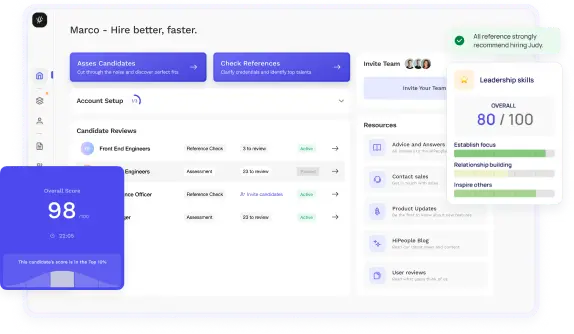
Streamline hiring with effortless screening tools
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to master the world of .NET Core interviews? Whether you're a seasoned developer looking to showcase your expertise or a newcomer eager to land your dream job, navigating the landscape of .NET Core interview questions can be both challenging and rewarding. This comprehensive guide is your key to unlocking the knowledge and strategies needed to ace .NET Core interviews, impress employers, and advance your career in the dynamic field of software development.
.NET Core interviews are a structured assessment process designed to evaluate a candidate's knowledge, skills, and suitability for roles related to .NET Core development. These interviews aim to assess both technical proficiency and soft skills, ensuring that candidates are well-rounded and capable of contributing effectively in .NET Core development roles.
.NET Core interviews cover a broad spectrum of topics, including:
Now that we've defined .NET Core interviews, let's explore why they hold such significance in the world of software development.
.NET Core interviews are not just a formality in the hiring process; they serve a vital purpose for both employers and candidates. Understanding their importance can help you approach these interviews with the right mindset and preparation.
In summary, .NET Core interviews are a critical part of the hiring process, serving as a two-way assessment that ensures both employers and candidates find the perfect match. As you navigate the world of .NET Core interviews, remember that they are an opportunity to showcase your expertise and further your career aspirations.
Now, let's dive deeper into the key concepts and fundamentals that will lay the groundwork for your success in .NET Core interviews.
.NET Core is not just a framework; it's the foundation of modern software development. It's an open-source, cross-platform framework that has revolutionized the way applications are built. Let's explore the core components of .NET Core in more detail:
To excel in .NET Core interviews, you'll need to speak the language of developers. Familiarize yourself with these common terms:
In the world of modern software development, responsiveness is key. Asynchronous programming allows your applications to handle multiple tasks simultaneously, ensuring smooth user experiences. Here's a closer look at this essential concept:
Asynchronous programming involves using the async
and await
keywords to perform non-blocking operations. This is especially crucial in scenarios like web applications, where you don't want to freeze the user interface while waiting for a resource to load.
Want to measure the performance boost of asynchronous code compared to its synchronous counterpart? Use this simple formula:
Speedup = Execution Time of Synchronous Code / Execution Time of Asynchronous Code
Understanding the speedup factor will help you make informed decisions about when and where to use asynchronous programming in your .NET Core applications.
Dependency Injection (DI) isn't just a concept; it's a development practice that can significantly enhance the maintainability and testability of your code. Let's explore DI in more detail:
At its core, DI involves injecting (passing) dependencies into a class rather than creating them within the class. This promotes the Single Responsibility Principle (SRP) and makes your code more modular and easier to test.
Here's a basic DI formula that captures the essence of this pattern:
Dependency = Interface
Service = Class implementing the Interface
Consumer = Class using the Service
By following this pattern, you'll create code that's easier to maintain and test, a crucial skill for any .NET Core developer.
If you're diving into web development with ASP.NET Core, understanding middleware and the middleware pipeline is essential. Let's break it down:
Middleware are components that sit between the web server and your application, processing HTTP requests and responses. Each middleware performs a specific function, such as authentication, logging, or routing.
Imagine the middleware pipeline as a series of processing stages for incoming HTTP requests. Middlewares are executed in the order they're added to the pipeline. This sequential processing allows you to build powerful and flexible web applications.
With a solid grasp of these key concepts and fundamentals, you're well on your way to mastering .NET Core interviews. Now, let's move on to the next section, where we'll explore essential interview preparation strategies.
Now that you have a solid understanding of the key concepts and fundamentals of .NET Core, it's time to focus on the crucial step of preparing for your interviews. Effective preparation can make all the difference in showcasing your skills and landing your dream job in the world of .NET Core development.
Your resume and portfolio are your initial introduction to potential employers. They serve as a window into your skills, experiences, and accomplishments. To create an impressive resume and portfolio:
Understanding the companies you're interviewing with is essential for success. Employers appreciate candidates who demonstrate genuine interest and alignment with their values and goals. Here's how to research potential employers effectively:
Job descriptions are your roadmap to success in interviews. They outline the skills and qualifications employers are seeking in candidates. To understand and leverage job descriptions effectively:
.NET Core interviews come in various formats, each designed to evaluate different aspects of your abilities. Understanding these formats will help you prepare for what lies ahead:
Remember that effective preparation is key to success in all interview formats.
In the world of .NET Core, technical interviews are a crucial part of the hiring process. They assess your proficiency in various areas, from programming concepts to framework-specific knowledge. To ensure you're well-prepared for the challenges that lie ahead, let's explore the key aspects of technical interview preparation.
Data structures and algorithms form the backbone of software development. In .NET Core interviews, you may encounter questions that test your ability to choose the right data structure or devise an efficient algorithm. Here's what you need to know:
C# is the primary language for .NET Core development. You should have a strong grasp of its fundamental concepts:
A deep understanding of the .NET Core framework is essential for technical interviews:
Entity Framework Core (EF Core) is a critical component of .NET Core development, especially when dealing with databases:
If you're venturing into web development, ASP.NET Core is your domain. Here's what you need to prepare:
For those aiming to build web APIs, understanding the intricacies of RESTful API design is crucial:
Unit testing ensures the reliability of your code. Be prepared to discuss:
In .NET Core interviews, you may be asked about design patterns and software architecture principles:
Mastering these technical interview preparation topics will significantly enhance your readiness for .NET Core interviews. In the next section, we'll delve into behavioral interview preparation, focusing on the soft skills and personal qualities that employers seek in candidates.
How to Answer: Explain that .NET Core is a cross-platform, open-source framework for building applications. Highlight the key differences such as platform independence, modularity, and improved performance.
Sample Answer: ".NET Core is an open-source, cross-platform framework designed for developing modern applications. Unlike the traditional .NET Framework, .NET Core is platform-independent, allowing us to run applications on Windows, Linux, and macOS. It's also more modular, allowing us to use only the necessary libraries, and it offers better performance due to its lightweight nature."
What to Look For: Look for a clear understanding of the differences between .NET Core and the traditional .NET Framework, emphasizing the advantages of .NET Core.
How to Answer: Describe Dependency Injection as a technique for achieving Inversion of Control (IoC) in which dependencies are provided to a class rather than created internally. Mention the built-in DI container in .NET Core.
Sample Answer: "Dependency Injection is a design pattern in .NET Core that allows us to provide dependencies (such as services or objects) to a class rather than having the class create them internally. This promotes decoupling and makes our code more maintainable. .NET Core comes with a built-in DI container that manages the injection of dependencies into classes, making it easier to implement IoC."
What to Look For: Evaluate the candidate's understanding of Dependency Injection and its significance in building loosely-coupled and testable applications.
How to Answer: Explain that ASP.NET Core is a cross-platform, high-performance framework for building web applications, while ASP.NET is Windows-centric. Highlight key differences like platform support and improved performance.
Sample Answer: "ASP.NET Core is a cross-platform framework for building web applications, while ASP.NET is primarily Windows-centric. ASP.NET Core is designed to run on Windows, Linux, and macOS, making it more versatile. It also offers better performance and modularity, allowing developers to use only the required components."
What to Look For: Assess the candidate's knowledge of ASP.NET Core and its advantages over ASP.NET, emphasizing platform independence and performance improvements.
How to Answer: Explain that middleware in ASP.NET Core is a pipeline of components that process requests and responses. Emphasize the order of execution and how middleware can be used for tasks like authentication and routing.
Sample Answer: "ASP.NET Core uses middleware to process requests and responses. Middleware components form a pipeline, and each component can perform specific tasks like authentication, routing, or logging. The order of middleware execution is crucial, as it determines the sequence in which these tasks are performed. This allows us to customize the request/response processing flow to suit our application's needs."
What to Look For: Look for a solid understanding of how middleware works in ASP.NET Core and its importance in request/response processing.
How to Answer: Describe Entity Framework Core as an open-source, cross-platform Object-Relational Mapping (ORM) framework and highlight differences like platform support and feature set.
Sample Answer: "Entity Framework Core is an open-source, cross-platform Object-Relational Mapping (ORM) framework for .NET Core applications. It's a lightweight and modular version of Entity Framework, designed to work with .NET Core. Unlike Entity Framework, Entity Framework Core is not tied to Windows and can run on various platforms. It offers improved performance and a simplified feature set."
What to Look For: Assess the candidate's understanding of Entity Framework Core and its benefits over the traditional Entity Framework, particularly its platform independence and performance enhancements.
How to Answer: Describe Code-First Migration as an approach where the database schema is generated based on the application's entity classes and their relationships. Mention the role of migrations in evolving the database schema.
Sample Answer: "Code-First Migration in Entity Framework Core is an approach where we define our entity classes and their relationships in code, and the database schema is generated based on these definitions. Migrations are scripts that capture changes to the database schema over time, allowing us to evolve the database schema as our application changes. It simplifies database versioning and schema management."
What to Look For: Evaluate the candidate's knowledge of Code-First Migration and its role in database schema management within Entity Framework Core.
How to Answer: Explain that ASP.NET Core Web API is a lightweight, cross-platform framework for building RESTful APIs, while ASP.NET Web API is Windows-centric. Highlight platform independence and performance improvements.
Sample Answer: "ASP.NET Core Web API is a cross-platform framework for building RESTful APIs, designed to run on Windows, Linux, and macOS. In contrast, ASP.NET Web API is primarily Windows-centric. ASP.NET Core Web API offers better performance and is more modular, allowing developers to include only the required components for their APIs."
What to Look For: Assess the candidate's understanding of ASP.NET Core Web API and its advantages over ASP.NET Web API, particularly its platform independence and performance enhancements.
How to Answer: Describe the steps to enable CORS in an ASP.NET Core Web API, including configuring CORS policies in the startup.cs file and specifying allowed origins, headers, and methods.
Sample Answer: "To enable CORS in an ASP.NET Core Web API, we need to configure CORS policies in the startup.cs file. We can use the AddCors
method to add a policy that specifies allowed origins, headers, and methods. For example, we can allow requests from 'https://example.com' with specific headers and HTTP methods using WithOrigins
, WithHeaders
, and WithMethods
."
What to Look For: Evaluate the candidate's ability to explain the process of enabling CORS in an ASP.NET Core Web API and their understanding of CORS-related configurations.
How to Answer: Define unit testing as the practice of testing individual units or components of code in isolation to ensure their correctness. Explain its importance in achieving code reliability, maintainability, and bug detection.
Sample Answer: "Unit testing is the practice of testing individual units or components of code in isolation to verify their correctness. It's crucial in .NET Core development because it helps ensure code reliability, maintainability, and early bug detection. Unit tests provide confidence that each piece of code behaves as expected and remains functional when changes are made."
What to Look For: Assess the candidate's understanding of unit testing in .NET Core and its role in maintaining code quality.
How to Answer: Describe the steps to create a unit test using the xUnit testing framework in .NET Core, including writing test methods, using attributes, and running tests.
Sample Answer: "To write a unit test in .NET Core using the xUnit testing framework, we first create a separate test project in our solution. Then, we write test methods and decorate them with attributes like [Fact]
to mark them as tests. We use various assert methods to check if the code under test produces the expected results. Finally, we run the tests using a test runner, such as the xUnit test runner."
What to Look For: Evaluate the candidate's ability to explain the process of writing unit tests in .NET Core using the xUnit testing framework.
How to Answer: List common security vulnerabilities such as SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF), and explain mitigation techniques like input validation, output encoding, and anti-forgery tokens.
Sample Answer: "Common security vulnerabilities in ASP.NET Core applications include SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF). To mitigate these risks, we can use input validation to ensure user inputs are safe, output encoding to prevent XSS attacks, and anti-forgery tokens to protect against CSRF attacks. Additionally, we should follow secure coding practices and stay updated with security patches."
What to Look For: Assess the candidate's knowledge of common security vulnerabilities in ASP.NET Core and their understanding of security best practices for mitigation.
How to Answer: Describe the process of implementing authentication and authorization in an ASP.NET Core application, including configuring authentication providers, defining roles and policies, and using attributes like [Authorize]
.
Sample Answer: "To implement authentication and authorization in an ASP.NET Core application, we can configure authentication providers like Identity or OAuth. We define roles and policies to control access, and we use attributes like [Authorize]
to restrict access to specific controllers or actions. This way, we can ensure that only authenticated users with the appropriate roles or permissions can access protected resources."
What to Look For: Evaluate the candidate's ability to explain the steps for implementing authentication and authorization in an ASP.NET Core application.
How to Answer: List performance optimization techniques such as caching, asynchronous programming, and minimizing database queries. Explain their benefits and when to apply them.
Sample Answer: "To optimize the performance of an ASP.NET Core application, we can use techniques like caching to reduce redundant computations, asynchronous programming to improve responsiveness, and minimizing database queries by using data caching or batch processing. These techniques can significantly enhance the application's speed and scalability, but it's essential to apply them judiciously based on specific use cases."
What to Look For: Assess the candidate's knowledge of performance optimization techniques in ASP.NET Core and their ability to identify situations where these techniques should be applied.
How to Answer: Describe tools and techniques for profiling and diagnosing performance issues in a .NET Core application, including profilers, logging, and performance monitoring tools.
Sample Answer: "Profiling and diagnosing performance issues in a .NET Core application can be done using tools like dotTrace or Visual Studio Profiler. We can also implement extensive logging with frameworks like Serilog or use built-in performance monitoring tools like Application Insights or Prometheus. These tools help identify bottlenecks, memory leaks, and other performance-related problems, allowing us to optimize the application effectively."
What to Look For: Evaluate the candidate's knowledge of performance profiling and diagnostic tools available for .NET Core applications and their ability to use them effectively.
How to Answer: Describe the steps to containerize a .NET Core application using Docker, including creating a Dockerfile, building an image, and running containers.
Sample Answer: "To containerize a .NET Core application using Docker, we start by creating a Dockerfile that specifies the application's environment and dependencies. Then, we use Docker commands to build an image from the Dockerfile and run containers from that image. Docker allows us to package our application along with its dependencies into a portable container, making it easy to deploy and scale across different environments."
What to Look For: Assess the candidate's understanding of containerization using Docker in the context of .NET Core applications and their ability to explain the process effectively.
Looking to ace your next job interview? We've got you covered! Download our free PDF with the top 50 interview questions to prepare comprehensively and confidently. These questions are curated by industry experts to give you the edge you need.
Don't miss out on this opportunity to boost your interview skills. Get your free copy now!
Beyond technical expertise, employers value candidates who possess strong soft skills and the ability to work effectively in teams. In behavioral interviews, you'll have the opportunity to showcase your personal qualities and experiences. Let's dive into the key aspects of behavioral interview preparation.
Behavioral interview questions often revolve around your past experiences and how you've handled various situations. Here are some common behavioral questions you might encounter:
These questions aim to assess your interpersonal skills, problem-solving abilities, and adaptability.
When responding to behavioral questions, it's essential to provide structured and well-articulated answers. The STAR method is a valuable framework to structure your responses:
The STAR method ensures that your responses are structured, concise, and focused on demonstrating your abilities effectively.
In addition to specific behavioral questions, employers are interested in assessing your soft skills and teamwork abilities. These qualities are vital for a harmonious and productive work environment. Here are some essential soft skills and teamwork aspects to consider:
In behavioral interviews, it's crucial to illustrate these soft skills through real-life examples and anecdotes. Employers are not only interested in what you say but also in how you've applied these qualities in practical situations.
By thoroughly preparing for behavioral interviews, you'll be well-equipped to impress employers with your interpersonal skills and ability to contribute positively to their teams. In the next section, we'll explore strategies for preparing for coding challenges, another common aspect of .NET Core interviews.
Coding challenges are a significant component of many .NET Core interviews, especially technical assessments. They assess your ability to write clean, efficient code and solve real-world problems. Let's delve into the strategies and techniques to excel in coding challenges.
Before you face a coding challenge in an interview, it's beneficial to practice on online coding platforms. These platforms offer a vast collection of coding problems, allowing you to hone your problem-solving skills. Some popular online coding platforms include:
To prepare effectively, spend time on these platforms regularly. Solve problems of varying complexity to build your confidence and problem-solving abilities.
Simulating real coding challenges through mock interviews is an excellent way to prepare. You can either practice with a mentor, a fellow developer, or use online platforms that offer mock interviews. Here's how to make the most of mock coding challenges:
By regularly simulating coding challenges, you'll become more comfortable with the process and improve your problem-solving skills.
Time management is critical during coding challenges. You need to balance problem-solving with efficient coding. Here are some time management strategies to consider:
Effective time management ensures that you make the most of the limited time available during a coding challenge.
During coding challenges, you might encounter bugs or realize that your initial solution can be optimized. Here are some debugging and optimization techniques:
Remember that coding challenges aren't just about solving problems; they're an opportunity to demonstrate your coding style, problem-solving approach, and ability to handle real-world scenarios.
By honing your skills on coding platforms, simulating interview conditions, managing your time effectively, and improving your debugging and optimization techniques, you'll be well-prepared to tackle coding challenges in .NET Core interviews.
Interview day can be both exciting and nerve-wracking. Your performance on this day can greatly influence the outcome of your .NET Core interview. Here are some key strategies to ensure you make a positive impression:
The interview doesn't end when you walk out the door. Proper post-interview follow-up can leave a lasting positive impression. Here's what you should consider:
Interviews can be high-pressure situations, and even the most qualified candidates can make mistakes. Being aware of common pitfalls can help you steer clear of them:
By being mindful of these common interview mistakes and implementing effective interview day strategies and post-interview follow-up, you'll be better equipped to navigate the interview process successfully and leave a positive impression on potential employers.
In conclusion, mastering .NET Core interview questions is your pathway to success in the dynamic world of software development. By equipping yourself with technical knowledge, problem-solving skills, and effective interview strategies, you'll confidently tackle any interview scenario and open doors to exciting career opportunities.
Remember, interviews are not just about proving your qualifications; they're also an opportunity to learn and grow. Continuously refine your skills, stay updated with industry trends, and embrace each interview as a chance to showcase your passion and expertise. With dedication and preparation, you'll shine in .NET Core interviews and embark on a fulfilling journey in the world of software development.