length. The form group is bound to the form attribute using the [formGroup] directive, and each form control is bound using the formControlName directive.
Reactive forms provide fine-grained control over form state and validation. They are ideal for complex form scenarios and custom validation requirements.
Angular provides powerful mechanisms for form validation and error handling. Whether you're using template-driven forms or reactive forms, you can easily implement and customize validation rules, display error messages, and provide a rich user experience.
Here are some techniques for form validation and error handling in Angular:
By leveraging Angular's form validation features, you can ensure data integrity, provide meaningful feedback to users, and create a seamless form experience.
Routing and navigation are essential parts of modern web applications, and Angular provides a powerful routing module to handle navigation between different views. We'll explore Angular's routing module and learn how to set up routes, navigate between views, and guard routes.
To use Angular's routing capabilities, you need to set up the Angular Router module. The Angular Router module enables you to define routes, associate components with routes, and handle navigation between views.
Here's how you can set up Angular routing:
1. Import the RouterModule and Routes from @angular/router in your module:
import { RouterModule, Routes } from '@angular/router';
@NgModule({
imports: [
RouterModule.forRoot(routes),
// other imports...
],
// other module configurations...
})
export class AppModule { }
The forRoot() method is used to configure the router module with the provided routes.
2. Define your routes as an array of route objects in a separate file, such as app-routing.module.ts:
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home.component';
import { AboutComponent } from './about.component';
import { ContactComponent } from './contact.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: 'contact', component: ContactComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule { }
In the above example, three routes are defined: an empty path that maps to the HomeComponent, /about path that maps to the AboutComponent, and /contact path that maps to the ContactComponent.
3. Import and add the AppRoutingModule to your module's imports:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, AppRoutingModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule { }
Now that you have set up Angular routing, you can navigate between views and handle routing-related tasks.
Angular provides the routerLink directive and the Router service to handle navigation between routes. The routerLink directive allows you to generate links to different routes in your templates, while the Router service allows you to programmatically navigate between routes in your component's code.
Here's how you can navigate between routes in Angular:
1. Using the routerLink directive:
<a routerLink="/">Home</a>
<a routerLink="/about">About</a>
<a routerLink="/contact">Contact</a>
In the above example, the routerLink directive is used to generate links to the home, about, and contact routes.
2. Using the Router service:
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-header',
template: `
<button (click)="goToHome()">Home</button>
<button (click)="goToAbout()">About</button>
<button (click)="goToContact()">Contact</button>
`,
})
export class HeaderComponent {
constructor(private router: Router) {}
goToHome() {
this.router.navigate(['/']);
}
goToAbout() {
this.router.navigate(['/about']);
}
goToContact() {
this.router.navigate(['/contact']);
}
}
In the above example, the Router service is injected into the HeaderComponent, and the navigate() method is used to navigate to the desired route.
Angular allows you to pass parameters to routes to make them dynamic. Route parameters allow you to define placeholders in the route path, while query parameters provide a way to pass optional data in the URL.
Here's an example of how to use route parameters and query parameters in Angular:
// app-routing.module.ts
const routes: Routes = [
{ path: 'products', component: ProductsComponent },
{ path: 'products/:id', component: ProductDetailComponent },
{ path: 'search', component: SearchComponent },
];
In the above example, the :id in the /products/:id route is a route parameter that can be accessed in the ProductDetailComponent. For example, if the URL is /products/123, the id parameter will be '123'.
To access route parameters in a component, you can use the ActivatedRoute service:
import { Component } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-product-detail',
template: `
<h1>Product Detail</h1>
<p>Product ID: {{ productId }}</p>
`,
})
export class ProductDetailComponent {
productId: string;
constructor(private route: ActivatedRoute) {
this.productId = this.route.snapshot.paramMap.get('id');
}
}
In the above example, the ActivatedRoute service is injected into the ProductDetailComponent, and the paramMap property is used to access the route parameters.
Query parameters can be added to the URL using the queryParams property of the Router service:
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-search',
template: `
<h1>Search</h1>
<button (click)="search()">Search with Query Params</button>
`,
})
export class SearchComponent {
constructor(private router: Router) {}
search() {
this.router.navigate(['/search'], { queryParams: { q: 'angular', category: 'web' } });
}
}
In the above example, the queryParams property is used to pass the q and category query parameters to the /search route.
Navigating to the /search?q=angular&category=web URL will activate the SearchComponent, and you can access the query parameters using the ActivatedRoute service in a similar way to route parameters.
Route guards allow you to control access to routes based on certain conditions. Angular provides several types of route guards, including CanActivate, CanDeactivate, CanLoad, and Resolve.
Here's an example of how to use the CanActivate route guard:
import { Injectable } from '@angular/core';
import { CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot, UrlTree, Router } from '@angular/router';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class AuthGuard implements CanActivate {
constructor(private router: Router) {}
canActivate(route: ActivatedRouteSnapshot, state: RouterStateSnapshot): Observable<boolean | UrlTree> | Promise<boolean | UrlTree> | boolean | UrlTree {
// Check if the user is authenticated
const isAuthenticated = // ... your authentication logic
if (isAuthenticated) {
return true;
} else {
// Redirect to the login page
return this.router.createUrlTree(['/login'], { queryParams: { returnUrl: state.url } });
}
}
}
In the above example, the AuthGuard implements the CanActivate interface and provides the canActivate method. Inside the canActivate method, you can perform your authentication logic and return true to allow access to the route or redirect to another route using the createUrlTree method.
To use the AuthGuard, you can add it to the route configuration:
const routes: Routes = [
{ path: 'profile', component: ProfileComponent, canActivate: [AuthGuard] },
];
In the above example, the AuthGuard is added to the ProfileComponent route. When a user tries to access the /profile route, the AuthGuard will be invoked, and the route access will be determined based on the canActivate method's return value.
Route guards provide a powerful mechanism to protect routes, authenticate users, and handle access control in your Angular application.
Optimizing the performance of your Angular application is crucial to ensure a smooth and responsive user experience. Let's discuss various techniques and best practices for optimizing Angular application performance.
Angular's change detection mechanism is responsible for detecting changes in the application's state and updating the UI accordingly. By default, Angular uses the "default" change detection strategy, which checks the entire component tree for changes during each change detection cycle.
However, in some cases, this default strategy can lead to unnecessary performance overhead. Angular provides two additional change detection strategies: "OnPush" and "Detached." These strategies allow you to optimize change detection by reducing the number of components checked during each cycle.
Here's an example of how to use the "OnPush" change detection strategy:
import { Component, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-user',
template: `
<h1>{{ name }}</h1>
<!-- Other component content -->
`,
changeDetection: ChangeDetectionStrategy.OnPush,
})
export class UserComponent {
name: string = 'John Doe';
// Other component code...
}
In the above example, the ChangeDetectionStrategy.OnPush is set in the component's metadata. This tells Angular to only run change detection for this component when its inputs change or when an event is triggered. By using the "OnPush" strategy, you can significantly reduce the number of components checked during change detection, resulting in improved performance.
Lazy loading allows you to load modules and their associated components on-demand, rather than loading everything upfront. This can greatly improve the initial load time of your application by only loading the required modules when they are needed.
To enable lazy loading in Angular, you need to configure your routes accordingly. Instead of eagerly loading a module, you can use the loadChildren property to specify the module to be lazy loaded.
Here's an example of lazy loading a module:
const routes: Routes = [
{ path: 'admin', loadChildren: () => import('./admin/admin.module').then(m => m.AdminModule) },
];
In the above example, the admin route is configured to lazy load the AdminModule when the route is accessed. The import() function dynamically loads the module, and the then() method resolves the module and returns it for lazy loading.
By lazy loading modules, you can reduce the initial bundle size and improve the application's startup time.
Angular applications are typically bundled and delivered to the client as JavaScript bundles. The size of these bundles directly impacts the application's load time and performance. Therefore, optimizing the bundle size is crucial for a smooth user experience.
Here are some strategies to optimize the bundle size of an Angular application:
By applying these bundle optimization techniques, you can significantly improve the performance of your Angular application and provide a better user experience.
Server-Side Rendering (SSR) is a technique where the initial rendering of the application is performed on the server and the resulting HTML is sent to the client. This approach can improve the perceived performance of your application by reducing the time-to-first-render and improving search engine optimization (SEO).
Angular provides built-in support for Server-Side Rendering through the Angular Universal project. Angular Universal allows you to render your Angular application on the server using Node.js or other server-side technologies.
To implement Server-Side Rendering in your Angular application, you'll need to follow specific setup and configuration steps outlined in the Angular Universal documentation.
By enabling Server-Side Rendering, you can improve the initial load time, enhance SEO visibility, and provide a better user experience for your Angular application.
Deploying an Angular application involves preparing your application for production and making it available to end-users. There are different deployment strategies for Angular applications.
Before deploying your Angular application, it's crucial to build it for production. The production build optimizes the application's code, removes unnecessary files, and prepares the application for deployment.
To create a production build of your Angular application, you can use the Angular CLI command:
ng build --prod
The --prod flag instructs Angular CLI to build the application with production optimizations.
The production build generates static files in the dist directory, which can be served by a web server.
One of the common deployment strategies for Angular applications is to host the application's static files on a web server. Here are the steps to deploy an Angular application to a web server:
Once deployed, your Angular application will be accessible to users through the configured web server.
Using a Content Delivery Network (CDN) for deploying an Angular application can provide several benefits, including improved global performance, high availability, and scalability.
To deploy an Angular application to a CDN, follow these general steps:
Once the DNS propagation is complete, your Angular application will be served from the CDN, providing improved performance and global availability.
Deploying an Angular application to a cloud platform offers scalability, flexibility, and managed infrastructure. Popular cloud platforms, such as AWS, Google Cloud, and Microsoft Azure, provide services specifically designed for hosting and deploying web applications.
To deploy an Angular application to a cloud platform, the general steps include:
Cloud platforms provide various deployment options and services tailored for different use cases. Consult the documentation of your chosen cloud platform for detailed instructions on deploying an Angular application.
By deploying your Angular application to a cloud platform, you can leverage the platform's scalability, managed infrastructure, and additional services for monitoring, logging, and security.
When answering this question, provide a concise definition of Angular as a TypeScript-based open-source framework for building web applications. Mention its key features, such as declarative templates, dependency injection, and two-way data binding.
"Angular is a popular TypeScript-based open-source framework for building web applications. It allows developers to create dynamic and responsive single-page applications (SPAs) by providing a set of tools, libraries, and patterns. Angular's key features include declarative templates, dependency injection, and two-way data binding."
Look for candidates who demonstrate a clear understanding of Angular's purpose, features, and benefits. They should be able to articulate the advantages of using Angular and explain how it differs from other frameworks.
Explain that AngularJS is the first version of Angular, commonly referred to as "Angular 1," while Angular refers to the newer versions, starting from Angular 2 and beyond. Highlight the major differences, such as language (AngularJS uses JavaScript while Angular uses TypeScript), architecture (Angular follows a component-based architecture), and performance improvements in Angular.
"AngularJS, also known as Angular 1, was the initial version of Angular, while Angular refers to the newer versions like Angular 2, Angular 4, and so on. The key differences include the language used (AngularJS uses JavaScript while Angular uses TypeScript), the architecture (Angular follows a component-based architecture), and significant performance improvements in Angular."
Candidates should demonstrate a clear understanding of the evolution of Angular and the improvements introduced in subsequent versions. Look for awareness of the benefits of using TypeScript and the component-based architecture in Angular.
Explain that an Angular component is a building block of an Angular application. It consists of a TypeScript class that contains the component's logic and data, as well as an associated HTML template that defines the component's structure and appearance. When the component is instantiated, the template is rendered and the component class handles its behavior.
"An Angular component is a fundamental part of an Angular application. It comprises a TypeScript class that contains the component's logic and data, and an associated HTML template that defines the component's structure and appearance. When the component is instantiated, the template is rendered, and the component class handles its behavior."
Candidates should demonstrate a clear understanding of the component-based architecture in Angular and how components work together to build an application's user interface. Look for knowledge of how components encapsulate behavior and data, and how they interact with templates.
Explain that data binding is a mechanism in Angular that allows for synchronization of data between the component class and the HTML template. There are different types of data binding, including interpolation (one-way binding), property binding, event binding, and two-way binding.
"Data binding in Angular is a powerful feature that enables the synchronization of data between the component class and the HTML template. It eliminates the need for manual DOM manipulation. Angular supports various types of data binding, including interpolation (one-way binding), property binding, event binding, and two-way binding."
Candidates should have a solid understanding of data binding and its different types. Look for candidates who can explain how data flows between the component class and the template and understand when to use different types of data binding.
Explain that an Angular service is a reusable code module that provides a specific functionality or performs a set of related tasks. Services play a crucial role in Angular applications by promoting code reusability, separating concerns, and facilitating communication between components through dependency injection.
"An Angular service is a modular unit that encapsulates specific functionality or a set of related tasks. Services are essential in Angular applications as they promote code reusability, separate concerns, and enable effective communication between components through dependency injection."
Candidates should understand the importance of services in Angular applications and how they facilitate code organization, modularity, and separation of concerns. Look for candidates who can articulate the benefits of using services and explain their role in dependency injection.
Explain that dependency injection is a design pattern used in Angular to provide dependencies to a component or service. Instead of creating dependencies within a component, Angular's dependency injection system resolves and injects the required dependencies automatically. This promotes loose coupling and makes components more testable and maintainable.
"Dependency injection is a design pattern employed by Angular to provide dependencies to components or services. Instead of manually creating dependencies within a component, Angular's dependency injection system automatically resolves and injects the required dependencies. This promotes loose coupling, enhances testability, and improves maintainability."
Candidates should demonstrate a clear understanding of dependency injection and its benefits. They should be able to explain how Angular's dependency injection system works and why it is advantageous for building scalable and maintainable applications.
Explain that routing in Angular enables navigation between different views or components within a single-page application. Angular's routing module allows developers to define routes, associate components with routes, and handle navigation between views. The router monitors the browser's URL and activates the appropriate component based on the current route.
"Routing in Angular facilitates navigation between various views or components within a single-page application. Angular's routing module enables developers to define routes, associate components with those routes, and handle navigation between views. The router monitors the browser's URL and activates the appropriate component based on the current route."
Candidates should demonstrate a solid understanding of Angular's routing capabilities and how they enable navigation within an application. Look for candidates who can explain how routes are defined, how components are associated with routes, and how the router handles navigation.
Explain that Angular provides different techniques to pass data between components during navigation, such as route parameters, query parameters, and route state. Route parameters allow for passing data directly in the route's URL, while query parameters provide a way to pass optional data. Route state allows for passing complex data objects that are not reflected in the URL.
"In Angular, there are multiple ways to pass data between components during navigation. Route parameters allow for passing data directly in the route's URL, while query parameters provide a way to pass optional data. Additionally, route state allows for passing complex data objects that are not reflected in the URL."
Candidates should demonstrate familiarity with the different techniques for passing data between components during navigation. They should be able to explain when to use route parameters, query parameters, or route state based on the nature and requirements of the data being passed.
Explain that Angular provides built-in form validation features, including template-driven forms and reactive forms. Template-driven forms rely on directives in the HTML template, while reactive forms use explicit form control objects in the component class. Validators can be applied to form controls to enforce validation rules, and Angular provides various built-in validators.
"Angular offers two approaches for implementing form validation: template-driven forms and reactive forms. Template-driven forms rely on directives in the HTML template to manage form validation. On the other hand, reactive forms use explicit form control objects in the component class. Validators can be applied to form controls to enforce validation rules, and Angular provides a range of built-in validators."
Candidates should demonstrate knowledge of both template-driven forms and reactive forms, and when to use each approach. They should be able to explain how to apply validators to form controls and how to display validation errors to users.
Explain that Angular allows for performing asynchronous form validation by using custom validators or asynchronous validator functions. These validators can make HTTP requests or perform other asynchronous operations to validate form input. The AsyncValidatorFn interface and the async validator are used to implement asynchronous validation.
"In Angular, asynchronous form validation can be achieved by using custom validators or asynchronous validator functions. These validators can make HTTP requests or perform other asynchronous operations to validate form input. The AsyncValidatorFn interface and the async validator are used to implement asynchronous validation."
Candidates should demonstrate an understanding of asynchronous form validation and be able to explain how to implement it using custom validators or asynchronous validator functions. Look for candidates who can discuss the challenges of asynchronous validation and propose strategies for handling them.
Explain that Angular provides testing utilities, such as TestBed and ComponentFixture, to write unit tests for components. These utilities allow for creating the component under test, interacting with its elements and properties, and asserting the expected behavior or state. Angular's testing framework is based on tools like Jasmine and Karma.
"To write unit tests for Angular components, we can leverage Angular's testing utilities, such as TestBed and ComponentFixture. These utilities enable us to create the component under test, interact with its elements and properties, and make assertions on its expected behavior or state. Angular's testing framework is based on popular tools like Jasmine and Karma."
Candidates should demonstrate familiarity with the testing utilities provided by Angular and how to write effective unit tests for components. Look for candidates who understand the concepts of test beds, fixtures, and assertions, and can explain how to set up and tear down tests appropriately.
Explain that Angular applications can be debugged using various techniques. The browser's built-in developer tools, such as the console, network panel, and debugger, are useful for inspecting and debugging Angular applications. Angular also provides additional debugging tools like Augury, which is a Chrome extension specifically designed for debugging Angular applications.
"To debug an Angular application, we can utilize the browser's built-in developer tools, including the console, network panel, and debugger. These tools allow us to inspect the application's state, track network requests, and set breakpoints for debugging. Additionally, Angular provides a helpful tool called Augury, which is a Chrome extension specifically designed for debugging Angular applications."
Candidates should be familiar with common debugging techniques and tools available for Angular applications. Look for candidates who can explain how to use the browser's developer tools effectively and how additional tools like Augury can assist in debugging Angular-specific issues.
Candidates should mention various performance optimization techniques, such as lazy loading modules, tree shaking, AOT (Ahead-of-Time) compilation, code splitting, caching, and reducing the bundle size. They should also discuss the importance of optimizing change detection, using lightweight data structures, and leveraging Angular's built-in performance optimization tools.
"To optimize the performance of an Angular application, we can employ several strategies. These include lazy loading modules to reduce the initial load time, tree shaking to eliminate unused code, AOT (Ahead-of-Time) compilation for faster rendering, code splitting to load only what is needed, caching to reduce network requests, and minimizing the bundle size. Additionally, optimizing change detection, using lightweight data structures, and leveraging Angular's built-in performance optimization tools can significantly improve performance."
Candidates should demonstrate an understanding of the various techniques for optimizing the performance of Angular applications. Look for candidates who can explain the benefits of each optimization strategy and how to apply them effectively.
Candidates should discuss techniques like lazy loading modules, code splitting, and leveraging Angular's built-in performance optimization tools like the Angular CLI's production build. They should also mention strategies like optimizing assets (e.g., compressing images), enabling Gzip compression on the server, and leveraging browser caching.
"To improve the initial load time of an Angular application, we can employ several strategies. Lazy loading modules allows us to load only the required modules on-demand, reducing the initial bundle size. Code splitting enables us to break the code into smaller chunks, loading only what is needed. Additionally, we can optimize assets by compressing images, enable Gzip compression on the server to reduce file size, and leverage browser caching to store static files."
Candidates should demonstrate an understanding of the factors that affect the initial load time of an Angular application and propose effective strategies for improving it. Look for candidates who can discuss trade-offs between different optimization techniques and suggest best practices for optimizing the initial load time.
Candidates should mention best practices like following Angular's style guide, organizing the code using modules, components, and services, using reactive programming with RxJS, keeping components lean and focused, and writing modular and reusable code. They should also discuss the importance of testing, version control, and staying up-to-date with Angular updates.
"When developing Angular applications, it is crucial to follow best practices to ensure maintainability and scalability. Some key practices include adhering to Angular's style guide, organizing the code into modules, components, and services, using reactive programming with RxJS for managing asynchronous operations, keeping components focused and lean, and writing modular and reusable code. It's also important to prioritize testing, leverage version control systems for collaborative development, and stay up-to-date with Angular updates and best practices."
Candidates should demonstrate a strong understanding of best practices for developing Angular applications. Look for candidates who can articulate the importance of each practice and provide specific examples of how they have implemented these practices in their previous projects.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
We have delved into the world of Angular interview questions, equipping you with the knowledge and insights needed to excel in your Angular job interviews. By understanding the fundamental concepts, best practices, and key techniques, you are now prepared to tackle any Angular interview with confidence.
Remember to showcase your understanding of Angular's core principles, demonstrate your problem-solving skills, and provide thoughtful and well-structured answers. With this guide as your resource, you are ready to shine and secure your dream job in the exciting realm of Angular development.