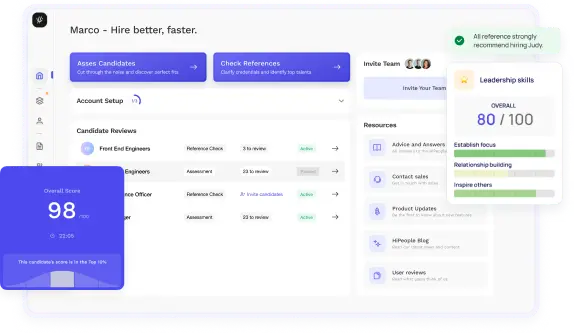
Streamline hiring withour effortless screening.
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Java debugging interviews are a specialized category of job interviews tailored to assess a candidate's debugging skills and problem-solving abilities within the context of Java programming. These interviews are particularly common for positions that involve software development, quality assurance, or system troubleshooting in Java-based projects.
During a Java debugging interview, candidates are presented with coding challenges, real-world scenarios, or problematic code snippets. They are expected to diagnose issues, identify bugs, and propose effective solutions. This assessment allows employers to evaluate how well candidates can identify, analyze, and resolve problems in Java applications.
Java debugging interviews are prevalent in industries where Java is a primary programming language, such as web development, mobile app development, and enterprise software development. To excel in these interviews, candidates need a strong understanding of Java debugging fundamentals, common debugging tools, and effective debugging strategies.
In Java interviews, whether for entry-level or senior positions, debugging skills are highly valued by employers. Debugging is not only an integral part of the software development process but also a key indicator of a candidate's competence in the following areas:
Java debugging interviews typically follow a structured process to evaluate a candidate's skills and suitability for the role. Here is an overview of the interview process:
Before the technical interview, candidates usually undergo an initial screening, which may involve a review of their resume, a phone interview, or an online assessment. During this stage, the recruiter may gauge the candidate's overall qualifications and interest in the position.
The technical interview is the core of the Java debugging interview process. It typically consists of one or more rounds in which candidates are presented with debugging challenges. These challenges can take the form of:
While the primary focus of Java debugging interviews is technical, behavioral interviews are sometimes included to assess soft skills. Candidates may be asked about their teamwork, problem-solving experiences, and their approach to handling challenging situations.
Some companies use coding assessments as part of the interview process. Candidates may be asked to solve coding problems or complete debugging tasks using online coding platforms.
For senior or specialized positions, there may be multiple rounds of technical interviews with increasing complexity. Final rounds may involve discussions with senior engineers or technical leads to assess a candidate's depth of knowledge and expertise.
The interview process varies from one company to another, and the number of rounds and specific evaluation criteria can differ significantly. Candidates should be prepared to demonstrate their Java debugging skills at different levels of difficulty throughout the interview process.
Debugging is a fundamental skill for every Java developer. It's the process of identifying and fixing issues in your code to ensure that your program runs smoothly and correctly. To excel in Java debugging interviews, you need to have a solid grasp of the debugging process.
Debugging typically involves several key steps:
Debugging is not just about fixing code; it's about problem-solving and critical thinking. Interviewers often assess your debugging skills to gauge your ability to tackle real-world challenges effectively. Therefore, understanding the debugging process is a fundamental step in preparing for a Java debugging interview.
To become a proficient Java debugger, you'll need to be familiar with various debugging tools and Integrated Development Environments (IDEs). These tools provide essential features to aid in the debugging process. Here are some commonly used ones:
Each of these tools has its strengths and weaknesses, but they all share core debugging principles. Familiarizing yourself with at least one of these tools and its debugging capabilities will be essential for a successful Java debugging interview.
Before diving into debugging, you need to ensure that your development environment is set up correctly. Here's a step-by-step guide on how to set up a debugging environment for Java:
By following these steps, you'll have a well-configured debugging environment, allowing you to effectively identify and resolve issues during your Java debugging interviews. Familiarity with the setup process demonstrates your preparedness and professionalism to potential employers.
How to Answer: When faced with a NullPointerException, start by examining the stack trace to pinpoint the exact line of code where the exception occurred. Then, inspect the variables and objects involved to identify which one is null. Finally, use proper null-checking techniques, such as conditional statements or optional types, to handle the null value and prevent the exception from occurring again.
Sample Answer: In the event of a NullPointerException, I would carefully analyze the stack trace to find the line causing the issue. Once identified, I would check the variables and objects involved to determine which one is null. For example, if objA
is null, I would add a conditional check like if (objA != null)
before attempting any method calls on objA
. This prevents the NullPointerException from happening again.
What to Look For: Look for candidates who demonstrate a systematic approach to diagnosing and addressing NullPointerExceptions. Ensure they understand the importance of null-checking and can provide clear examples of how to implement it in code.
How to Answer: A Java debugger is a tool used for inspecting, analyzing, and debugging code during runtime. To use it, you set breakpoints at specific lines of code, run the program in debug mode, and then step through the code one line at a time. You can inspect variable values, evaluate expressions, and identify the root cause of issues.
Sample Answer: A Java debugger is essential for identifying and fixing bugs in code. To use it effectively, I would first set breakpoints at critical points in the code where I suspect issues might occur. Then, I'd run the program in debug mode. As the program runs, I'd step through the code line by line, examining variable values and evaluating expressions to pinpoint the problem. This allows me to understand the code's flow and identify the root cause of any issues.
What to Look For: Look for candidates who are familiar with debugging tools and can articulate their debugging process. Ensure they understand how to set breakpoints, step through code, and use debugging features effectively.
How to Answer: A stack trace is a textual representation of the call stack, showing the sequence of method calls leading to an exception or error. It helps in identifying issues by providing a clear path of execution and highlighting the exact location where an exception occurred. Analyzing the stack trace allows developers to trace back through the code and identify the source of the problem.
Sample Answer: A stack trace is a crucial tool in Java debugging. It displays a list of method calls, starting from the point of the exception and going back to the initial method invocation. By examining the stack trace, I can trace the sequence of method calls that led to the issue, helping me pinpoint where the problem originated. This makes it easier to understand the code's execution flow and identify the root cause of errors.
What to Look For: Look for candidates who can explain the purpose of a stack trace and its importance in debugging. They should be able to demonstrate their ability to interpret stack traces and use them effectively to diagnose issues.
How to Answer: Debugging a memory leak involves using memory profiling tools to identify objects that are not being properly garbage collected. Candidates should describe how they would use tools like VisualVM or YourKit to analyze memory usage, identify the objects causing the leak, and then refactor code to release unused resources.
Sample Answer: To debug a memory leak, I would start by using memory profiling tools like VisualVM or YourKit to monitor the application's memory usage. These tools can help identify objects that are not being properly garbage collected. Once I identify the objects causing the leak, I would examine the code responsible for creating and retaining those objects. I'd refactor the code to release unused resources and ensure proper garbage collection, thus resolving the memory leak.
What to Look For: Look for candidates who are proficient in memory profiling tools and can outline a systematic approach to debugging memory leaks. They should be able to demonstrate their ability to identify and resolve memory-related issues.
How to Answer: To analyze and debug performance issues, candidates should mention profiling tools like Java Flight Recorder and VisualVM. They should explain how to capture performance data, analyze CPU and memory usage, and optimize code and resource usage for better performance.
Sample Answer: To analyze and debug performance issues in a Java application, I would use profiling tools like Java Flight Recorder or VisualVM. These tools allow me to capture performance data, including CPU and memory usage, thread activity, and method execution times. By analyzing this data, I can pinpoint bottlenecks and areas of inefficiency in the code. I would then work on optimizing these areas, such as reducing memory allocation, improving algorithms, or optimizing database queries, to enhance the application's performance.
What to Look For: Look for candidates who are skilled in using profiling tools and can explain their process for identifying and addressing performance bottlenecks in Java applications.
How to Answer: Handling concurrent programming issues involves understanding concepts like synchronization, locks, and thread safety. Candidates should discuss strategies for identifying race conditions, deadlocks, and thread-related problems, and how to use tools like thread dumps and profilers to debug these issues.
Sample Answer: Debugging concurrent programming issues in Java requires a strong understanding of thread safety and synchronization. I would start by reviewing the code for proper synchronization mechanisms, such as synchronized blocks or locks, to prevent race conditions. If I encounter a deadlock, I would analyze thread dumps to identify the locked threads and the resources they are waiting for. Profiling tools can also help pinpoint performance bottlenecks caused by concurrent access. By carefully analyzing and addressing these issues, I can ensure the application functions correctly in a multi-threaded environment.
What to Look For: Look for candidates with expertise in concurrent programming and the ability to explain how they handle and debug issues related to thread safety and synchronization. They should demonstrate proficiency in using thread dumps and profiling tools to diagnose problems.
How to Answer: Candidates should discuss practices such as writing clear and modular code, using meaningful variable names, documenting code, and incorporating unit tests. They should emphasize the importance of regular debugging sessions and collaboration with colleagues to catch issues early.
Sample Answer: Efficient Java debugging starts with writing clean, modular code. Using meaningful variable names and documenting code helps make debugging easier. Regular debugging sessions are essential, and I find it beneficial to collaborate with team members during code reviews to identify potential issues. Additionally, incorporating unit tests helps catch problems early in the development process, reducing the need for extensive debugging later on.
What to Look For: Look for candidates who advocate for best practices that promote clean code and early issue detection. They should prioritize collaboration and testing as integral parts of the debugging process.
How to Answer: Candidates should discuss strategies like profiling, benchmarking, and load testing. They should explain how to identify and optimize resource-intensive code sections, database queries, and external dependencies to improve application performance.
Sample Answer: To efficiently debug performance bottlenecks in a Java application, I would start by using profiling tools to identify resource-intensive code sections, slow database queries, or external dependencies causing delays. Then, I'd perform benchmarking and load testing to simulate real-world scenarios and measure performance improvements. I'd focus on optimizing these identified areas, such as optimizing database indexes, reducing unnecessary CPU usage, and implementing caching mechanisms to enhance overall application performance.
What to Look For: Look for candidates who demonstrate a structured approach to debugging performance issues. They should be knowledgeable about profiling tools and performance optimization techniques.
How to Answer: Candidates should explain that "ClassCastException" occurs when casting objects to incompatible types and should discuss using instanceof checks, reviewing the code for type mismatches, and considering design changes to avoid such exceptions.
Sample Answer: Handling and debugging a "ClassCastException" in Java involves first using instanceof checks to ensure that casting is safe. I would review the code where the exception occurs, examining the types involved to identify any type mismatches. If needed, I might consider design changes, such as using polymorphism or reevaluating the class hierarchy, to prevent such exceptions in the future.
What to Look For: Look for candidates who can effectively handle and debug "ClassCastException" by applying safe casting practices and considering design improvements.
How to Answer: Candidates should describe the scenarios where "ConcurrentModificationException" occurs, discuss using iterators and synchronized collections, and consider alternative data structures or approaches to avoid this exception.
Sample Answer: "ConcurrentModificationException" typically occurs when modifying a collection while iterating over it. To handle this exception, I would use iterators, as they allow safe modification of the collection during iteration. If synchronization is required, I would consider using synchronized collections or employing explicit synchronization mechanisms. In some cases, it might be beneficial to explore alternative data structures or approaches, such as using concurrent collections, to avoid "ConcurrentModificationException."
What to Look For: Look for candidates who understand the causes of "ConcurrentModificationException" and can provide solutions to handle and prevent it effectively.
How to Answer: Candidates should mention tools like Eclipse Debugger, IntelliJ IDEA Debugger, and command-line debugging tools. They should explain how these tools assist in setting breakpoints, inspecting variables, and tracking program flow during debugging sessions.
Sample Answer: Commonly used debugging tools in Java include Eclipse Debugger and IntelliJ IDEA Debugger, both of which provide user-friendly debugging environments. These tools allow developers to set breakpoints in the code, inspect variable values, and step through code execution. Additionally, command-line debugging tools like jdb offer similar functionality for debugging Java applications from the command line. These tools greatly assist in identifying and resolving issues during development.
What to Look For: Look for candidates who are familiar with popular Java debugging tools and can explain their features and benefits for effective debugging.
How to Answer: Candidates should discuss the use of logging frameworks like Log4j or SLF4J to capture relevant information during runtime. They should explain how to configure logging levels, log messages, and utilize log analysis tools to identify issues.
Sample Answer: Java logging frameworks like Log4j or SLF4J are valuable for debugging and troubleshooting. I would configure logging levels to capture relevant information during runtime, ensuring that it's neither too verbose nor too minimal. By strategically placing log messages in the code, I can trace the program's flow and values of variables. Additionally, log analysis tools can help identify patterns and potential issues by analyzing log files, making troubleshooting more efficient.
What to Look For: Look for candidates who understand how to effectively use logging frameworks to aid in debugging and troubleshooting Java applications.
How to Answer: Candidates should emphasize the importance of communication and collaboration within the team. They should discuss strategies like code reviews, pair programming, and using version control systems effectively to catch and resolve issues early.
Sample Answer: When working on a codebase as part of a development team, collaboration is key to effective debugging. We regularly conduct code reviews, which help catch issues early and ensure code quality. Pair programming is another strategy we employ, allowing two developers to work together and provide immediate feedback. Utilizing version control systems helps us track changes and revert to previous states if needed. Overall, effective communication and teamwork contribute to more efficient debugging processes.
What to Look For: Look for candidates who emphasize collaboration and teamwork as essential components of successful debugging in a team environment.
How to Answer: Candidates should describe a diplomatic approach to addressing the problem. They should discuss open communication with the colleague, explaining the issues, offering assistance, and working together to find a resolution. The focus should be on teamwork and achieving a common goal.
Sample Answer: If a colleague's code causes issues in a shared project, I believe in open communication and teamwork. I would reach out to the colleague, explaining the specific issues that have arisen due to their code. Instead of assigning blame, I'd offer my assistance in resolving the problem together. By collaborating and focusing on the shared goal of project success, we can work towards a solution that benefits everyone involved.
What to Look For: Look for candidates who prioritize effective communication and collaboration when addressing code-related issues caused by colleagues.
How to Answer: Candidates should outline a systematic approach that includes understanding the problem, reproducing the issue, gathering relevant information, isolating the root cause, implementing a solution, and testing thoroughly. They should emphasize the importance of documentation and continuous improvement in their methodology.
Sample Answer: My methodology for approaching complex debugging tasks in Java begins with a deep understanding of the problem. I make sure to reproduce the issue and gather as much relevant information as possible, including stack traces and log files. Next, I isolate the root cause through systematic investigation, using debugging tools and techniques. Once identified, I implement a solution and thoroughly test it, making sure it doesn't introduce new issues. Throughout the process, documentation is crucial for tracking the steps taken and sharing knowledge with the team. Continuous improvement is also a key aspect of my methodology, as I strive to learn from each debugging experience to become a more effective developer.
What to Look For: Look for candidates who demonstrate a structured and comprehensive methodology for tackling complex debugging tasks in Java. They should emphasize thorough understanding, documentation, and a commitment to continuous improvement.
In the realm of Java debugging, several crucial concepts are essential for mastering the art of identifying and resolving issues in your code. These concepts provide the foundation for success in Java debugging interviews and real-world development scenarios.
Breakpoints are invaluable tools for Java developers when it comes to debugging. They are markers you place in your code, indicating specific locations where you want your program's execution to pause. Breakpoints allow you to inspect the state of your program, examine variables, and understand how your code flows. Here's a deeper look at breakpoints and their usage:
Effective use of breakpoints in your Java debugging interviews demonstrates your ability to pinpoint problems, analyze code, and control the flow of your program to find solutions efficiently.
Stepping through code is a fundamental debugging technique that allows you to observe the execution flow of your program in detail. This concept involves several actions you can take while debugging to gain insights into your code's behavior:
Mastering these stepping actions is essential, especially when navigating complex codebases. During your Java debugging interview, you may be asked to demonstrate your ability to step through code effectively to identify issues and understand program behavior.
Understanding how to inspect variables and data during debugging is crucial for identifying the root causes of issues. Debuggers provide tools and features to help you examine the values of variables and data structures:
Being proficient in inspecting variables and data not only helps you find issues more quickly but also allows you to gain insights into how your code operates. This skill is highly valuable in both interviews and practical development scenarios.
Exception handling is a critical aspect of Java development, and debugging exceptions efficiently is essential for delivering robust and reliable code. Here are key points to understand:
Effective exception handling and debugging are essential skills for a Java developer. In interviews, you may be asked about your approach to dealing with exceptions and your ability to troubleshoot code when exceptions occur.
Multithreaded Java applications introduce complexities that require specialized debugging techniques. Debugging in a multithreaded environment involves:
In Java debugging interviews, you may be presented with multithreaded code scenarios and asked to diagnose and resolve synchronization or deadlock issues. Proficiency in multithreading debugging sets you apart as a skilled Java developer.
In Java debugging interviews, you'll often encounter questions and scenarios that test your problem-solving skills and your ability to debug real-world code. Let's explore these common interview topics in detail:
Debugging Techniques and Strategies are crucial for effectively identifying and fixing issues in your Java code. It's not just about finding bugs; it's about doing so efficiently and systematically. Here are some key debugging techniques and strategies to keep in mind:
During your Java debugging interview, you might be asked about your preferred debugging techniques and how you approach challenging debugging scenarios. Demonstrating a systematic and creative approach to debugging can set you apart.
Debugging Code Examples are a common way for interviewers to assess your practical debugging skills. You may be given a piece of Java code with issues, and your task is to identify and fix them. Here's what to keep in mind:
During the interview, the code examples provided may cover various topics such as logic errors, exceptions, multithreading issues, or data structure problems. Your ability to systematically approach debugging and communicate your thought process is essential.
Runtime exceptions can be challenging to deal with, but knowing how to Handle Runtime Exceptions effectively is crucial for maintaining the stability of your Java applications. During debugging interviews, you might be asked about your approach to handling exceptions. Here are some key points to consider:
Handling runtime exceptions is a fundamental aspect of Java development, and your ability to do so effectively will be evaluated in interviews.
Multithreading introduces complexities and challenges in debugging. Interviewers may assess your ability to Debug Multithreaded Applications, as it's a common requirement in many Java projects. Here's what you should know:
Multithreading debugging can be particularly challenging, as issues may be intermittent and hard to reproduce. Demonstrating your ability to handle these complexities during interviews is essential.
Debugging Best Practices encompass a set of guidelines and strategies to help you become a more effective debugger. Employing these practices not only makes debugging more efficient but also results in cleaner and more maintainable code.
By following these debugging best practices, you can minimize the likelihood of encountering bugs and streamline your debugging process. During interviews, demonstrating your commitment to best practices showcases your professionalism as a developer.
Preparing for a Java debugging interview goes beyond just knowing how to debug code effectively. It involves demonstrating your expertise, problem-solving abilities, and your ability to communicate your thought process clearly. Here are some essential steps to prepare for your Java debugging interview:
A Debugging Portfolio is a collection of your debugging accomplishments, showcasing your skills and expertise to potential employers. This portfolio can set you apart from other candidates and provide concrete evidence of your abilities. Here's how to build an impressive debugging portfolio:
Having a well-organized debugging portfolio allows you to present tangible evidence of your abilities during the interview. It's a powerful tool for demonstrating your expertise and problem-solving skills.
Practice is essential for success in a Java debugging interview. By Practicing Interview Questions and Scenarios, you can sharpen your skills and gain confidence. Here's how to approach practice effectively:
By practicing a wide range of debugging scenarios and questions, you'll be better prepared to handle whatever challenges your interview presents. Practicing also helps you refine your problem-solving strategies and improve your efficiency.
Effective communication is a vital skill during a Java debugging interview. Your ability to Effectively Communicate your thought process, reasoning, and findings can greatly influence the interviewer's impression of your skills. Here are some tips to enhance your communication:
Effective communication is not only about finding solutions but also about conveying your thought process clearly. Practicing communication skills alongside technical skills is essential for interview success.
In summary, preparing for a Java debugging interview involves building a portfolio that showcases your skills, practicing a variety of scenarios, and honing your communication abilities. By following these steps, you'll be well-equipped to excel in your interview and demonstrate your expertise in debugging Java applications.