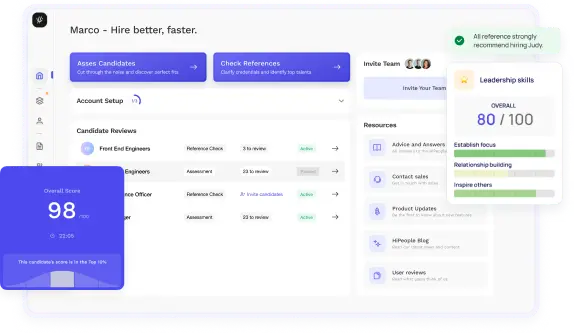
Streamline hiring withour effortless screening.
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to tackle the most challenging aspects of technical interviews head-on? In today's competitive job market, excelling in technical interviews is essential for both employers seeking top talent and candidates striving to stand out. This guide dives deep into the realm of top technical interview questions, equipping you with the knowledge, strategies, and tips needed to navigate these critical assessments successfully. From mastering data structures and algorithms to acing system design and behavioral questions, this guide covers it all, ensuring you're prepared to excel in any technical interview scenario.
Technical interviews are a crucial part of the hiring process for technical roles in fields such as software development, engineering, data science, and IT. Unlike traditional interviews, which may focus more on behavioral questions and general qualifications, technical interviews assess candidates' specific technical skills, problem-solving abilities, and domain knowledge.
Technical interviews play a critical role in identifying candidates who possess the necessary skills and expertise to excel in technical roles. Here are some reasons why technical interviews are important:
While traditional interviews focus on assessing candidates' general qualifications, personality traits, and cultural fit, technical interviews delve deeper into candidates' technical skills and problem-solving abilities. Here are some key differences between technical interviews and traditional interviews:
By understanding the importance of technical interviews and how they differ from traditional interviews, both employers and candidates can better prepare for the unique challenges and opportunities they present.
Preparing for technical interviews is crucial for both candidates and employers to ensure a smooth and effective interview process. Let's delve into each aspect of preparation in detail.
Technical interviews come in various formats, each with its own unique set of challenges and expectations. Understanding these formats can help you tailor your preparation accordingly. Common formats include:
Researching the company and the specific position you're applying for is essential to demonstrate your genuine interest and suitability for the role. Here's what you should focus on:
Reviewing core technical concepts lays the foundation for success in technical interviews. Here are some key areas to focus on:
Practice is key to mastering problem-solving techniques and improving your coding skills. Here's how you can effectively practice:
By understanding common interview formats, researching the company and position, reviewing core technical concepts, and practicing problem-solving techniques, you can significantly increase your chances of success in technical interviews. Remember to stay focused, confident, and adaptable during the interview process.
Technical interviews encompass a wide range of topics, testing candidates' knowledge and problem-solving abilities. Let's explore the top technical interview question categories in detail.
Data structures and algorithms form the backbone of computer science and are extensively tested in technical interviews. Here are the key subcategories:
System design questions assess candidates' ability to design scalable and efficient software systems. Key areas include:
Object-oriented programming concepts are fundamental to software development and are often assessed in technical interviews. Key topics include:
Operating system concepts are essential for understanding how software interacts with hardware. Key areas include:
Database-related questions assess candidates' knowledge of relational databases and SQL. Key areas include:
Behavioral and situational questions assess candidates' soft skills and ability to work effectively in a team. Key areas include:
By familiarizing yourself with these top technical interview question categories and preparing accordingly, you can significantly increase your chances of success in technical interviews. Remember to practice regularly, stay updated with industry trends, and approach each interview with confidence and enthusiasm.
How to Answer: When answering this question, start by defining Big O notation as a mathematical notation used to describe the performance or complexity of an algorithm in terms of its input size. Explain its significance in algorithm analysis, emphasizing how it helps in understanding the worst-case scenario, efficiency, and scalability of algorithms.
Sample Answer: "Big O notation is a mathematical notation used to describe the upper bound of an algorithm's time or space complexity in relation to the size of its input. It helps in evaluating how an algorithm performs as the input size grows. For example, O(1) represents constant time complexity, O(n) linear time complexity, and O(n^2) quadratic time complexity."
What to Look For: Look for candidates who demonstrate a clear understanding of Big O notation and its applications in analyzing algorithm efficiency. Strong candidates should be able to provide examples and discuss the implications of different complexity classes on algorithm performance.
How to Answer: Candidates should discuss the fundamental differences between arrays and linked lists, such as memory allocation, access time, and insertion/deletion operations. They should also explain scenarios where one data structure is preferred over the other based on specific requirements or performance considerations.
Sample Answer: "Arrays allocate contiguous memory locations, allowing for fast random access but have fixed size. Linked lists use non-contiguous memory and offer efficient insertion and deletion but slower access. Arrays are preferable for direct access scenarios, while linked lists are better suited for frequent insertions and deletions."
What to Look For: Seek candidates who can articulate the pros and cons of arrays and linked lists and provide clear reasoning for choosing one over the other in different situations. Strong answers will demonstrate an understanding of trade-offs and consideration of performance implications.
How to Answer: Candidates should outline the key components of a URL shortening service, including URL encoding, storage of mappings, and handling redirections. They should discuss scalability, fault tolerance, and potential challenges such as link collisions and spam prevention.
Sample Answer: "I would design the service with a front-end for URL submission, a URL encoder to generate short links, and a database to store mappings between short and original URLs. To handle scalability, I'd employ sharding or partitioning. Implementing rate limiting and CAPTCHA can help prevent spam and abuse."
What to Look For: Look for candidates who demonstrate a systematic approach to system design, considering scalability, performance, and reliability aspects. Strong answers will include strategies for handling potential challenges and ensuring the security of the service.
How to Answer: Candidates should define load balancing as the process of distributing incoming network traffic across multiple servers to optimize resource utilization, reliability, and response time. They should discuss various load balancing algorithms like Round Robin, Least Connection, and Weighted Round Robin, along with their advantages and use cases.
Sample Answer: "Load balancing ensures even distribution of traffic among servers, improving performance and reliability. Round Robin assigns requests sequentially, while Least Connection directs traffic to the server with the fewest active connections. Weighted Round Robin allows assigning different weights to servers based on their capacity."
What to Look For: Seek candidates who can explain load balancing principles and demonstrate knowledge of different algorithms and their suitability for various scenarios. Look for examples illustrating the understanding of trade-offs between algorithms.
How to Answer: Candidates should discuss the four pillars: encapsulation, inheritance, polymorphism, and abstraction. They should explain each concept and its significance in OOP, along with examples illustrating their use.
Sample Answer: "Encapsulation bundles data and methods within a class, protecting data integrity. Inheritance enables a class to inherit properties and behavior from another class, promoting code reuse. Polymorphism allows objects of different types to be treated uniformly, enhancing flexibility. Abstraction focuses on hiding implementation details, exposing only necessary interfaces."
What to Look For: Look for candidates who can articulate the four pillars of OOP and provide clear explanations along with relevant examples. Strong answers will demonstrate an understanding of how these principles contribute to code organization, reuse, and maintainability.
How to Answer: Candidates should explain the concepts of composition and inheritance and highlight their differences. They should discuss when to prefer one over the other and the advantages and disadvantages of each approach.
Sample Answer: "Composition involves creating objects within another class to represent parts or components, promoting flexibility and modularity. Inheritance allows a class to inherit properties and behavior from another class, facilitating code reuse but potentially leading to tight coupling and hierarchies."
What to Look For: Seek candidates who can provide clear distinctions between composition and inheritance and discuss the trade-offs associated with each approach. Strong answers will demonstrate an understanding of design principles and considerations in object-oriented programming.
How to Answer: Candidates should define ACID (Atomicity, Consistency, Isolation, Durability) and explain each component's role in ensuring transactional integrity and reliability. They should provide examples to illustrate how ACID properties are maintained in database transactions.
Sample Answer: "ACID is a set of properties that guarantee database transactions are processed reliably. Atomicity ensures that transactions are treated as indivisible units, either fully completed or aborted. Consistency ensures that the database remains in a valid state before and after transactions. Isolation ensures that concurrent transactions do not interfere with each other. Durability ensures that committed transactions persist even in the event of system failures."
What to Look For: Look for candidates who demonstrate a clear understanding of ACID properties and their importance in database management. Strong answers will include real-world examples and discuss how ACID compliance impacts transactional reliability.
How to Answer: Candidates should discuss the differences between SQL (relational) and NoSQL (non-relational) databases, including data model, scalability, schema flexibility, and use cases. They should provide insights into when each type of database is suitable based on project requirements.
Sample Answer: "SQL databases use a structured schema and are ideal for complex queries and transactions requiring ACID compliance. NoSQL databases offer schema flexibility and horizontal scalability, making them suitable for handling large volumes of unstructured data and distributed systems. SQL is preferable for applications requiring strong consistency and relational data modeling, while NoSQL is suitable for scenarios demanding high availability and scalability."
What to Look For: Seek candidates who can articulate the differences between SQL and NoSQL databases and provide rationale for choosing one over the other based on project requirements. Strong answers will demonstrate an understanding of trade-offs and considerations in database selection.
How to Answer: Candidates should define the Agile methodology, emphasizing iterative development, collaboration, and flexibility in responding to change. They should compare Agile with the traditional waterfall model, highlighting key differences in approach, project management, and adaptability.
Sample Answer: "Agile is an iterative software development approach that prioritizes customer collaboration, adaptive planning, and rapid response to change. Unlike the waterfall model, which follows a sequential process of requirements gathering, design, development, testing, and deployment, Agile focuses on incremental delivery, continuous feedback, and self-organizing teams."
What to Look For: Look for candidates who can explain the principles and practices of Agile methodology and contrast it with traditional waterfall development. Strong answers will include examples of Agile practices such as Scrum, Kanban, or Extreme Programming (XP).
How to Answer: Candidates should outline the Scrum framework, including roles (Scrum Master, Product Owner, Development Team), artifacts (Product Backlog, Sprint Backlog, Increment), and events (Sprint Planning, Daily Standup, Sprint Review, Sprint Retrospective). They should explain how Scrum promotes collaboration, transparency, and iterative delivery.
Sample Answer: "Scrum is an Agile framework for managing complex software development projects. It includes three key roles: Scrum Master, responsible for facilitating Scrum events and removing impediments; Product Owner, accountable for maximizing product value and managing the product backlog; Development Team, self-organizing cross-functional individuals responsible for delivering increments of product functionality."
What to Look For: Seek candidates who can provide a comprehensive overview of the Scrum framework, including its roles, artifacts, and events. Strong answers will demonstrate an understanding of how Scrum principles drive team collaboration, continuous improvement, and adaptability.
How to Answer: Candidates should define client-side rendering and server-side rendering and discuss their respective advantages and disadvantages. They should explain when each rendering method is preferred and how they impact performance, SEO, and user experience.
Sample Answer: "Client-side rendering involves generating web content dynamically in the user's browser using JavaScript frameworks like React or Angular. Server-side rendering, on the other hand, renders web content on the server before sending it to the client's browser. Client-side rendering offers better interactivity and responsiveness, while server-side rendering improves initial page load time and SEO."
What to Look For: Look for candidates who can articulate the differences between client-side and server-side rendering and discuss their implications for web development. Strong answers will include considerations of performance optimization and user experience.
How to Answer: Candidates should discuss the features of CSS Grid Layout, such as defining rows and columns, grid lines, grid areas, and alignment properties. They should compare CSS Grid Layout with CSS Flexbox, highlighting their strengths, use cases, and when to choose one over the other.
Sample Answer: "CSS Grid Layout is a two-dimensional layout system that allows precise control over rows and columns, making it ideal for complex grid-based designs. It enables the creation of grid tracks, grid areas, and alignment of content within the grid. CSS Flexbox, on the other hand, is a one-dimensional layout model primarily focused on arranging items along a single axis. Flexbox is better suited for building flexible and responsive layouts, while CSS Grid Layout excels in creating grid-based designs."
What to Look For: Seek candidates who demonstrate a solid understanding of CSS Grid Layout features and its differences from CSS Flexbox. Strong answers will include practical examples and considerations for layout design and responsiveness.
How to Answer: Candidates should define Continuous Integration (CI) as the practice of regularly merging code changes into a shared repository, followed by automated builds and tests. Continuous Deployment (CD) extends CI by automatically deploying code changes to production environments. They should explain how CI/CD pipelines streamline development workflows, improve code quality, and enable faster and more frequent releases.
Sample Answer: "Continuous Integration (CI) involves integrating code changes into a shared repository frequently, followed by automated build and test processes to detect and fix integration errors early. Continuous Deployment (CD) goes a step further by automatically deploying code changes to production environments after passing CI tests. CI/CD pipelines automate the software delivery process, enabling teams to deliver high-quality software faster, with reduced manual intervention and risk."
What to Look For: Look for candidates who can explain the principles and benefits of CI/CD practices in DevOps and how they contribute to accelerating software delivery and improving overall development efficiency.
How to Answer: Candidates should explain the concepts of IaaS, PaaS, and SaaS and distinguish between them based on the level of abstraction and responsibility shared between the service provider and the user. They should provide examples of each service model and discuss their benefits and use cases.
Sample Answer: "Infrastructure as a Service (IaaS) provides virtualized computing resources over the internet, including virtual machines, storage, and networking. Platform as a Service (PaaS) offers a platform with development tools and runtime environments for building, deploying, and managing applications. Software as a Service (SaaS) delivers software applications over the internet on a subscription basis, eliminating the need for users to manage underlying infrastructure or software."
What to Look For: Look for candidates who can clearly explain the differences between IaaS, PaaS, and SaaS and provide examples illustrating their respective service models and benefits.
How to Answer: Candidates should discuss security challenges in cloud computing, such as data breaches, identity and access management, and compliance requirements. They should explain best practices for securing cloud environments, including encryption, network security, access control, and monitoring.
Sample Answer: "Securing cloud environments involves implementing a multi-layered approach to protect data, applications, and infrastructure. Best practices include encrypting data at rest and in transit, implementing strong identity and access management controls, regularly patching and updating systems, monitoring for suspicious activities, and complying with industry regulations and standards such as GDPR or HIPAA."
What to Look For: Seek candidates who demonstrate a strong understanding of cloud security principles and can discuss best practices for mitigating security risks in cloud environments. Look for examples of security measures and their implications for ensuring data confidentiality, integrity, and availability.
Successfully navigating technical interview questions requires more than just technical knowledge. Here are some tips to help you ace your next technical interview:
Avoiding common pitfalls can help you perform better in technical interviews and increase your chances of success. Here are some common mistakes to watch out for:
As an employer, conducting technical interviews requires careful planning, assessment, and consideration of various factors to ensure you identify the best candidates for your team. Let's delve into each aspect of interviewing from the employer's perspective.
Structuring technical interviews involves defining clear objectives, selecting appropriate interview formats, and creating a consistent and fair evaluation process.
Evaluating candidate responses requires careful observation, active listening, and thoughtful analysis of their technical proficiency, problem-solving skills, and communication abilities. Here's how you can effectively evaluate candidate responses:
Assessing problem-solving skills is a critical aspect of technical interviews, and leveraging pre-employment screening assessments can be an effective strategy. Pre-employment assessments allow employers to evaluate candidates' technical skills and problem-solving abilities in a controlled environment before advancing to the interview stage. These assessments can include coding challenges, algorithmic problems, and system design exercises tailored to the specific requirements of the role. By incorporating pre-employment assessments into the hiring process, employers can streamline candidate evaluation, identify top talent more efficiently, and make more informed hiring decisions.
Gauging cultural fit is essential for ensuring candidates will thrive and contribute positively to your team and organization. Here are some strategies for assessing cultural fit during technical interviews:
By structuring technical interviews effectively, evaluating candidate responses thoughtfully, leveraging pre-employment screening assessments, and gauging cultural fit, employers can identify the best candidates for their technical roles and build high-performing teams that drive innovation and success.
Mastering technical interview questions is a journey that requires dedication, practice, and continuous learning. By understanding the core concepts, practicing problem-solving techniques, and approaching interviews with confidence, both employers and candidates can increase their chances of success. Remember, technical interviews are not just about demonstrating knowledge; they're also an opportunity to showcase problem-solving abilities, communication skills, and cultural fit.
For candidates, preparation is key. Take the time to review core technical concepts, practice coding problems, and familiarize yourself with common interview formats. Additionally, focus on effective communication, problem-solving strategies, and staying calm under pressure. For employers, structuring interviews effectively, evaluating candidates thoughtfully, and assessing cultural fit are essential. By following these principles and strategies, both candidates and employers can navigate technical interviews with confidence and achieve their goals.