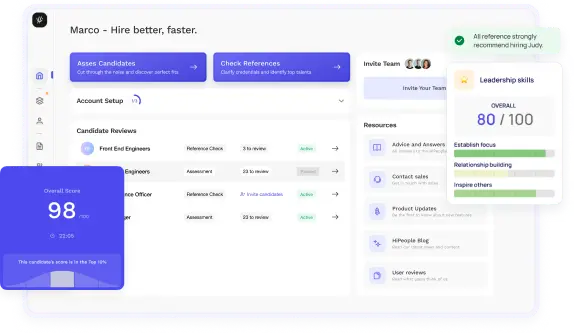
Streamline hiring withour effortless screening.
Optimise your hiring process with HiPeople's AI assessments and reference checks.
Are you ready to master Express.js interviews and stand out as a top candidate or employer in the ever-evolving tech landscape? Delve into our guide on Express JS interview questions, designed to equip you with the knowledge and insights needed to excel in discussions related to Express and web development. Whether you're seeking to demonstrate your expertise or evaluate potential hires, this guide is your go-to resource for navigating the intricate world of Express.js interviews with confidence and competence.
Express.js interviews are a crucial part of the hiring process for web developers, especially those who specialize in building web applications using the Express.js framework. These interviews are designed to assess a candidate's knowledge, skills, and experience related to Express.js and its ecosystem. Whether you're an aspiring candidate or an employer looking to evaluate potential hires, understanding the dynamics of Express JS interviews is essential to succeed in the competitive tech industry.
ExpressJS, being a widely adopted Node.js web application framework, holds significant importance in the world of web development interviews. Here's why it's a focal point:
Understanding the significance of Express.js in interviews helps both candidates and employers focus their efforts on the areas that matter most, leading to more successful interviews and hiring decisions.
Express.js is the go-to framework for many web developers when it comes to building web applications with Node.js. We'll delve deeper into the fundamentals of Express JS, starting with an overview and then exploring its key features, setting up an Express application, and the critical concept of middleware.
Express.js, or ExpressJS, often referred to simply as Express, is a web application framework for Node.js. It was created to simplify the process of building robust and efficient web applications by providing a minimal, unopinionated framework with essential features. Express is known for its speed and flexibility, making it a popular choice in the Node.js ecosystem.
Express is designed to handle routing, HTTP requests and responses, middleware management, and more. It doesn't impose strict conventions on how you should structure your application, giving you the freedom to build your web server the way you want.
ExpressJS comes with a range of features and advantages that contribute to its popularity among developers:
Before you start building web applications with Express, you'll need to set up your development environment and create a basic Express application. Here's an overview of the steps involved:
package.json
file:
npm init -y
This command uses the -y
flag to accept all default options for simplicity.
npm install express --save
The --save
flag adds Express as a dependency in your package.json
file.app.js
or index.js
). Here's a basic example to get you started:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Start the Application: Finally, run your Express application with the following command:
node app.js
Your Express server should now be running locally on port 3000.Middleware is a fundamental concept in Express JS. It allows you to execute functions or logic at specific points during the request-response cycle. Express uses middleware for various purposes, including processing requests, handling authentication, logging, and error management.
Middleware functions have access to the request
and response
objects and can modify them or terminate the request cycle by sending a response. You can use built-in middleware, third-party middleware packages, or create custom middleware to suit your application's needs.
To better understand middleware, let's explore some common use cases and examples in the context of Express JS.
How to Answer: Begin by explaining that Express.js is a web application framework for Node.js. Highlight its popularity due to its minimalistic design, robust routing capabilities, middleware support, and ease of building RESTful APIs. Mention that it simplifies the process of building web applications in Node.js.
Sample Answer: "Express.js is a web application framework for Node.js, known for its simplicity and flexibility. It's popular in web development because it provides a minimalist structure to build web applications, offers robust routing capabilities, supports middleware for extending functionality, and makes creating RESTful APIs a breeze. It streamlines the development process and enhances productivity."
What to Look For: Look for candidates who can articulate the key features and advantages of Express.js and its relevance in modern web development. Assess their understanding of how Express.js simplifies application development.
How to Answer: Define middleware as functions that process incoming HTTP requests or modify responses before they reach the route handler. Explain its importance in Express.js, as it allows for modular and extensible application design.
Sample Answer: "Middleware in Express.js refers to functions that handle request processing or response modification before they reach the route handler. It's essential because it enables modular and extensible application design. Middleware functions can perform tasks like authentication, logging, and data validation, enhancing the application's functionality and maintainability."
What to Look For: Evaluate candidates' understanding of middleware in Express.js and their ability to provide clear examples of its use in web applications. Look for familiarity with common middleware libraries and their benefits.
How to Answer: Describe that app.get()
is used to define a route for handling GET requests, while app.use()
is used to mount middleware functions that are executed for all HTTP methods. Emphasize the distinction between routing and middleware.
Sample Answer: "In Express.js, app.get()
is used to define a route specifically for handling GET requests. On the other hand, app.use()
is used to mount middleware functions that are executed for all HTTP methods, regardless of the specific route. app.get()
is primarily for routing, while app.use()
is for middleware that should be executed globally."
What to Look For: Assess candidates' comprehension of route handling and middleware application in Express.js. Check if they can differentiate between the two and when to use each method.
How to Answer: Explain that routing parameters are defined in route paths using colons (:
) followed by parameter names. Access these parameters in the route handler using req.params
. Provide an example of parameterized routing.
Sample Answer: "In Express.js, you can handle routing parameters by defining them in route paths using colons, like :paramName
. To access these parameters in the route handler, you use req.params.paramName
. For instance, if the route is '/users/:id', you can access the 'id' parameter using req.params.id
in the handler."
What to Look For: Look for candidates who demonstrate a clear understanding of how to define and utilize routing parameters in Express.js. Check for their ability to provide practical examples.
How to Answer: Define route chaining as the practice of attaching multiple route handlers to a single route path. Explain that it is accomplished by invoking app.route()
and chaining HTTP method calls. Mention its benefits in code organization.
Sample Answer: "Route chaining in Express.js involves attaching multiple route handlers to a single route path. It's implemented by using app.route()
to specify the path and then chaining HTTP method calls like get()
, post()
, or put()
. This approach improves code organization by keeping related route handlers together."
What to Look For: Assess candidates' familiarity with route chaining in Express.js and their ability to explain its purpose and implementation. Look for clarity in their responses.
How to Answer: Describe the use of middleware for error handling in Express.js. Explain that error-handling middleware typically has four parameters (err
, req
, res
, and next
) and should be defined after all other route handlers. Emphasize the importance of calling next(err)
to propagate errors.
Sample Answer: "In Express.js, error handling is implemented using middleware specifically designed for error handling. Error-handling middleware typically has four parameters: err
, req
, res
, and next
. It should be defined after all other route handlers. To propagate errors, you should call next(err)
within the error-handling middleware. This allows you to centralize error handling and respond to errors appropriately."
What to Look For: Evaluate candidates' understanding of error handling in Express.js, including the use of error-handling middleware and the importance of propagating errors using next(err)
. Look for examples of how they've handled errors in previous projects.
How to Answer: Differentiate between synchronous error handling using throw
and asynchronous error handling using next(err)
. Explain that synchronous errors are immediate, while asynchronous errors require passing the error to the next middleware.
Sample Answer: "Synchronous error handling in Express.js involves using throw
to immediately trigger an error response. On the other hand, asynchronous error handling is achieved by calling next(err)
, allowing you to pass the error to the next middleware in the stack. Synchronous errors are immediate, whereas asynchronous errors continue through the middleware chain until encountered by error-handling middleware."
What to Look For: Assess candidates' grasp of the distinction between synchronous and asynchronous error handling in Express.js. Look for examples of when each approach is appropriate.
How to Answer: Explain that authentication middleware is used to verify user identity before granting access to protected routes. Describe the process, including checking credentials, setting user information in req
, and invoking next()
if authentication succeeds.
Sample Answer: "To implement authentication middleware in Express.js, you typically check user credentials, such as a username and password. If authentication succeeds, you set user information in the req
object and call next()
to pass control to the next middleware or route handler. If authentication fails, you can respond with an error message or redirect as needed."
What to Look For: Look for candidates who can outline the steps involved in implementing authentication middleware and who demonstrate an understanding of user authentication concepts.
How to Answer: Explain that JWT (JSON Web Tokens) authentication is a stateless authentication mechanism using tokens. Describe the process of generating and verifying JWTs in Express.js, emphasizing the use of libraries like jsonwebtoken
.
Sample Answer: "JWT authentication in Express.js is a stateless authentication method that uses tokens for user identification. To implement it, you generate a JWT token when a user logs in and verify it for subsequent requests. You can use libraries like jsonwebtoken
to handle token generation and verification, making it a secure and scalable choice for authentication."
What to Look For: Assess candidates' knowledge of JWT authentication and their ability to explain its implementation in Express.js. Look for experience with JWT libraries and best practices for secure token handling.
How to Answer: Explain that designing a RESTful API in Express.js involves mapping HTTP methods (GET, POST, PUT, DELETE) to CRUD operations (Create, Read, Update, Delete) on resources. Describe the use of route paths and HTTP methods to create a RESTful API structure.
Sample Answer: "Designing a RESTful API in Express.js means mapping HTTP methods to CRUD operations on resources. You use route paths and HTTP methods like GET, POST, PUT, and DELETE to define endpoints for creating, reading, updating, and deleting resources. Properly structured route paths and consistent naming conventions are essential for a well-designed RESTful API."
What to Look For: Evaluate candidates' understanding of RESTful API design principles and their ability to explain how Express.js facilitates the creation of RESTful APIs. Look for experience in structuring RESTful routes.
How to Answer: Describe the use of query parameters (e.g., page
and limit
) to implement pagination in a RESTful API. Explain that pagination allows clients to retrieve subsets of data from large collections.
Sample Answer: "To handle pagination in a RESTful API using Express.js, you can use query parameters like page
and limit
. Clients can request a specific page and specify the number of items per page using these parameters. Implementing pagination helps manage large data collections efficiently and provides a better user experience."
What to Look For: Assess candidates' knowledge of pagination techniques in RESTful APIs and their ability to explain how query parameters are used for this purpose. Look for practical examples or experience in implementing pagination.
How to Answer: List common security vulnerabilities (e.g., SQL injection, XSS, CSRF) and describe mitigation strategies, such as input validation, using secure authentication mechanisms, and setting appropriate HTTP headers.
Sample Answer: "To mitigate common security vulnerabilities in Express.js applications, you should implement input validation to prevent SQL injection and XSS attacks. Use secure authentication mechanisms like JWT and OAuth for user authentication. Additionally, set appropriate HTTP headers to protect against CSRF attacks and other security threats."
What to Look For: Look for candidates who can identify security vulnerabilities and explain how to address them in Express.js applications. Assess their familiarity with security best practices.
How to Answer: Define CORS (Cross-Origin Resource Sharing) as a security feature that controls access to resources from different origins. Explain how to enable CORS in Express.js by using the cors
middleware or configuring headers to allow specific origins.
Sample Answer: "CORS, or Cross-Origin Resource Sharing, is a security feature that controls which origins can access resources on a server. In Express.js, you can enable CORS by using the cors
middleware, which simplifies configuration. Alternatively, you can manually configure response headers to allow specific origins, methods, and headers for cross-origin requests."
What to Look For: Assess candidates' understanding of CORS and their ability to explain how to enable it in Express.js applications. Look for knowledge of both the cors
middleware and manual configuration.
How to Answer: Explain performance optimization techniques, including using caching mechanisms, compressing responses, minimizing blocking code, and employing load balancing. Emphasize the importance of profiling and benchmarking.
Sample Answer: "To optimize the performance of an Express.js application, you can implement various strategies. Use caching mechanisms like Redis to store frequently accessed data, compress responses to reduce bandwidth usage, minimize blocking code by leveraging asynchronous operations, and consider load balancing for distributing traffic. Profiling and benchmarking are crucial to identify bottlenecks and areas for improvement."
What to Look For: Look for candidates who can discuss performance optimization techniques and their understanding of how to enhance the efficiency of Express.js applications. Check for experience with tools and practices for performance monitoring.
How to Answer: Explain how Express.js utilizes Node.js's event loop to handle concurrency by processing multiple requests concurrently. Mention that Express.js is single-threaded but non-blocking, allowing it to efficiently manage high levels of concurrency.
Sample Answer: "Express.js leverages Node.js's event loop to handle concurrency efficiently. While Express.js runs in a single thread, it's non-blocking, meaning it can handle multiple requests concurrently. This is achieved by delegating I/O operations to the event loop, allowing Express.js to efficiently manage high levels of concurrency without creating new threads."
What to Look For: Assess candidates' understanding of how Express.js handles concurrency and its relationship with the Node.js event loop. Look for insights into how this architecture benefits application performance.
When it comes to Express JS interviews, there are several key topics that you should be well-prepared for. These topics go beyond the basics and are often the focus of technical discussions and assessment. Let's explore each of these topics in detail:
Routing is a fundamental concept in web development, and Express provides a powerful mechanism for defining and handling routes in your application. In Express, routes are used to determine how your application responds to client requests. You'll be expected to understand:
Understanding the request
and response
objects is crucial for building robust Express applications. These objects represent the incoming HTTP request and the outgoing HTTP response, respectively. In your interview, you may be asked to:
request
and response
objects.response
object to send various types of responses (e.g., JSON, HTML, files).request
and response
objects.Error handling is a critical aspect of web development, and Express provides mechanisms for effectively managing errors in your application. You should be prepared to discuss:
In many Express JS interviews, you may encounter questions related to designing RESTful APIs. REST (Representational State Transfer) is a widely used architectural style for designing networked applications. Be ready to discuss:
Security is a top priority in web development, and you should be familiar with implementing authentication and authorization mechanisms in Express. Expect questions on:
Asynchronous programming is a core concept in Node.js, and Express JS interviews often include questions related to handling asynchronous operations. You should be comfortable discussing:
Testing is a crucial part of software development, and your knowledge of testing Express applications will be assessed in interviews. Be prepared to discuss:
These common interview topics cover a wide range of Express JS concepts and skills. By thoroughly understanding and practicing these areas, you'll be well-equipped to excel in Express JS interviews and demonstrate your expertise in building web applications with Express.
In the world of Express JS interviews, showcasing expertise in advanced topics can set you apart as a seasoned developer. These topics require a deeper understanding of Express and its ecosystem. Let's delve into each of these advanced areas:
Security is paramount in web development, and it's a topic of great importance in Express JS interviews. You'll be expected to demonstrate a strong grasp of security best practices, including:
Optimizing the performance of your Express applications is crucial for delivering a smooth user experience. During interviews, you may be asked about strategies and techniques for optimizing Express applications, including:
Real-time applications are increasingly common, and understanding how to implement them with WebSockets in Express is a valuable skill. Be prepared to discuss:
Creating custom middleware is a powerful way to extend the functionality of your Express applications. In interviews, you may be asked to:
Database integration is a fundamental aspect of web development, and Express JS interviews often explore how you interact with databases. Be prepared to discuss:
Mastering these advanced topics will not only impress interviewers but also equip you with the skills necessary to build high-performance, secure, and real-time Express applications. Keep honing your knowledge and practice to excel in Express JS interviews.
Preparing for an Express JS interview requires more than just technical knowledge; it's about showcasing your skills and potential as a valuable team member. Here are some essential tips to help you ace your Express JS interview:
Conducting an Express JS interview that accurately evaluates candidates can be a challenging task. Here are some tips for employers to ensure a successful interview process:
By following these tips, employers can conduct Express JS interviews that identify candidates who not only possess the technical skills but also align with the company's culture and goals.
Mastering Express JS interview questions is the key to unlocking exciting opportunities in the world of web development. Armed with a solid understanding of Express fundamentals, advanced topics, and valuable tips, you're well-prepared to tackle technical discussions, showcase your skills, and land your dream job.
For employers, this guide provides valuable insights into evaluating Express JS candidates effectively, ensuring that you identify individuals who can contribute to your team's success. Express JS continues to play a pivotal role in modern web development, making it essential for both candidates and employers to stay ahead of the curve. Keep learning, practicing, and adapting, and you'll thrive in the dynamic world of Express JS.